Class Cast Exception in Java with Examples
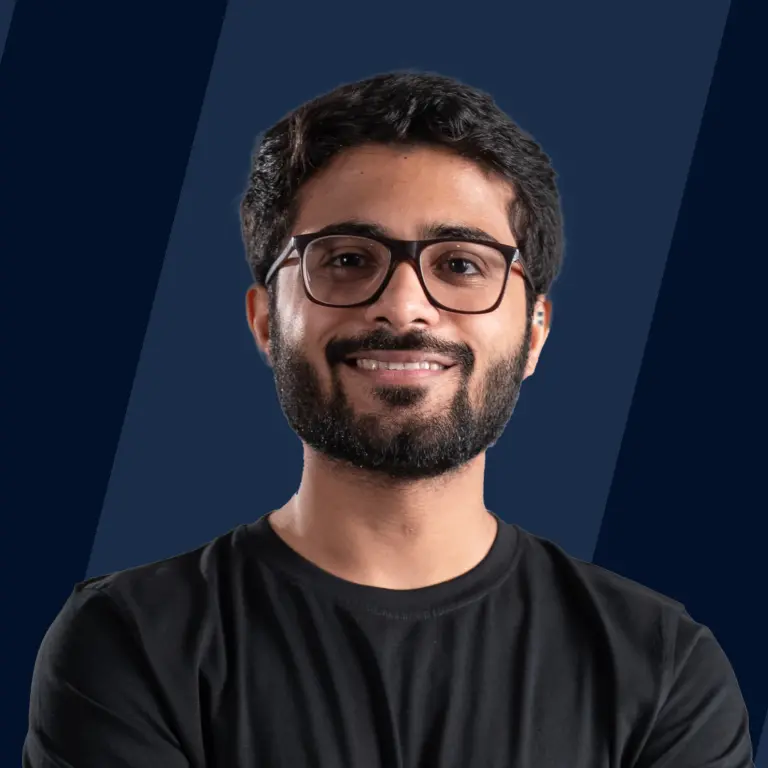
Overview
The Java programming language is known for its robust type-safety mechanisms that help prevent many runtime errors. However, one common exception that developers often encounter is the ClassCastException in Java. ClassCastException in Java occurs when an attempt is made to cast an object to a class type that it is not an instance of. This article aims to provide a comprehensive overview of the ClassCastException in Java, shedding light on its causes, prevention strategies, and effective solutions.
Types of Exceptions in Java
Checked Exception
Checked exceptions are enforced by the compiler and must either be handled with a try-catch block or declared in the method's signature using the throws keyword if they might occur during execution. Examples of checked exceptions include:
- ClassNotFoundException: Occurs when a class is not found.
- IOException: Related to input/output operations.
- SQLException: Related to database operations.
Checked exceptions often involve external factors like files or databases and require graceful handling.
Unchecked Exception
Unchecked exceptions, also known as runtime exceptions, do not require explicit handling but should still be handled for application stability. They are typically caused by programming errors or unexpected runtime conditions. Examples of unchecked exceptions include:
- ArithmeticException: Occurs when performing illegal arithmetic operations.
- ArrayStoreException: Thrown when attempting to store an object of an incompatible type in an array.
It is recommended to handle unchecked exceptions whenever possible to maintain the stability of the application.
ClassCastException in Java
ClassCastException in Java occurs during runtime when an inappropriate type-casting operation is attempted. It signifies a mismatch between the actual runtime type of an object and the target type of the cast.
Example:
Output:
Explanation:
In this code, we upcast a Dog object to an Animal reference and then attempt to downcast it to a Cat reference. Since the actual object is a Dog, the downcast is not valid, resulting in a ClassCastException.
To avoid this, use the instanceof operator to check the object's type before casting:
Output:
This prevents the ClassCastException by checking the object's type before performing the cast.
