Class Class in Java
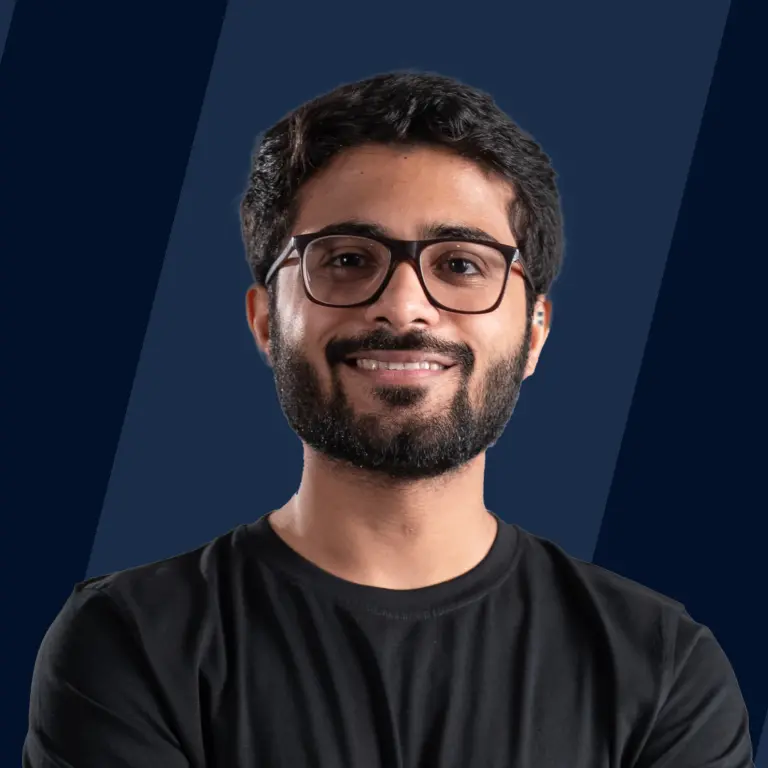
Overview
This article mainly explains the Class class present in java.lang package. This Class class present in Java is mainly used in API (Application User Interface) and also in many Java applications. The methods used for creating the object of this class are explained in the article. The article also explains the several methods present in java.lang.class.
What is Class Class in Java?
java.lang.Class is one of the most important classes in Java and it has many useful methods which are used in Application development like isArray(), isEnum(), etc. They are popularly used for loading Oracle or MySQL drivers in the Java application.
It also provides methods like Class.newInstance() which is the backbone of the reflection class present in Java and it helps us in creating an instance of a class without using the new() operator unlike for other classes. The Class class present in Java has a private constructor, so a new keyword does not help in creating the instance of it, instead of it we use three methods for creating the object of the class. And also the class instance is created by JVM at Runtime when a class is loaded. The object of class Class is also used to represent classes, enum, interfaces, and annotations in a running Java application. java.lang.class is a final class so it cannot be inherited into other classes.
The primitive data types like byte, short, char, int, float, and boolean are also represented using these Class instances.
For example:
String str = "strings";
Class c = Integer.class;
In the above instance, we see that "strings" are stored as a string data type in the application but whereas Integer is stored as a class type in the program which is referenced by the variable of class type c. Using c we can create an object for the Integer class. We can get the details about that class dynamically even during Runtime.
Class present in Java is also used to represent an instance of the array in Java.
Three Ways to Create a Class object in Java
As we have discussed earlier, the Class class has a private constructor so it cannot be called or created outside the class (globally), so to avoid that we mainly have three ways of instantiating the object of the Class class in Java. They are discussed in this section:
- Using Class.forName(): This internally uses Class loader support to load the class.
For example:
Class c = Class.forName("java.util.Date")
This Class.forName method loads java.util.Date class and returns the object of java.lang.Class having loaded class name as the data of the object. Here c is the reference variable pointing to the object of java.lang.Class. The below image clearly explains the memory diagram of the instantiated class:
2. Using getClass() method of java.lang.Object class. This method can be used on any class because it is invokable on any class object.
