Convert Char to String in C++
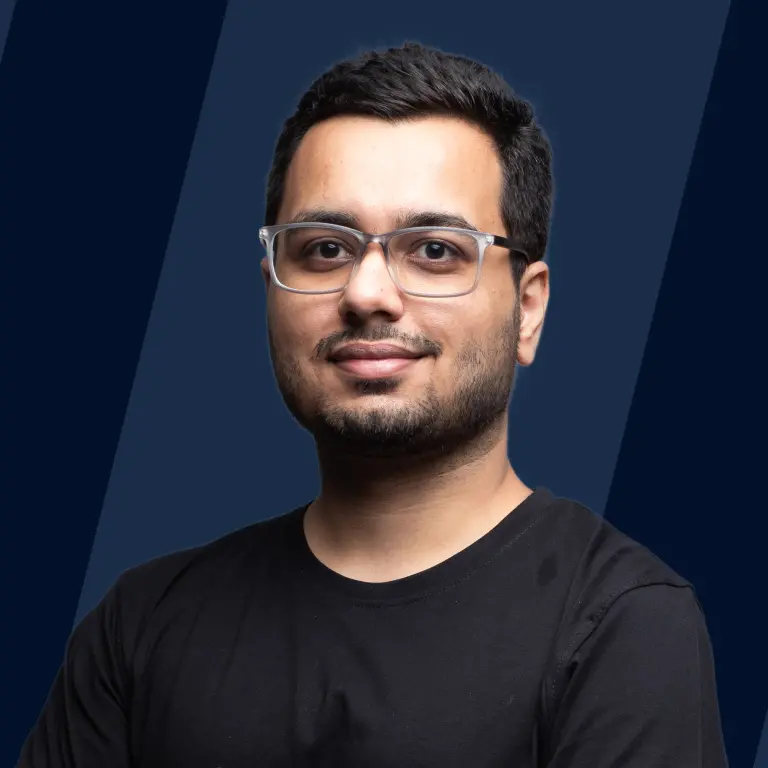
In programming, many times the user may need to convert char to string C++, for example, for calling a function we need the string to pass as a parameter but we have currently a character so in this case, the user needs to convert character to string first and it will be possible to call the function.
To convert char to string C++ we have many methods, in some of them we need the empty string to add the character like the += operator method, pushback method, append method, etc. On the other hand, many of them don’t require an empty string they will first make the string empty and then add the new character some of them are: assign method, replace method, etc.
Let’s discuss the methods to convert char to string in C++
1. Using the = Operator
As the string is the collection of the characters there is the possibility of the string containing a single character. The assignment operator or = is used to store the result of the expression on its right side to the left side variable or data structure. If we assign a single character to a string then the string will contain only a single character which will eventually change of data type of character.
Let's see the syntax to convert char to string using the assignment operator:
Syntax:
In the above syntax, we have simply assigned a character/character variable to the string and it will be stored in the string variable. Also if the string is non-empty then previous data will be updated by only a single given character.
Note: String must be initialized before assigning a character to it.
Let’s see a code to implement the above syntax and get more understanding:
Example: In this example, we are going to create a single-character variable and a string and by using the assignment operator we will assign a character to a string that will store a single character in the string.
Output:
Explanation: In the above code, we created a single character variable and a string in C++ and by using the assignment operator assigned char to string which eventually changed the data type from char to string.
2. Using the '+=' Operator
Converting char to string C++ using the += operator is similar to converting char to string C++ using the assignment operator the only difference is string must empty if we are using the += operator.
Let's see the syntax to convert char to string using the += operator:
Syntax:
Or
In the above syntax first, we simply declared a string and assigned it an empty string, and later added a character to it while in the second case we assigned an empty string to a string containing some value and then added a character to it.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we are going to create a single character variable and a string and by using the assignment operator we will assign an empty string to it then we will implement both the above-discussed syntax.
Output:
Explanation:
In the above code, we created a single character variable and a string in C++, and by using the assignment operator assigned an empty string to the declared string then by using the += operator we added a character to the string. As now string contains a character we first made it empty then again added a character to the string and printed it.
3. Using std::stringstream Function
stringstream is defined in the sstream header file allowing the string to read from the stream similar to the cin or input stream. We can write any type of primitive data type value in the stringstream object and read the content of the stringstream object (which is a single character) through the string or simply say data from the stringstream object can be read with the help of a string.
Let’s see the syntax to convert char to string C++ using stringstream:
Syntax:
In the above syntax, we have used the insertion operator (<<) to insert a character to the object of the stringstream then we used the extractor operator (>>) to extract the data that is character into a string. The advantage of converting char to string C++ using stringstream is we don’t need to initialize a string. Also, if the string is initially containing some value then it will be automatically deleted and the string will only contain the provided character.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will create an object of stringstream and then add a character to stringstream object using the insertion operator. In the end, we will read object data with the help of a string and print it.
