Class Not Found Exception in Java
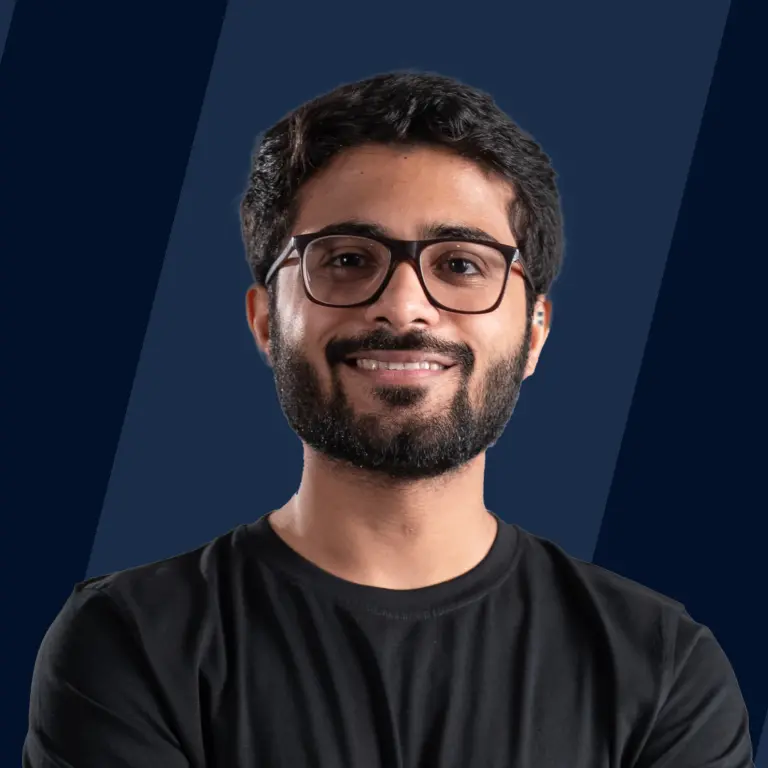
Overview
The java.lang.ClassNotFoundException is a checked exception in Java that occurs when the Java Virtual Machine (JVM) attempts to load a specific class, but cannot locate the class in the classpath.
ClassNotFoundException is a checked exception, hence it must be explicitly handled in methods that can throw it, either with a try-catch block or by using the throw clause.
What Causes ClassNotFoundException in Java?
ClassNotFoundException in Java is a checked exception that occurs when the Java Virtual Machine (JVM) has traversed the entire classpath and cannot find it.
Since we are indirectly loading the class using Classloader, the ClassNotFoundException always occurs at runtime. The Java compiler has no way of knowing whether or not the class will be present in the classpath at runtime.
ClassNotFoundException in Java occurs when an application tries to load a class using:
- forName method in Class class.
- findSystemClass method in ClassLoader class.
- loadClass method in ClassLoader class.
ClassNotFoundException is a checked exception that extends the ReflectiveOperationException, therefore ClassNotFoundException should be handled with a try-catch block or by using the throw clause.
Example of Class Not Found Exception in Java
Let's have an example for the class not found error in Java:
We will try to connect to MySQL using the Java Database Connectivity (JDBC) driver. We will load the driver using the forName method in Class class. Since the driver’s JAR file is not present in the classpath therefore this program will lead to ClassNotFoundException.
We'll use the Java Database Connectivity (JDBC) driver to try to connect to MySQL. The forName method in the Class class will be used to load the driver. Because the driver's JAR file is not in the classpath, this program will throw a ClassNotFoundException.
To throw the exception we will use the throw clause.
Output:
As you can see above there's a ClassNotFoundException for the class com.mysql.jdbc.Driver.
Let's have an another example, this time we will try to load a wrong class using the loadClass method.
Output:
Since, there is no class with string name com.scaler.temporary hence this program will lead to ClassNotFoundException. Now let's learn how to solve this exception.
How to Solve java.lang.ClassNotFoundException in Java?
ClassNotFoundException in Java can be solved in the following ways:
- Firstly, check that the requested class's name is correct and the requested JAR file is present in the classpath. In our case, mysql-connector-java.jar is not present in the classpath.
- First, ensure that the requested class has the correct name and that the requested JAR file is in the classpath. In our case, the classpath does not contain mysql-connector-java.jar.
- If the requested JAR file is not present in the classpath we must explicitly add it in the classpath.
- If the requested JAR file is present, then the classpath is getting overridden and we must find the exact classpath.
Now to fix the ClassNotFoundException in the first example we must add the mysql-connector-java.jar in the application's classpath.
And for the second example the requested class's name is wrong, therefore we must change the class name.
Conclusion
- ClassNotFoundException in Java is a checked exception that occurs when the JVM attempts to load a class but can not locate the class in the classpath.
- ClassNotFoundException exception occurs when we try to load the class using the forName, findSystemClass, and loadClass methods.
- ClassNotFoundException can be solved by verifying the name of the requested class and the requested JAR file in the classpath. If the requested JAR is present then we must find the exact classpath.