C++ Program to Convert Char Array to String
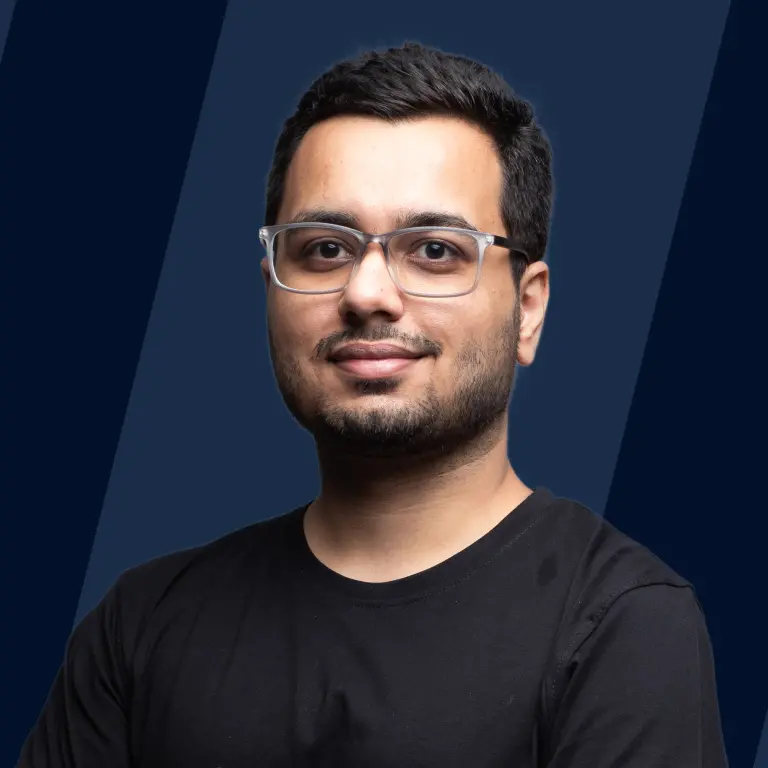
Overview
In C++, the built-in string class contains multiple built-in methods that enable the implementation of various functions much easier than dealing with a character array. So it is better to convert a char array to a string in C++ to have a simpler and faster implementation of programs.
Introduction
Char in C++:
The data type character stores characters. The keyword char denote the character data type. Characters are normally 1 byte in size and range from -128 to 127 or 0 to 255.
Strings in C++:
Strings store a series of characters (text). This is not a built-in type, although it functions similarly in its most basic application. String values must be enclosed in double quotation marks.
Example:
Output:
The String class offers a variety of functions that enable various string operations. Character arrays don't have many built-in methods for manipulating strings. As a result, converting a char array to a string is frequently advantageous for reducing code complexity. Click here to learn more about Strings in C++.
Program to Convert Char Array to String Using For Loops
We can also convert a char array to a string in C++ using the' for' loop.
We can transform a character to a string by following these steps:
- The Character Array is declared first, then its size is assigned.
- Then we declare two variables, one string and the other int.
- Following that, we can utilize the for loop by assigning 0 to the int variable, which will have less value than the array size, and increasing the int variable value by one at each iteration.
- Before displaying the string variable, we must save the value in the string variable on each iteration.
Syntax of "for" Loop
Expression : 1
It is the initialization statement. It is performed only once before the instructions in the block are executed.
Expression: 2
It is the condition checked after each iteration before executing the block of code.
Expression : 3
It is the update statement executed after each iteration.
Example
Output:
Explanation
In the above program, we scan each character array member and append it to our result string using the + operator. In this manner, the length of our result string increases by one at a time, and after traversing all of the members of the character array, we obtain our final string and convert the char array to string C++.
Complexity Analysis
Time Complexity:
We are traversing every element of the character array only once. So our for loop runs n times (n here refers to the size of our character array), and in each iteration, we are just appending a single character at the end of our result string, which is a constant time operation. Hence our overall time complexity is to convert a char array to string C++.
Space Complexity:
In our above program, we only need an additional string variable of length n to store and display our result. Therefore space complexity of the program is .
Program to Convert Char Array to String Using the '=' Operator
The '=' operator is the most straightforward way to convert a char array to a string in C++. It assigns our character array to the string variable as it does for other data types. As a result, this program:
- Construct a character array first.
- Use the '=' operator to assign it to a string variable.
- Output the resultant string.
Syntax
Example
Output:
Explanation
In the above program, we assign our character array to a string directly and output the result by converting the char array to string C++.
Complexity Analysis
Time Complexity:
Assigning a char array to a string is a constant time operation. Hence overall time complexity is .
Space Complexity:
In our above program, we only need an additional string variable of length n to store and display our result. Therefore space complexity of the program is .
Program to Convert Char Array to String Using Inbuilt std::string Constructor
The std::string class includes an inbuilt constructor that handles the job for us. As input, this constructor expects a null-terminated character sequence. However, we can only use this function when declaring a string, and it cannot be used again for the same string since it calls a constructor that is only invoked when we define a string.
Steps:
- Get the size of the character array.
- Declare a string (an object of the string class) and pass the character array as an argument to its constructor.
- Use the following syntax:
- Output the resultant String.
Syntax
Example
Output:
Explanation
In this program, we call the inbuilt constructor of the String class in C++ by passing the character array as a parameter. It converts the character array to string and assigns it to our string variable.
Complexity Analysis
Time Complexity:
This inbuilt constructor takes time to convert a char array to a string in C++ because the entire char array needs to be traversed to convert it into a string.
