cin in C++
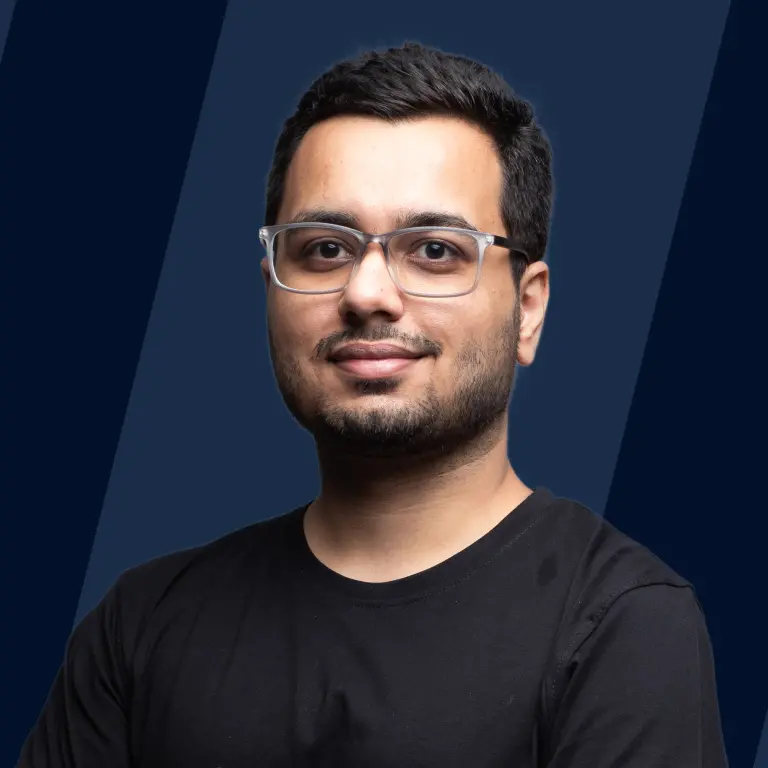
Overview
cin in C++ refers to character input from the user from the input stream. cin in C++ is an object of class istream and it is declared in the iostream header file. cin object helps us to read data from a standard input device like a keyboard with the use of extraction operator (>>) or member functions like getline(), read(), etc. along with the cin object.
Syntax of Cin in C++
Let's see how we can use the cin object in a C++ program with the help of the below-mentioned syntax:
- The syntax for cin if we are not using namespace std; in our C++ program:
Various variables, functions, classes, and other objects in the same scope cannot have the same name. As a result, namespace was created to address this issue. We don't have to use std with cin once we have included the using namespace std statement in our c++ program.
- The syntax for cin if we are using namespace std; in our C++ program:
Note:
- >> is known as the extraction operator.
- var_name is often a variable name, and it can be of any data type. It can also be a component of containers such as arrays, lists, vectors, and so on.
Prototype of Cin in C++
In the iostream header file of the C++ libraries, the prototype of cin is defined as:
In C++, the cin object belongs to the istream class and it is linked to stdin, the standard C input stream. The extern keyword is used to define the external objects.
When an object of type std::ios_base::Init is generated for the first time in a C++ program, it ensures the accurate initialization and destruction of the default C++ input/output stream objects like std::cin, std::cout, and so on.
Exceptions for Cin in C++
- If we are using an extraction operator >> with the cin object, we can only take input from the user until a delimiters like space or enter appears.
Example C++ program:
Input:
Output:
Explanation: We can see from the output that only Have string is stored in the str variable as space is considered as a delimiter with the extraction operator >>.
- If we are using the getline() function with the cin object, we can take input from the user until enter delimiter appears. space is not considered as a delimiter with the getline() method.
Example C++ program:
Input:
Output:
Explanation: We can see from the output that the whole Have a good day! string is stored in the str variable as space is not considered as a delimiter with the extraction operator >>.
Example C++ Program
Let's see how we can use cin in our C++ programs:
Input:
Output:
Explanation: We can use cin and cout objects as we have included the iostream (input/output stream) header file in our C++ program. We are taking an input string (but we can use any data type with cin, for example, int, float, etc.) from the user from the input stream using the cin object and an extraction operator >> and displaying the input in the output using the cout object.
Cin in C++ with Extraction Operator
To accept a stream of characters from the user, the extraction operator >> can be used with the cin object. To accept multiple inputs, the extraction operator >> can be used numerous times with a single cin statement.
Example C++ Program:
Input:
Output:
Explanation: In the above program, we are using a cin object with two extraction operators (one for each variable) to take two integer values from the user, and then we are printing the sum of these two integer values in the output.
Cin with Member Functions
There are various methods available in the istream class which can be used with the cin object to extract the input from the input stream. Member methods like getline(), read(), etc. are used with the cin object. The following are some of the most regularly utilized member functions:
-
It reads a character from the input stream entered from the keyboard and saves it in the var variable.
-
It reads a string stream of length l characters and saves it in the str array.
-
It reads a line (string) of the input until an enter key delimiter appears and saves it in the str variable.
-
It's used to disregard the first n characters from the input.
Examples of cin in C++ with Member Functions
- Using cin.get(char& var);
Input 1:
Output 1:
Input 2:
Output 2:
Explanation: get() method only takes one character from the input stream and stores it in the variable. We can see from the output that S is the only character printed with SA as input.
- Using cin.read(char *str, int l);
Input:
Output:
Explanation: From the above output, we can conclude that only 3 characters string Tru has been taken as an input instead of the full string Truth by using the read() method and passing 3 as the second argument.
- Using getline(cin, str);
Input:
Output:
Explanation: From the above output, we can conclude that the whole string is stored in the name variable, and space is not considered as a delimiter here.
- Using cin.ignore(int n)
Input:
Output:
Explanation: We can conclude from the output that the first five characters (Skill) have been ignored by the compiler from the input stream and only the ful string is stored in the course variable.
Conclusion
- cin in C++ refers to character input from the user from the input stream.
- cin is an object of class istream and it is declared in the iostream header file.
- Character input can be taken using extraction operator (>>) or using member functions like getline() or read() with the cin object.
- We can take multiple inputs simultaneously from the input stream using multiple extraction operators (>>) with the cin object.