What is Call by Value and Call by Reference in Python?
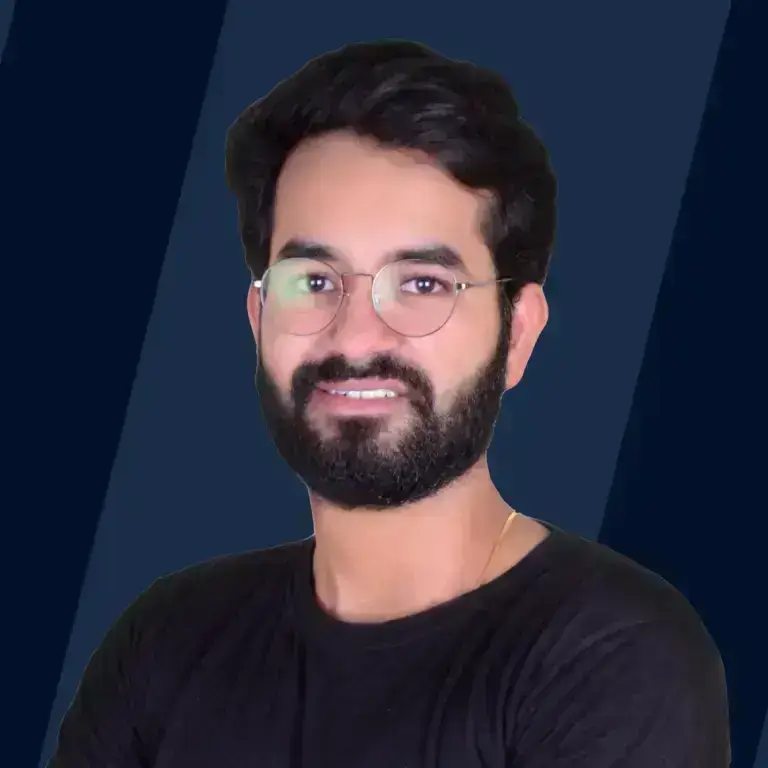
Call by Value
It is a way of passing arguments to a function in which the arguments get copied to the formal parameters of a function and are stored in different memory locations. In short, Any changes made within the function are not reflected in the actual parameters of the function when called.
Call by Reference
It is a way of passing arguments to a function call in which both the actual argument and formal parameters refer to the same memory locations, and any changes made within the function are reflected in the actual parameters of the function when called.
If we consider call by value and call by reference in Python, then we must keep in mind that,
Python variables are not storage containers. Rather Python’s variables are like memory references. They refer to the memory address where the value is stored.
Hence, we should know about Mutable and Immutable Objects.
Mutable objects
An object whose internal state can be changed is called a mutable object. Examples of Mutable objects are Lists, Sets, Dictionaries, bytes and arrays. User-defined classes can be mutable or immutable, depending on whether we can change their internal state.
Note- All operations using mutable objects may or may not change the object.
Immutable objects
An object whose internal state cannot be changed is called an immutable object. Examples of immutable objects are Numbers(int, float, bool, etc), Strings, Tuples, Frozen sets(mutable version of sets are termed as Frozen sets)
Note-All operations using immutable objects make copies.
Code Snippet explaining mutable and immutable objects
Output and Explanation:
Now that we know what Mutable and Immutable Objects are, Lets delve into What is Call by value and Call by reference in Python.
Call by Value in Python
When Immutable objects such as whole numbers, strings, etc are passed as arguments to the function call, the it can be considered as Call by Value.
This is because when the values are modified within the function, then the changes do not get reflected outside the function.
Example showing Call by Value in Python
Output:
Explanation: As we can see here, the value of the number changed from 13 to 15, inside the function, but the value remained 13 itself outside the function. Hence, the changes did not reflect in the value assigned outside the function.
Call by Reference in Python
When Mutable objects such as list, dict, set, etc., are passed as arguments to the function call, it can be called Call by reference in Python. When the values are modified within the function, the change also gets reflected outside the function.
Example 1 showing Call by reference in Python
Output:
Explanation: Here we can see that the list value within the function changed from [1] to [1, 3, 1] and the changes got reflected outside the function.
But, Consider this example-
Example 1 showing Call by reference in Python
Output:
Unlike previous example above, new values are assigned to myList but after function call we see that all the changes do not get reflected outside the function.
Then, is it Call by reference?
Lets take a look into the memory environment for both the examples. We'll print the address of the list before calling the function and after calling the function.
For example 1
Output:
Explanation: Here we see the value of the list before calling the function, within the function and after calling the function gets stored in the same memory address. Hence, changes made within function gets reflected outside the function as well.
Lets consider Example 2 now:
Output:
Explanation: Here we see that, the address of the list changes when new values are assigned to it within the function but outside the function, the value still refers to the previous memory allocation.Hence, the changes made within the function do not get reflected outside the function.
What do we infer? Well, we see that when mutable objects are passed as arguments to a function then there are two cases:
a.) Change is reflected outside function This is when a mutable object is passed as argument and no new value is assigned to it, ie.,the values refer to the same memory address.
b.) Change is not reflected outside function This is when a mutable object is passed as argument and new values or datatype is assigned to it within the function,ie. when the values outside the function and the values assigned within function refer to different memory addresses.
Note: |
---|
In python the changes in values before and after function call solely depends on the memory address.There is object reference depending on memory environment. |
Arguments are passed by assignment in Python. Since, assignment just creates references to objects, there’s no alias between an argument name in the caller and callee. |
Difference between Call by Value and Call by Reference
Call by Value | Call by Reference |
---|---|
1. The arguments are copied to the function parameter. | The arguments, as well as the formal parameters, refer to the same location. |
2. Changes made inside the functions are not reflected. | Changes made inside the functions are reflected. |
3. Immutable Objects are passed in arguments. | Mutable objects are passed in arguments |
Conclusion
- Depending upon the mutability/immutability of it's data type, a variable behaves differently.
- If a variable refers to an immutable type, then any change in its value will also change the memory address it refers to. Still, if a variable refers to a mutable type, any change in the value of the mutable type will not change the memory address of the variable.
- Arguments are passed by assignment in Python. The assignment creates references to objects.
- Hence, Immutable objects passed as arguments are referred as in Call by Value and Mutable objects, when passed as arguments are referred to as Call by Reference.