call(), apply(), bind() Methods in JavaScript with Examples
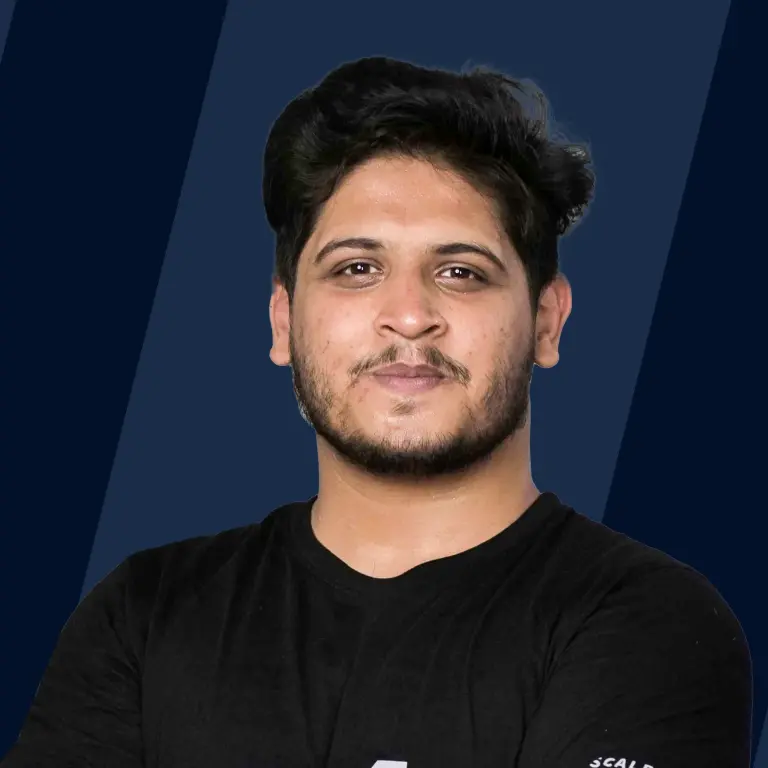
Understanding the call(), apply(), and bind() methods in JavaScript is crucial for any developer looking to master function manipulation and execution contexts. These methods are foundational to writing maintainable and extensible code, enabling greater code flexibility and reusability.
Bind() Method in JavaScript
The bind() method in JavaScript is a function that allows developers to explicitly set the context in which a function is executed. It creates a new function, referred to as a bound function, where the specified object becomes the this value within that function.
Syntax
- originalFunction: The function to be bound.
- thisArg: The object to which the this keyword should refer within the bound function.
- arg1, arg2, ...: Optional parameters that can be preset in the new function.
The bind() method creates a new function with the specified thisArg and optional preset arguments. When boundFunction is later invoked, the this value inside the function will be the provided by thisArg, and any preset arguments will be appended to the arguments passed during invocation. The bind() function returns a new function with the bound context.
Examples
In this code, a function greet is defined, taking a name parameter. Inside the function, a greeting message is logged to the console, incorporating both the provided name and a property framework accessed through the this keyword.
Output
The first argument passed to bind() is an object { framework: 'JavaScript' }, setting the context (this) for the newly created function. The second argument, 'Developer', is a preset value for the name parameter in the original greet function.
Solution for this Problem Using bind()
To resolve the this binding issue, you can use bind() to explicitly set the context when calling the callback function.
- In the customFilter method, during each iteration, the callback is invoked with the correct context using callback.bind(this).
- The callback function is then immediately invoked with the current element, index, and the array as arguments.
- The condition in the callback checks whether the current number is even and if true, it is added to the result array.
Output:
By using bind() in this context, we ensure that the this keyword inside the callback refers correctly to the array being filtered, addressing the potential this problem in polyfills and custom methods.
In regular functions, the value of this is dynamically determined at runtime based on how the function is invoked. Arrow functions, on the other hand, inherit the this value from the surrounding enclosing scope. They do not have their own this context and cannot be used with apply() function, making the application of arguments impractical. Similarly it is irrelevant to use arrow functions with call() or bind() functions too.
Call() Method in JavaScript
Moving on to the call() method, it is a function prototype method that allows the immediate execution of a function with a specified context and a set of arguments. The call() method enables developers to explicitly set the context for a particular function call.
Syntax
The call() method immediately executes the specified function (functionName) with the provided thisArg as the context. Any additional arguments (arg1, arg2, ...) are passed to the function during the call.
Example
- The greet function is defined to log a greeting message, incorporating the name property of the object referenced by this.
- The call() method is applied to the greet function with different objects as arguments.