For example:
button b = new button();
Class c = b.getClass();
The button is the data of the object which is the object of java.lang.Class. C is the reference variable for the object of the java.lang.Class. The below image clearly explains the memory diagram of the instantiated class:
3. Using Myclass.class writing .class after a class name, helps in referencing the Class object which represents the given class. It is frequently used with primitive and also non-primitive data types. This method is used only when the name of the class is known before creating the object. The class name for which the Class object is to be created is determined at compile time.
For example:
Solution s = new Solution()
Class c = Solution.class()
This statement does not give any error because it is instantiated with the Solution class.
Methods of Class Class in Java
This section explains a few methods present in Class class in Java with the method name, syntax, and description:
S.No | Method | Syntax | Description |
---|---|---|---|
1. | asSubclass(Class < U> clazz) | public < U > Class<? extends U> asSubclass(Class < U > clazz) | This Class's object helps in casting it to a subclass that represents a specified class object. The parameter U is the type to cast this class object into and clazz is the class of the type to cast this class object to. |
2. | cast(Object obj) | public T[] cast(Object obj) | The cast() method of java.lang.Class is used to cast the specified object to the object of this class. |
3. | desiredAssertionStatus() | public boolean desiredAssertionStatus() | It is used to get the Assertion Status of this class, which would get assigned to the class when this method is invoked. |
4. | forName(String name, boolean initialize,ClassLoader loader) | public static Class <T > forName(String className, boolean initialize, ClassLoader classLoader) | This method is associated with the class or the interface with the given classname, using the given classloader. |
5. | getAnnotatedInterfaces() | public Annotation[] getAnnotatedInterfaces() | This method is used to get the super interface type annotations present in this class. |
6. | getAnnotatedSuperclass() | public AnnotatedType getAnnotatedSuperclass() | This method returns an object representing the superclass. |
7. | getAnnotation(Class < A> annotationClass) | public T getAnnotation(Class< T> annotationClass) | This method of java.lang.Class returns the specified object of the annotation class. This method accepts a parameter annotationClass which is the type of annotation to get. |
8. | getAnnotations() | public Annotation[] getAnnotations() | The method returns an array of annotations present in the given class. This method does not accept any parameters. |
9. | getAnnotationsByType(Class<A> annotationClass) | public <T extends Annotation> T[] getAnnotationsByType (Class< T> annotationClass) | This method of java.lang.Class returns annotations that are associated with this element. |
10. | getCanonicalName() | public String getCanonicalName() | This method of java.lang.Class returns the canonical name of the class. This method does not have any parameters. |
11. | getClasses() | public Class[] getClasses() | This method returns an array containing objects representing the interfaces and classes that are members of the class and are represented by this class Object. |
12. | getClassLoader() | public ClassLoader getClassLoader() | This method returns the class loader for this class. |
13. | getComponentType() | public Class getComponentType() | This method of java.lang.Class returns the class which represents the component type of an array if the element is one otherwise it returns null. |
14. | getConstructor(Class<?>...parameterTypes) | public Constructor<T> getConstructor(Class[] parameterType) throws NoSuchMethodException, SecurityException | This method returns the constructor object which reflects the specified constructor represented by this present class object. The parameter parameterType, is the array of parameter types that are for the specified constructor. |
15. | getConstructors() | public Constructor[] getConstructors() | This method returns an array of constructors which are represented by this class object. |
16. | getDeclaredAnnotation(Class < A> annClass) | public T getDeclaredAnnotation(Class< T> annClass) | This method returns this present element’s annotation if it is present, else this method returns null. |
17. | getDeclaredAnnotations() | public Annotation[] getDeclaredAnnotations() | This method of java.lang.Class returns annotations that are present on this element. |
18. | getDeclaredAnnotationsByType(Class < A> annClass) | public A[] getDeclaredAnnotationsByType(Class < T> annClass) | The parameter present in this method is annClass which is the type of the declared annotation to be required and obtained. |
19. | getDeclaredClasses() | public Class[] getDeclaredClasses() throws SecurityException | This method returns an array of Class objects which reflects all the interfaces and classes that are represented by this present class object. |
20. | isAnonymousClass() | public boolean isAnonymousClass() | This method does not accept any parameters. This method returns a boolean value True if this class is an anonymous class else False. |
