C++ Program to Convert char to int with Examples
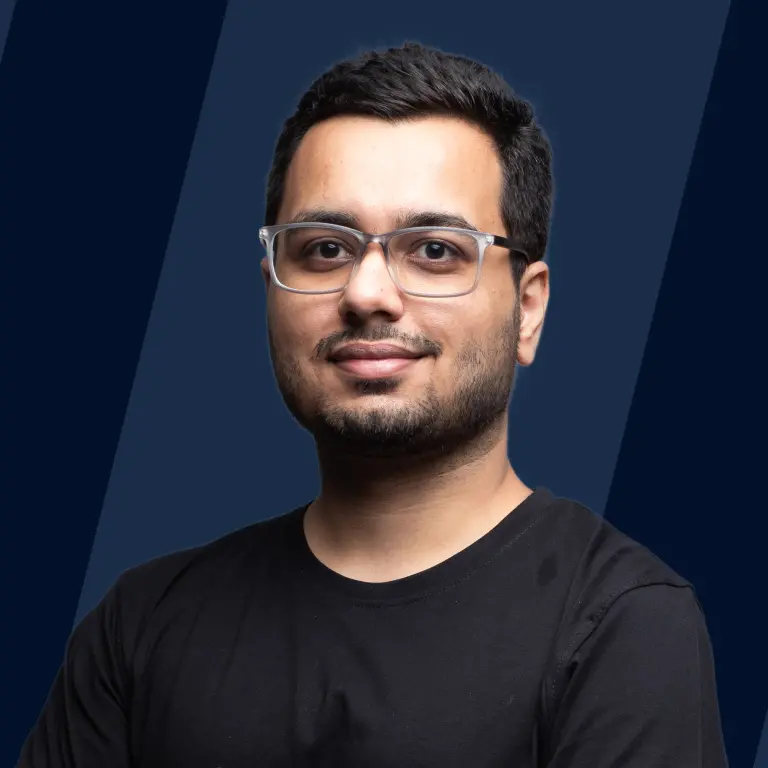
Overview
Converting a char to int C++ is required in many programs and projects. This conversion can help when integer values have the character data type, which may happen if they are a part of the string. In cases where we want to do some integer operations over them, getting those integer values as integer type would be required only. Converting any character to an integer type will transform the character value to its corresponding ASCII value. (For example, ‘A’ converts to 65).
Different Ways to Convert char to int in C++
There are many ways to convert a char to an int in C++. We will discuss the following :
- Using Typecasting
- Using static_cast.
- Using sscanf()
- Using stoi()
- Using atoi()
- Using string stream
Using Typecasting
Typecasting is a feature provided by C++ to change the type of any variable for just that statement (i.e., not permanently).
Method - 1
Any character can be typecasted to an integer, and its value is converted into its corresponding ASCII value (an integer).
For example :
Output :
Here, the char variable type a is converted to int and its value to the corresponding ASCII value, i.e., 65. This typecasting is valid only over the casted variable in that particular statement. When we try to print the variable's data type in the following line, it becomes a character only (c in the output).
We could have directly printed (int) an also.
Method - 2
If we want to convert a char to an int value to an integer with the same value rather than the ASCII value, we can do the following :
Output :
This was possible because operations like addition or subtraction on characters are applied to their ASCII values. Subtracting 0 from variable a means subtracting the ASCII value of 0 from that of the integer value in variable a. This will give us the number concerning 0, what we wanted.
Hence, the above code is equivalent to :
Output :
Using static_cast
static_cast is another function provided by C++ for converting data types of variables. The program to convert a char to int in C++ using static_cast is given below :
Output :
Note that no special library must be included to use static_cast.
Using sscanf()
The sscanf() function in C is used to read a value from the console and assign the value in the variable provided in the argument.
Similarly, the sscanf() function reads the value not from the console but from the character array (represented through the pointer) provided in the arguments.
The argument also contains the data type to convert and the variable to store the converted value in.
sscanf() operates over a character array and converts it into an integer with the same value, there is no role or use of ASCII codes in this case, and similar is the case whenever a string or array of characters is being operated upon.
For example, "1526" is converted into 1526 (one thousand two hundred and thirty-four). The below program demonstrates the use of sscanf for converting char to int :
Here “%d” refers to the integer data type, and the statement says that the value at a is stored at the address of x as an integer.
Output :
In cases where the character array cannot be converted into an integer because it contains some non-integer values, the result of the conversion will be a garbage value.
This case is shown in the program below :
Output :
If the array begins with integer values and then contains non-integer values (hence, still non-convertible), only all the beginning integer values are converted and stored in the variable.
For example : for “12ab23”, integer 12 will be stored.
Using stoi()
The stoi() function provided by C++ converts a string to an integer value (string to int: stoi).
For example, if the string is “3425”, the integer will be 3425 (Three thousand six hundred four).
Below is the piece of code showing the conversion from string to int using stoi() :
Output :
A character pointer can also be used in the above program instead of a string.
Note : There is no role of conversion into ASCII here either. Here the code will produce an error if a string with non-integer values is provided as an input to the stoi() function.
Using atoi()
atoi() is a function similar to stoi() but is a part of C (hence C++, too, though), and that’s why it supports character arrays only and not strings.
Moreover, if the string is not convertible into an int, atoi() will silently fail, returning a 0, whereas stoi() will simply throw an exception.
Below is the program to show char to int conversion using atoi() :
Output :
Using stringstream
StringStream is a class in C++ derived from the iostream class. You can read from a string as though it were a stream (like cin) with the help of the stringstream class, which links a string object with a stream. This will be better understood from the following program.
We must include the sstream header file to utilize stringstream. When processing input, the stringstream class comes in quite handy. Below is the program to convert char to int using sstream class.
Output :
The string is read as a stream and stored in variable a, and then that stream is output in the integer variable n, converting the string to an integer.
For strings that cannot be converted into integers, its behavior is similar to sscanf(). It will convert the integer values in the beginning (before the first non-integer value), if any, and ignore the others. If there’s none, it returns 0.
Converting char Value to int by Adding 0
To convert char to int by adding 0, we initially typecast the character to an integer using int() and later add 0 to the typecasted value, which converts a character to an integer.
For example :
Output :
Here we first typecast the character to an integer using int() and later add 0 to the typecasted value, which converts a character to an integer. As in the above example, the ASCII value of 'b' is 98.
Example with Full Code in C++
Output :
Conclusion
In this article, we discussed various ways by which we can convert a char to an int in C++.
- Any of these ways can be used depending upon the application, for example, if a string of characters is to be converted to an integer or a single character.
- Also, whether it is required to throw an exception on having a non-integer string can also determine which method to use.
- The use of ASCII values also differs in these methods.
Hence, knowing these factors will help decide which method to use.