Causes of ClassCastException in Java
Before discussing the causes of ClassCastException in Java, it's crucial to understand upcasting and downcasting.
Upcasting
Upcasting involves treating a derived class object as an instance of its base class. It's a safe operation since the derived class inherits all properties and behaviours of the base class. The compiler implicitly handles upcasting due to the "is-a" relationship in inheritance. For example:
Downcasting
Downcasting is the opposite, treating a base class object as an instance of its derived class. This is more complex because not all base class instances are genuinely derived class instances. To perform downcasting, explicitly specify the type with parentheses and use the instanceof operator to check compatibility:
Now, let's explore the causes of ClassCastException in Java.
-
Incompatible Type Casting: Attempting to cast an object to an unrelated type in the inheritance hierarchy leads to ClassCastException.
Example:
Here, trying to cast a Fruit object to an Apple reference causes ClassCastException due to incompatible types.
-
Casting to Subclasses: Downcasting to a subclass type without proper verification results in ClassCastException.
Example:
Attempting to cast a Car to a Bicycle reference, even though both are subclasses of Vehicle, leads to ClassCastException.
-
Incorrect Use of Generics: Incorrect usage of generics, such as casting a generic object to an incompatible type, can trigger ClassCastException.
Example:
Here, casting a Box containing Apple objects to a Box of strings results in ClassCastException due to incorrect parameterization.
Avoid these scenarios to prevent ClassCastException in Java. Always ensure compatibility when performing type casting to maintain the integrity of your code.
Handling ClassCastException in Java
Handling ClassCastException in Java involves using strategies to prevent or gracefully handle situations where incompatible type casting occurs. Two common approaches are using the instanceof operator and checking the type hierarchy.
Using instanceof Operator
The instanceof operator allows you to check the type of an object before performing a cast, thus preventing a ClassCastException in Java.
Code:
Explanation:
In this example, before casting animal to a Dog, we use instanceof to verify that animal is indeed an instance of Dog. If the condition is met, we perform the cast and call the makeSound() method. If the condition is not met, we handle the case where the object is not a Dog, thus avoiding ClassCastException in Java.
Checking the Type Hierarchy
By carefully designing the class hierarchy, you can reduce the need for casting and, consequently, avoid ClassCastException in Java.
Code:
Explanation:
Here, the class hierarchy is designed such that each shape type is a subclass of Shape. By checking each subclass type using instanceof, we ensure that the correct casting is performed based on the actual type of the object. The shape object of the Shape class is safely downcasted to a Square object or Circle object, and then using this object, we call the draw() method of the respective subclass.
FAQs
Q. What is ClassCastException in Java?
A. ClassCastException in Java is a runtime exception that occurs when an inappropriate type-casting operation is attempted, such as casting an object to an incompatible class type.
Q. How can you prevent ClassCastException in Java?
A. You can prevent ClassCastException in Java by using the instanceof operator to verify types before casting and by designing class hierarchies and using generics effectively.
Q. What's the impact of an unhandled ClassCastException in Java?
A. An unhandled ClassCastException in Java can lead to program crashes or unexpected behaviour, disrupting the normal flow of execution and potentially causing data corruption.
Q. Is ClassCastException in Java a checked or unchecked exception?
A. ClassCastException in Java is an unchecked exception, meaning it doesn't require explicit handling or declaration, but should be prevented through proper coding practices.
Conclusion
- ClassCastException in Java occurs when incompatible types are cast, revealing the importance of verifying object types before casting.
- ClassCastException in Java happens during runtime, highlighting the dynamic nature of Java's type system and the need for cautious coding.
- Utilizing instanceof checks, designing class hierarchies wisely, and employing generics help prevent and manage situations where ClassCastException in Java can occur.
- ClassCastException in Java is an unchecked exception, demanding vigilance during development to ensure safe type conversions and reliable code.
- Properly handling or avoiding ClassCastException in Java ensures smoother program execution, enhancing application stability and end-user experience.