Space Complexity:
In our above program, we only need an additional string variable of length n to store and display our result. Therefore space complexity of the program to convert a char array to string c++ is .
Program to Convert Char Array to String Using Custom Function
Char array in C++ can also be converted to string using custom functions by following these steps:
- We must first build a custom function with two arguments and have a string as the return type.
- We then define two variables within the custom function: one of type string and the other of type integer.
- Then, we utilize the for loop, where we assign 0 to an int variable, check whether the size of the int variable is less than the array size, and increase the value of the int variable by one at each iteration.
- The string will be returned by the function.
- We define the character array and its size for the primary function, then pass the character array and its size to the custom function.
- Finally, we output the string variable that holds the custom function's returned value.
Function Prototype of Custom Function
Example
Output:
Explanation
In the above program, we have created our function fun, which takes a character array and its size as an argument and returns a resultant string to the main function. In fun, we run a loop to traverse all the characters in a char array and append them to the resultant string individually. After iterating through the entire car array, we have our final string in the res variable, and thus, we return it to our main function and convert the char array to string C++.
Complexity Analysis
Time Complexity:
Here also, we are traversing every element of the character array only once. So our for loop runs n times (n here refers to the size of our character array), and in each iteration, we are just appending a single character at the end of our result string, which is a constant time operation. Hence our overall time complexity is in this case for the conversion of char array to string C++.
Space Complexity:
In our above program, we need two additional string variables. Both the strings are of length n. Therefore space complexity of the program is still .
Program to Convert Char Array to String Using std::stringstream Function
stringstream :
A stringstream connects a string object to a stream, allowing you to read from it as if it were a stream (like cin). Stringstream requires the inclusion of the sstream header file. The stringstream class comes in handy when processing input.
Two methods of stringstream that will be used here are:
- >> (operator) :
To get something from the stringstream object. - << (operator) :
To add a input to the stringstream object.
In C++, you can use std::stringstream to convert a character array to a string. This function inserts the input character into a buffer and retrieves the character from the buffer as a string using std::string.
Steps:
- Create a string variable as well as a stringstream object.
- Insert the supplied char array into the buffer.
- Using the stringStream variable, take characters from the buffer as a string.
- Output the result variable.
Syntax
Example
Output:
Explanation
Here in the above program, we first add a char array to the stringstream object using the << operator and then extract the added input as a string using the>> operator.
Complexity Analysis
Time Complexity:
Since we are adding and extracting all the characters only once so the overall time complexity of our program is .
Space Complexity:
In our above program, we only need an additional string variable of length n to store and display our result. Therefore space complexity of the program is .
Program to Convert Char Array to String by Assigning String Literal to the Char Array
A character array with a list of characters can also be readily transformed into a string variable by directly assigning the character array to the string variable to convert the char array to string C++.
Syntax
Example
Output:
Explanation
Here in the above program, we directly assign the character array to the string literal. The character array ['W', 'E', 'L', 'C', 'O',' M', 'E'] is converted to a string and is assigned to string variable str.
Complexity Analysis
Time Complexity:
Since we directly assign character array to string literal. So it takes constant time. Hence the time complexity of the program is .
Space Complexity:
In our above program, we don't need any additional string to store our result because we directly assign a character array to a literal string. Therefore space complexity of the program is .
Program to Convert Char Array to String Using string.append() Function
To convert a char array to a string, construct an empty string and then append the char array to this one. The string is now made up of characters from the char array, and we converted the char array to string C++.
Steps:
- Include all necessary libraries.
- Define the char array to be converted to a string.
- Use the string.append() method.
- Output the result string.
Syntax
Example
Output:
Explanation
Here in the above program to convert a char array to a string in C++, we have directly used string.append() function from the string class to convert the char array to string. It accepts a char array and converts it to a string.
Complexity Analysis
Time Complexity:
The time complexity of our above program depends entirely on the time complexity of the append function of the string class. And append function's time complexity is . Hence the time complexity of our program is also .
Space Complexity:
In our above program, we only need an additional string of length n to store and display our result. Therefore space complexity of the program becomes .
Program to Convert Char Array to String Using "memmove" Function
memmove() function copies a memory block from one place to another. It's defined in string.h.
A simpler method to convert a char array to a string in C++ is to transfer char* data to an initialized string container. In this case, you must know the length of the char array ahead of time to send it to the memmove method.
Steps:
- Include all required libraries.
- Define the length of the char array (denoted by l here in this program).
- Initialize the result string and set its length to l.
- To replicate a data block from one memory location to another, use the memove function.
- Output the result string.
Syntax
Example
Output:
Explanation
In the above program to convert a char array to a string in C++, we have used the memove function, which copies blocks of memory from one place to another.
Complexity Analysis
Time Complexity:
The time complexity of our above program depends entirely on the time complexity of the memove function. And memmove function's time complexity is because its running time grows proportional to the number of bytes required to move. Hence the time complexity of our program is also .
Space Complexity:
In our above program, we only need an additional string of length n to store and display our result. Therefore space complexity of the program becomes .
Conclusion
- Char in C++:
The data type character stores characters. - Strings in C++:
Strings store a series of characters (text). - Need:
The String class offers a variety of functions that enable various string operations, but character arrays don't have many built-in methods for manipulating strings. - Methods to Convert char array to a string :
- For Loops.
- ‘=’ Operator and String Class.
- Inbuilt std::string Constructor.
- Using Custom Function.
- Using std::stringstream Function.
- By Assigning String Literal to the Char Array.
- Using the string.append() function.
- Using the memmove function.