21. | isArray() | public boolean isArray() | This method returns a True if the Class is the Array class else it returns False. |
22. | isAssignableFrom(Class<?>cls) | public boolean isAssignableFrom(Class<T> class) | This method checks whether the interface or class represented by the class object is either the same or is a superclass or super interface represented by this given class parameter, returns True if it is else False. |
23. | isEnum() | public boolean isEnum() | This method returns true if the class is the Enum class, else returns False. |
24. | isInstance(Object obj) | public boolean isInstance(Object obj) | It checks whether the specified obj is compatible with the object represented by this class, if yes then it returns True else it returns False. |
25. | isInterface() | public boolean isInterface() | If the given class is interface then it returns True else it returns False. |
26. | isLocalClass() | public boolean isLocalClass() | It returns the boolean value True or False. This method returns True if this Class is the Local class, else returns false otherwise. |
27. | isMemberClass() | public boolean isMemberClass() | This method returns True if this Class is the Member class. Else it returns false otherwise. |
28. | isPrimitive() | public boolean isPrimitive() | This method returns True if this class represents a primitive class, else it returns False. |
29. | isSynthetic() | public boolean isSynthetic() | If the method is a synthetic construct then the function returns true otherwise it will return false. |
30. | newInstance() | public T newInstance() throws InstantiationException, IllegalAccessException | This method creates a new instance of the class represented by this current class object. |
31. | toGenericString() | public String toGenericString() | This method returns a string that gives the details of this current method, which includes details of the type parameters, modifiers, and type of arguments of this method. |
32. | toString() | toString() | This method returns the String representation of the present class's object. In Java, if we print any object, the Java compiler internally invokes the toString() method on the object. |
33. | getMethods() | public Method[] getMethods() | This method returns the methods of this class in the form of an array of Method objects. |
34. | getModifiers() | public int getModifiers() | This method returns the modifiers for the method represented by this method object. These modifiers are encoded as the integer and an integer value is returned. |
35. | getName() | public String getName() | This method returns the name of the entity represented by this class object in the form of the string data type. |
36. | getPackage() | public Package getPackage() | This method returns the package of this class. |
37. | getResource(String name) | public URL getResource(String name) | The parameter name represents the name of the desired resource. This method returns the URL of the object of this class. |
38. | getResourceAsStream(String Name) | public InputStream getResourceAsStream(String Name) | This method has a parameter Name which represents the resource to get. This method returns the specified resource of this class in the form of the InputStream objects. |
39. | getSigners() | public Object[] getSigners() | This method returns the signers of the object of this class. |
40. | getSimpleName() | public String getSimpleName() | This method returns the name of the class in form of a String, if this class is anonymous it returns an empty string. |
41. | getSuperClass() | public Class<T> getSuperclass() | This method returns the superclass of this present class. |
42. | getTypeParameters() | public TypeVariable<Method>[] getTypeParameters() | This method returns an array of TypeVariable objects which are declared by the generic declaration of this object's method. |
43. | isAnnotation() | public boolean isAnnotation() | This method returns a boolean value True or False. It returns True if this Class is of the Annotation type, else it returns False. |
44. | isAnnotationPresent(Class<? extendsAnnotation> annotationClass) | isAnnotationPresent(Class<? extendsAnnotation> annClass) | The parameter annClass represents the type of the annotation to be obtained. It checks if an annotation of the annClass is same as the annotation type is present in this particular class. |
45. | getInterfaces() | public Class < T>[] getInterfaces() | This method does not accept any parameter. This method returns an array of interfaces that are directly implemented by this class. |
Examples of Class Class in Java
Below is the Java program which helps in practical understanding of the above-mentioned methods present in Class class present java.lang package:
Output:
The second example of Java implementation to mention all the different methods mentioned above is:
Output:
Conclusion
- This article explained the Class class from the lang package in Java.
- Class class in Java has different functions which are popularly used in Application Interface programs, and App development using Java.
- Different methods present in this Class class are discussed in the above article.
- The instance of the Class class present in Java represents classes and interfaces in a running Java application.
- Differenet methods like isEnum(), isArray(), getMethod(), etc are useful during Java application programs.