Output:
Explanation:
In the above code, first, we included the sstream header file then in the main function created an object of stringstream. By using the insertion operator we added a character variable to an object of the stringstream class and later extracted it by using the extraction operator and string variable. In the end, we printed the character stored in the string.
4. Using Inbuilt std::string Constructor
As we know the string is defined in the string header file where a class is defined for the string data type which provides the option of constructors to initialize the string object or variable. There is a predefined constructor that takes two parameters one is an integer and another is a character this will initialize a string of the size provided integer and every character of the string is equal to the provided character. If we pass string size as one then it will eventually convert char to string.
Let’s see the syntax to convert char to string C++ using an inbuilt string constructor:
Syntax:
In the above syntax, we declared a string object and initialized using a constructor of size n and a given character. We need only a single character so we will use n equals 1.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will create an object of string and then pass one as size and character to its constructor.
Output:
Explanation:
In the above code, we implemented the above-discussed syntax that uses a string class constructor. We passed 1 as the size and passed the character which converted into the string.
5. Using string::assign() Function
The assign function is the inbuilt function of the string class defined in the string header file and assign function is used to replace the previous value of the string with the provided number of similar characters.
Let’s see the syntax to convert char to string C++ using the assign() function:
Syntax:
In the above syntax, we assigned the string object a new value which is n copies of the provided character.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will create a character and an object of string and then assign the string a single copy of the given character using the assign function.
Output:
Explanation:
In the above code, first, we declared the character and the string variable then using the assign function assigned string 1 copy of the provided character.
6. Using tring::push_back Function
STL library provides the facility of the push_back() function and the string class also supports it, as the name suggests push_back() function adds a new character at the end of the string.
Let’s see the syntax to convert char to string C++ using the push_back() function:
Syntax:
In the above syntax, string_variable is the string in which we have to add the new character which is character_variable.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will a string and then going to push a new character in the back of it.
Output:
Explanation:
In the above code, we have declared a string and character variable and by using the push_back() function added a new character in the empty string.
7. Using string::append() Function
Append function of the String class works similarly to its name means it takes two variables, one is the number of characters and another is a character, and adds a given number of times the given character is behind the string.
Let’s see the syntax to convert char to string C++ using append() function:
Syntax:
In the above syntax, is the integer that represents the number of times to copy a given character behind the given string.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will create an object of string and then going to pass one as size and character to the append function.
Output:
Explanation:
In the above code, we declared a character variable and a string variable, then by using the append function by passing one as size and given character variable we converted the character to a string.
8. Using string::insert() Function
The insert function is used to insert a new character in the string at a given specific position and also can add a new character at the end of the string or in the empty string.
Let’s see the syntax to convert char to string C++ using insert() function:
Syntax:
In the above syntax, is the integer that represents the number of times to copy a given character at the provided specific position in the string.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will create an object of string and then going to insert a character at the last by passing one as size and giving the character as parameters to insert function.
Output:
Explanation:
In the above code, we declared a character variable and a string variable, then by using the insert function by passing one as size and given character variable we inserted a character at the 0th position of an empty string and converted the character to string.
9. Using string::replace() Function
Replace function is similar to the insert function with the only difference it deletes the substring from the current position of a given size and adds the new string of the given size which is just a copy of the given character a given number of times.
Let’s see the syntax to convert char to string C++ using replace() function:
Syntax:
In the above syntax, the position is the starting position from where to replace the substring, we have to pass the size of the new string, and the string to remove is the integer which represents the number of times to copy a given character at the provided specific position in the string.
Let’s see a code to implement the above syntax and get more understanding:
Example:
In this example, we will declare a string and a character, and by using the replace function we will first remove characters from the string and then add only a single character to it.
Output:
Explanation:
In the above code, we have declared the character and the string, as our string was empty we used the replace function and there was nothing to replace, we just provided a single character to add to it.
Conclusion
-
char or character is the primitive data type of C++ language which has a memory of 1 byte while string is a data type that can store characters the same as the character array.
-
To convert char to string C++ we have many methods:
- In some of them, we need the empty string to add the character like the += operator method, pushback method, append method, etc.
- In some methods we don’t require an empty string they will first make the string empty and then add the new character some of them are: the assign method, replace method, etc.
-
If we are storing the character in the empty string by any method then the time complexity will be .
-
If the string is non-empty and let’s assume its length is then we have to appropriate method to make it empty first and then we are going to add a character that will take time.