ceil() in C++
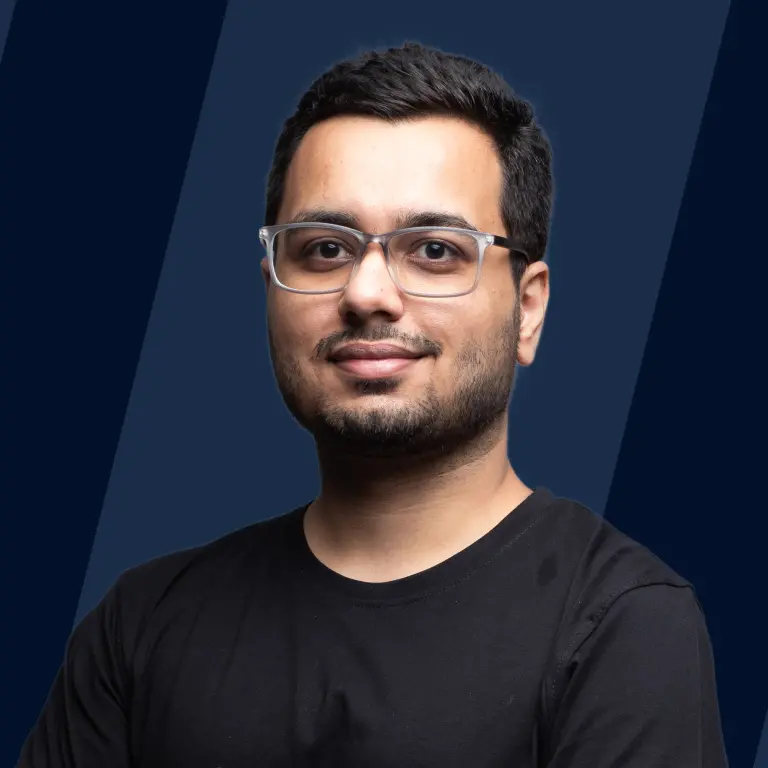
The ceil() function defined under the cmath header file in C++ returns the smallest integer value greater than or equal to the given number. For example, the ceil of 14.2 is 15. ceil in C++ takes a floating-point number as an argument and returns the smallest integer, which is greater or equal to a floating-point number.
The syntax of ceil in C++ is as follows :
The syntax is simply passing a double as an argument to the ceil in C++.
Parameters of ceil() in C++
The ceil function in C++ takes a floating-point value like double, float, etc, as a parameter whose ceil has to be calculated. Doubles are floating-point numbers with double precisions compared to a floating-point number.
Return Value of ceil() in C++
The ceil in C++ returns the smallest possible integer value, which is greater than or equal to the parameter passed to the ceil() function in C++.
Example
Here is an example of the ceil() function in C++.
Code:
Output:
Explanation:
In the above example, we included the cmath header file. The ceil() function in C++ is included under the cmath header file in C++. The variable num of the double data type is initialized with a value of 19.25. The num variable is passed as the parameter to the ceil() function. The ceil() function returns the ceiling of the value. That is the least integer number greater than or equal to the given number. The returned value is stored in the result variable, which is of double type.
What is Ceil in C++
The ceil of a number can be understood better with a number line. An integer or a round number that comes to the exact right of a given number is the ceil of the given number.
The ceil function takes a floating-point value as a parameter. The function returns the ceiling value of the number entered as a parameter.
More Examples
Example 1
- ceil in C++ for Positive numbers.
Code:
Output:
Explanation:
In the above code,
- We passed 2.25 as a parameter to the ceil function.
- The ceil in C++ returns 3, the smallest integer value greater than the number passed as the parameter.
Example 2
- ceil in C++ for Negative numbers.
Code:
Output:
Explanation:
In the above code,
- We passed -1.34 as a parameter to the ceil function.
- The ceil in c++ returns -1, the smallest integer value greater than the number passed as the parameter.
- As we know that -1 is greater than -2. Therefore, -1 is returned by the ceil function.
Example 3
- ceil in C++ for Positive Integral Types.
Code:
Output:
Explanation:
In the above code,
- We passed 15 as a parameter to the ceil function.
- The ceil in C++ returns 15, equal to the number passed as the parameter.
- The number passed as a parameter is an integer (round number). Therefore the number returned by the ceil function is the same as the number passed as the parameter.
Example 4
- ceil in C++ for Negative Integral Types.
Code:
Output:
Explanation:
In the above code,
- We passed -1 as a parameter to the ceil function.
- The ceil in C++ returns -1, which is equal to the number passed as the parameter.
- As the number passed as a parameter is an integer (round number). Therefore the number returned by the ceil function is the same as the number passed as the parameter.
Example 5
Code:
Output:
Explanation:
In the above code, we passed -0.23 as a parameter to the ceil function. The next nearest round number to -0.23 is 0. In the output, we got -0 as a result. The next question which pops into our mind Is 0 and -0 the same? The answer is Yes. Signed and unsigned zeros are the same.
Conclusion
- The ceil in C++ is included in the cmath header file.
- The ceil function takes a double value as a parameter.
- The ceiling of a number is the smallest integer number that is greater than or equal to the given number.
- The ceil function returns the ceiling value of the number passed as the parameter.