Char Array to String in Java
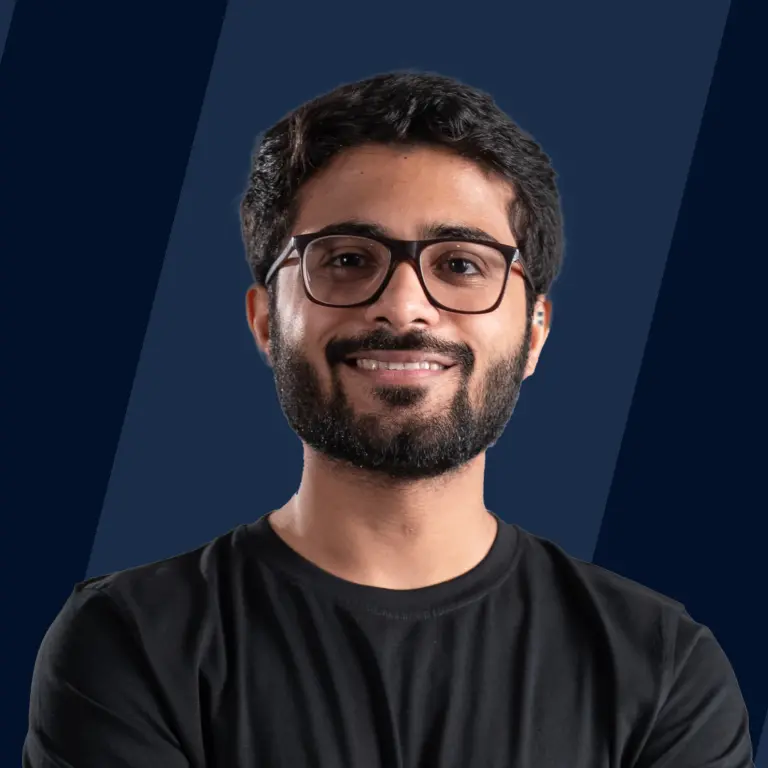
Overview
A string is nothing but a sequence of characters. Programs use strings for various purposes such as storing data, performing operations, taking input, and displaying results. An array of characters is a homogeneous array that stores characters. It is sometimes necessary to convert character arrays into strings and vice versa, depending upon requirements.
How to Convert Char Array to String in Java?
Character is one of the eight primitive data types in Java. The char data type is a single 16-bit Unicode character. A character array in java is a collection of characters as shown below:
The arr variable is a char array that stores all the elements with a character data type.
String in Java is an object of the java.lang.String class. Strings can also be defined as an array of characters. The main difference between a char array and a string in java is that the string is terminated with a special character \0 which is also called the null value. It marks the end of the string.
The s variable represents a Java String with value "Hello".
A string in java can be converted into a character array and we can also convert a character array into a string. In this article, let's see how we can convert a character array to a string. Following are some examples of conversion of a char array into a string.
Examples
- Input char array: char word[ ] = {'W', 'e', 'l', 'c', 'o', 'm', 'e'}
- Ouput String: "Welcome"
- Input char array: char word2[ ] = {'J', 'a', 'v', 'a'}
- Ouput String: "Java"
The different methods that can be used for the conversion of a char array into a string are as follows:
- Using String class Constructor
- Using StringBuilder class
- Using valueOf() method of String class
- Using copyValueOf() method of String class
- Using Collectors in Streams
All the above methods are discussed in detail along with java programs in the following sections of this article.
Method 1: Using String Class Constructor
String class is a java class found in package java.lang. Arrays of characters can be passed to the constructor of String class, which allocates these characters into the new String.
Syntax:
Here value is the character array.
Example: In this example, we will convert a char array to string in java using the constructor of String class. We will pass the char array to the constructor of the String class while creating an instance of String class.
Note: A constructor is a special method that has the same name as the class it belongs to. When an object of a class is instantiated, its constructor is called. Hence, the constructor will be called by default when the object of the String class is created, thus there is no need to invoke it explicitly.
Output:
Explanation:
- In the above example, the char array ch_arr is passed as a parameter to the constructor of the String class while instantiating the object of the class.
- The constructor converts the character array to a string and we get the required output.
Method 2: Using StringBuilder Class
The char array can be converted to String using the StringBuilder class in java. The StringBuilder class is also located in java.lang package of Java.
StringBuilder also stores a sequence of characters. The major difference between String and StringBuilder is that String is immutable whereas StringBuilder is mutable. This means that we can change or update an instance of StringBuilder class, unlike String.
As StringBuilder is mutable we can append the characters from the char array through iterations. At the end of this process, we will get a String that stores all the characters from the char array.