Output:
When greet.call(person1) is executed, the this within the greet function refers to person1. Therefore, the message logged is "Hello, Alice!" Similarly, the next method sets this to person2, resulting in the message "Hello, Bob!" being logged.
For an in-depth exploration of the call() method, refer to this comprehensive guide.
Apply() Method in JavaScript
The apply() method in JavaScript is a function prototype method that executes the function with a specified context and an array or array-like object as its arguments. The apply() method explicitly sets the context for a function call, but differs by accepting an array of arguments. This is useful when the number of arguments or their values may vary dynamically.
Syntax
The [arg1, arg2, ...] is an array or array-like object containing the arguments to be passed to the function during the call.
The apply() method executes the specified function (functionName) with the provided thisArg as the context (the value of this inside the function). The array of arguments is then passed to the function during the call.
Example
- The sum function is used to dynamically calculate the sum of the elements within the numbers array.
- The apply() method is applied to the sum function with numbers as the context. The array numbers are passed as the argument, effectively becoming the array on which the reduce operation is performed.
- The reduce operation is used to iterate through the array and accumulate the values.
Output:
Since the number is passed to the apply function, the this within the sum function refers to the numbers array during execution. The reduce method is then applied to sum up the elements of the numbers array, starting from an initial accumulator value of 0. The result is logged to the console, producing the sum of the elements as the output.
Explore more about the apply() method and how this can be used to resolve the this problem of polyfils in here.
Polyfills in JavaScript
Polyfills in JavaScript serve as crucial mechanisms for enabling the use of modern language features in older environments. As new features are introduced in the language, older browsers may not inherently support them, potentially causing compatibility issues. Polyfills provide the necessary functionality for modern language features in environments where they are not natively supported.
Example
Let's consider the String.startsWith() method, which checks if a string starts with a specified sequence of characters. Before widespread browser support, developers often created polyfills for this functionality.
Explanation:
- The code checks whether the startsWith method is already defined for the String prototype. The String.prototype refers to the set of methods and properties available for all string instances in JavaScript.
- If the startsWith method is not present, a polyfill is created by adding a custom implementation of the startsWith method.
- The polyfill utilizes the indexOf method to check if the string starts with the specified substring, returning true or false accordingly.
Implementation
If the code is executed in a modern browser, the startsWith method will be invoked or, the declared polyfill will be invoked.
Output:
In this output, the polyfill startsWith() method successfully checks whether the string greeting starts with the specified substrings, providing the expected results.
Problem of this Keyword in Polyfills
In JavaScript, the this keyword refers to the current execution context within a function. However, in polyfills, the this keyword might not refer to the intended instance of the object, which can lead to unexpected behavior, errors, or unintended side effects.
Example:
Explanation
- The custom filter method is designed to iterate through each element of the array, applying a user-provided callback function (callback).
- The customFilter method creates an empty array called result to store elements that pass the filtering criteria. For each element in the original array (this), the callback is invoked with the current element, its index, and the original array as arguments. If the callback returns true, the element is added to the result array.
- However, there is an issue with the use of the this keyword inside the callback. The this inside the callback may not refer to the array being operated on, leading to unintended behavior.
Implementation:
In this output, due to the incorrect binding of this, the filtering logic produces unexpected results.
The this binding issue can be resolved by using the methods call, apply, bind in javascript.
FAQs
Q: Why use bind() instead of call() or apply()?
A: While call() and apply() immediately execute the function, bind() creates a new function with a specified context, allowing deferred execution.
Q: Can I use apply() with arrow functions?
A: No, arrow functions do not have their own this context, making the use of apply() impractical.
Q: How does bind() handle function parameters, and why is it beneficial in certain scenarios?
A: The bind() allows developers to preset parameters in the newly created function. This feature is advantageous when there is a need to partially apply arguments, creating more flexible and reusable functions.
Conclusion
- Call, apply, bind in javascript are indispensable methods, providing developers with the means to dynamically manipulate function behavior and ensure cross-browser compatibility, particularly in older environments.
- Polyfills are useful in bridging compatibility gaps, enabling the emulation of modern JavaScript features in browsers that lack native support. This empowers developers to write code that works seamlessly across different browser versions.
- Polyfills may encounter the this binding problem, wherein the correct referencing of this becomes challenging. This can be fixed using the bind() method, allowing developers to explicitly set the context and ensure proper this binding.
- The bind() is used for creating a new function with a specified context and arguments. Its ability to delay execution and enhance flexibility makes it useful in creating more adaptable and scalable JavaScript solutions.
- Immediate execution of functions with a specified context is achieved through the call() and apply() methods. These methods allow dynamic context manipulation and argument passing, contributing to the versatility and adaptability of JavaScript code.