Cloneable Interface in Java
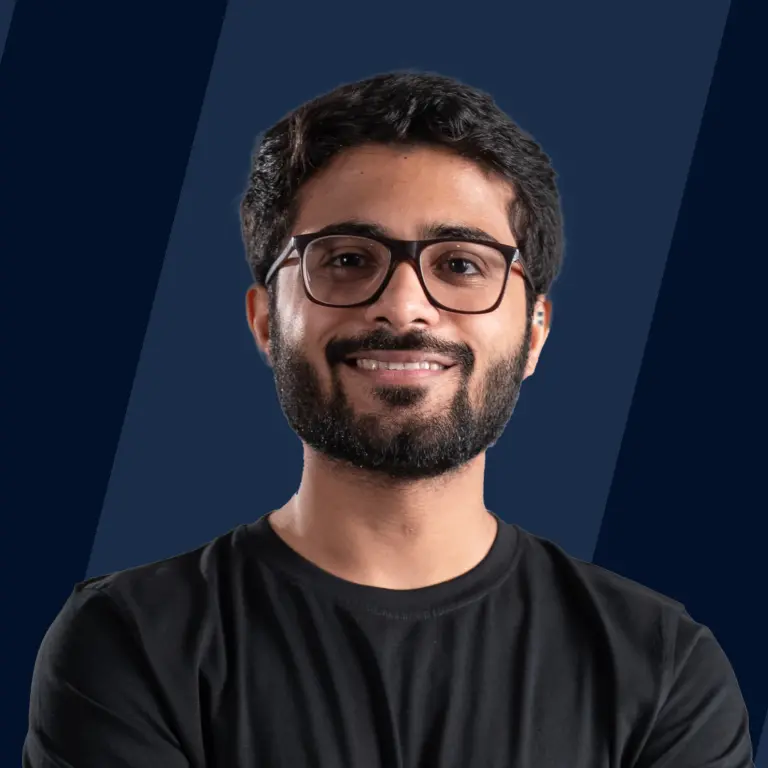
To create an exact copy of an object in Java, we use a Clonable interface. A Java class implements the Clonable interface to create a clone of the class object. The Cloneable interface exists in the java.lang package.
What is Cloneable Interface in Java?
Cloning is a technique for creating an exact copy of Java objects. By copy, we mean that all the data are replicated in the newly copied object.
A Cloneable interface in Java is used to clone an object in the Java class. The object class has a clone() method, which creates an exact copy of the object. The cloned object has a separate space in memory where the copy of the original object is stored. If a class doesn't implement the clonable interface and we try to clone the object, we get an exception called CloneNotSupportedException.
Declaration of Cloneable Interface in Java
Cloneable interface in Java is an interface that indicates that a class can be cloned. It is an empty interface that does not contain any methods to implement.
By convention, a class that implements the Cloneable interface is expected to override the Object.clone() method to provide a public clone method that returns a copy of the object.
Cloneable Interface is declared as :
Note that the clone() method is declared with the @Override annotation, indicating that it overrides the implementation provided by the Object class. The method also throws a CloneNotSupportedException if the class does not support cloning.
Examples of Cloneable Interface in Java
Example - 1 :
Let's see an example where the class doesn't implement the cloneable interface and tries to clone an object. It will result in CloneNotSupportedException.
Output :
In the above example, the class Department doesn't implement a cloneable interface but tries to clone the Department d1 object and create a copy d2 object of Department type. So it throws an error of Object cannot be converted to the department.
Example - 2 :
Now, we will see a proper example of a cloneable interface in which the clone() method is used to clone an object.
Output :
In the above example the Department class implements the cloneable interface and then tries to clone the department d1 object and create a new object d2 of type department using the clone() method of java. This doesn't throw any error as the class already implemented a cloneable interface so it can use the clone() method to clone an object.
Deep Copy Using clone() Method
Deep copy creates an exact copy of the original object in a separate memory space with all the fields similar to the original object. The Clone() method is used to do Deep copy. The Clone() method can also be used for a shallow copy, but the implementation varies for both. Deep copy creates a new memory for the copied object, so if the copied object field is changed, it does not affect the original object.
The code below is an example of a deep copy using the clone method.
Output :
In the above example, two classes are created. Marks class is a simple class with attributes x and y of type integer. The Marks2 class has attributes a and b of type integer, and the marks2 class also implements the cloneable interface.
Now, a copy of the marks2 class object is created (m2), and a new object of the marks class is also created, referenced by the cloned object of the marks2 class object. (m2.m = new Marks()).
Thus, when a value is assigned to the attributes of these cloned objects, they reference a separate memory space, and so the value of the original attribute remains the same even after cloning. This is known as deep cloning.
Conclusion
- Cloneable interface creates an exact copy of the object in java.
- Java class should implement the cloneable interface to use the clone() method to clone an object.
- Cloneable interface in java exists in java.lang package.
- The CloneNotSupportedException exception occurs when the class doesn't implement the cloneable interface. -A deep copy of an object creates a new object, that is an exact copy of the original object but has new memory space.