Note: In java, mutable objects are those objects whose value can be modified. In the case of mutable objects, the changes are reflected in the same existing object, and no new object is created.
Immutable objects are those objects that cannot be changed once created. Every change done in these objects creates a new object.
Syntax:
StringBuilder can be declared as follows:
Example:
In this example, we will iterate through the char array and append all its elements in StringBuilder class. This will convert the char array to string in java which is our required output.
Output:
Explanation:
- In the above Java program, sb_obj is an instance of StringBuilder class.
- The for() loop is used to iterate through the character array ch_arr and all the elements of ch_arr are added to sb_obj using the append() method.
- As StringBuilder is mutable we can change its value and the append() method adds every new element at the end.
- As we iterate through the array, the code above will have a time complexity of O(n).
- Moreover, the code will create the defensive copy of the array while converting it into a string, causing the space complexity to be O(n). Here n represents the size of the character array to be converted.
Method 3: Using valueOf() method of String class
The string class has some predefined methods that can be used to convert character arrays to strings. The valueOf() method is a static method present in the String class that converts different types of values like characters, float, int, double, boolean, and even objects to string. This method takes a char sequence or any other sequence as a parameter and returns a string.
Syntax:
Here a is the character array.
Note: An instance of a static method belongs to the class rather than the instance of the class. A method is accessible to every instance of a class, but methods defined in an instance are only accessible to that object.
Example: Here we will use the same valueOf() method to convert char array to string in java.
Output:
Explanation:
- In this example, the character array ch_arr is passed as a parameter to valueOf() method of the String class.
- The valueOf() method converts the array and returns String s1.
Method 4: Using copyValueOf() method of String class
Another method of the String class that can be used to convert a char array to String in java is copyValueOf(). This method is also a static method of the String class. Just like the valueOf() method, this method also accepts an array of characters as an argument and returns a string with the character sequence.
The copyValueOf() method differs in its functionality, as it simply copies characters from the array. In contrast to the valueOf() method, which works with a variety of data types and objects, copyValueOf() is only used for character arrays.
Syntax:
Here chr is the character array.
Note: An instance of a static method belongs to the class rather than the instance of the class. A method is accessible to every instance of a class, but methods defined in an instance are only accessible to that object.
Example1: Here we will use the copyValueOf() method to copy contents of char array to string in java.
Output:
Explanation:
- In the above example, the character array ch_arr is passed as a parameter to the copyValueOf() method of the String class in java.
- The method copies the elements from ch_arr and returns in form of string s2.
A substring can also be extracted from a character array using the copyValueOf() method. Later on in this section, we will see an example of the same.
Syntax:
The parameters in the above syntax are described below.
- chr : The character array.
- index : The starting position of array from which copy is to start.
- num : Number of elements that has to be copied.
Example2: In this example we will extract a substring from a char[] array using the copyValueOf() method.
Output:
Method 5: Using Collectors in Streams
In Java8 a new package was introduced called java.util.stream. This is also known as Stream API and is used to process collections of objects. Collectors is a class in java.util.stream package that is used with Stream API implementing the Collector interface and Stream API.
We can use the same Collectors class to convert a char array to string in java. First, we will collect all the array elements (chars) using the collect() method of the Stream class and then return it as a single string using the joining() method of the Collectors class.
Example: Here, we will use methods from the Stream class and Collectors class to convert char array to string in java.
Output:
Explanation:
In the above example, various methods of the Stream and Collectors class are used, let's understand their functionality.
- Stream.of(): This method returns a Sequential Stream with the specified elements.
- Stream.map(): This method returns a stream that consists of the results of applying the specified function to the elements of this stream.
- Stream.collect(): This method returns the results of operations performed on the stream.
- Collectors.joining(): This method of Collectors Class combines various elements of a character or string array into a single string object.
Now, let's refer to the above explanation of all the methods and understand the flow of execution of the above code.
- Character array ch_arr is converted into the sequential stream using Stream.of method.
- New string arr is returned to the stream using map() method. Collectors.joining() method is used to join various elements of a char array into a single string object.
- This string is returned into variable string_op using Stream.collect() method.
Conclusion
- The String is an array of characters, terminated with the special character "\0".
- A char array can be converted to a string and vice versa.
- A string from a character array can be obtained by using String class methods, String class constructor, StringBuilder, Streams, and Collectors class in Java.
- StringBuilder is a mutable class in java. Its instance can be modified without creating a new instance in the memory.
- The valueOf() and copyValueOf() methods of String class can be used to convert a char array into a string.
- The append() method of StringBuilder class can be used to append each character of the character array to a StringBuilder class instance.
- Character array can be converted into a string using the Collectors class of Stream API.
- Though conversion of char array to string in java can be done in multiple ways the result will be the same i.e., a string.