Java String charAt() Method
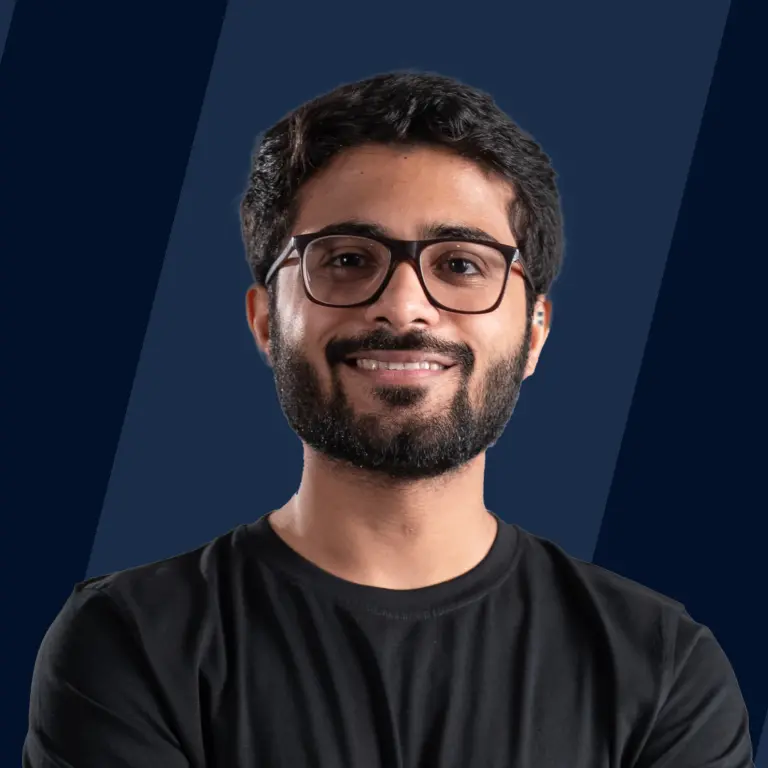
The charAt() method in Java belongs to the String class and enables the extraction of a character at a specific index within a string. With indices starting from 0, charAt() offers a precise way to access individual characters, facilitating various string-related operations. Understanding and leveraging charAt() is essential for tasks involving character-level processing and manipulation within Java applications.
Syntax
The syntax for the charAt() method in Java is as follows:
The charAt() method is invoked on a String object and takes an integer index as its parameter. It returns the character at the specified index within the string.
Parameter Values
index (int): The parameter represents the position of the desired character within the string. Indices start from 0 for the first character and extend to the length of the string minus one.
Return Type
The method returns a single character, which is the character located at the specified index within the string.
Exception
The charAt() method may throw an IndexOutOfBoundsException if the specified index is negative or greater than or equal to the string's length. Handling this exception is crucial to ensuring the program's robustness when using the charAt() method.
Java String charAt() Method Examples
Example 1: Java String charAt() Method
Code:
Output:
Explanation: As can be seen above, we have printed the first and nineteenth characters from the string which says "Learning Java". Here the total length of the character in the string is 12, and we asked to print characters 1 and 7, which fall in the range we are seeing the correct output as assumed. Also, we know that in Java, the index of strings starts from 0 and not 1. Therefore, when we use the chartAt(0), it gives output as L, the first character.
Example 2: Java String charAt - IndexOutOfBoundsException
Let us understand the charAt() Method to understand the IndexOutOfBoundsException using the example below.
Code:
Output:
Explanation:
Here we see that we get StringIndexOutOfBoundsException as the index that we gave was negative and as we already studied for any index that is negative of more than the range of the index for a string we will get this exception.
Example 3: Java String charAt - Print All Characters of the String
Let's understand the charAt() Method for printing all the characters of the string using the example below.
Code:
Output:
Explanation:
Here, we see that we have used the logic of loops in Java to print all the characters of a string. We used the for loop to print each index character from the index value ranging from 0 to lengthofstring()-1. If we exceed that, it will give us a bound exception.
Example 4: Java String charAt - Printing the First and Last Character of a String
Let's understand the charAt() Method for printing the first and last characters of a string using the example below.
Code:
Output:
Explanation:
Here, we are using the charAt() method to print the first and last characters of the string. As we already studied, the index value in Java starts from 0 and not 1; we use the index value as 0 to print the first character and the length of string-1 to print the last character of the string.
Example 5: Java String charAt - Print Characters Presented at Odd Positions
Let us understand the charAt() Method for printing the characters present at the odd places of a string with the help of the example below.
Code:
Output:
Explanation:
Here, we have used the for loop along with charAt() in Java to specify a condition of the index value to give output other than 0, which states that those are odd positions. Once we have those index values, we then print the values of those characters from the string.
Try yourself by modifying the code to print the characters present on the even position.
Example 6: Java String charAt - Counting the Number of Vowels in a String
Let us understand the charAt() Method to the Number of Vowels in a String with the help of the example below.
Code:
Output:
Explanation: Here, we have used charAt() in Java to count the number of vowels in a string.
Example 7: Java String charAt - Counting the Occurrence
Let us understand the charAt() Method to count the Occurrence of a character from a string with the help of the below example.
Code:
Output:
Explanation:
Here, we have counted the occurrence of the character 'S' in the string. We have used the for loop with charAt() in Java to increase the counter value every time it sees the character 'S'. As shown in the code above, we have counted the number of times the letter 'S' occurred in the string, which in this case was 1.
Conclusion
- The method charAt() in Java can be defined as the inbuilt method that returns the character value at the stated index number when searched in a string.
- The syntax of the charAt() in java is as follow:
-
The output for negative integer or out-of-bounds positive integer when passed while executing the charAt() in Java gives the IndexOutOfBoundsException exception.
-
We have studied many examples covering a variety of scenarios where we can use simple charAt() in Java to simplify our process.