C++ <cmath>
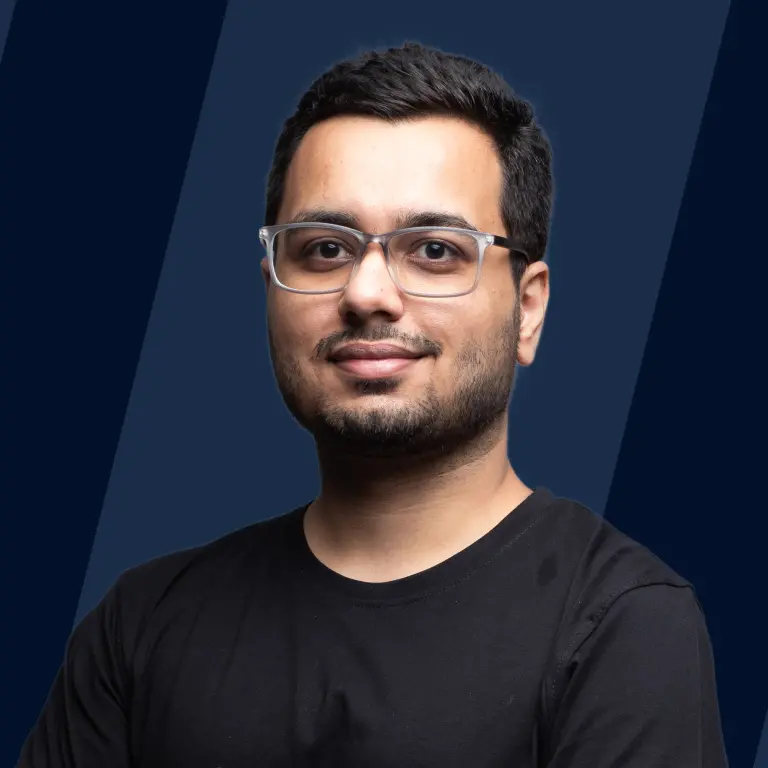
While programming much time we do mathematical calculations which require the use of many mathematical operations like evaluating square root, finding the exponent of a number, etc, so instead of writing these functions on our own, C++ provides a huge library (cmath c++ library) of these pre-defined functions which we can directly use to simplify our program.
What is C++ <cmath>?
<cmath> is a header file which consists of many pre-defined mathematical functions which can be directly used to simplify our code and also reduces our time to code. To include the cmath c++ header file in your code write #include <cmath>.
Commonly used Types of C++ <cmath>
Macros
A macro is a section of code that a program replaces with its value. The #define directive defines a macro. When the compiler comes across a macro name, it substitutes the name with the macro definition.
Example:
- INFINITY: evaluates to a value that will overflow a float.
- NAN: evaluates to float NAN(not a number).
Functions
Basic Operations
Basic operations include operations like finding max, min of two numbers or finding the absolute value of a number, etc.
Example:
- abs(num): returns absolute value of num.
- min(a , b): returns minimum of a and b.
- max(a , b): returns maximum of a and b.
Exponential Functions
It includes functions such as finding the logarithm of a number or finding exponential(e) raise to a given number, etc.
Example:
- exp(num): returns value of e^num.
- log(num): returns natural log (base e) of num.
Power Functions
It includes functions such as finding the exponent of another number raised to a number, finding the square root, cube root of a number, etc.
Example:
- pow(x , y): returns x^y.
- sqrt(num): returns square root of num.
- cbrt(num): returns cube root of num.
Trigonometric Functions
It includes functions for finding trigonometric values of a number.
Example:
- sin(num): returns sine of num.
- cos(num): returns cosine of num.
- tan(num): returns tangent of num.
Nearest Integer Floating-Point Operations
It includes functions to find the nearest integer to a given floating-point number.
Example:
- ceil(num): returns num after rounded up to the nearest integer.
- floor(num): returns num after rounded down to the nearest integer.
- trunc(num): returns num after truncating its decimal value.
Floating-Point Manipulation Functions
It includes functions that manipulate floating point numbers in some way.
Example:
- ldexp(x , y): returns x*(2^y).
- logb(num): Using FLT RADIX as the base for the logarithm, the C++ logb() function returns the logarithm of |num|. Logb() is similar to log2() for positive numbers since FLT RADIX is typically 2.
Classification and Comparison
It includes functions that classify a number or compare two numbers.
Example:
- isnan(num): checks if a number is nan (not a number) or not.
- isgreater(num1, num2): checks if num1 is greater than num2 or not.
Less Commonly used Functions of C++ <cmath>
Linear Interpolation
Linear interpolation creates new values based on an existing set of values.
lerp(a , b , t): returns a + t * (b - a).
Hyperbolic Functions
Hyperbolic functions in mathematics are analogues of regular trigonometric functions that are defined using the hyperbola rather than the circle. The points (cosh t, sinh t) form the right half of the unit hyperbola, just as the points (cos t, sin t) make a circle with a unit radius.
Example:
- sinh(x): computes hyperbolic sine.
- cosh(x): computes hyperbolic cosine.
- asinh(x): computes inverse hyperbolic sine.
Error and Gamma Functions
-
erf(arg): computes error of arg i.e it returns the value of the below function:
-
.
-
gamma(arg): computes gamma function of arg i.e it returns the value of the below function:
-
Mathematical special functions
-
beta(x , y): computes a beta function of x and y i.e it returns the value of the below function:
-
expint(x): computes exponential integral of x i.e it returns the value of the below function:
-
hermite(n , x): Computes the Hermite polynomials of the degree n and argument x i.e it returns the value of the below function:
Examples
- Program for using std::max and std::min
Output:
Explanation:
First, we declare and initialize two integer variables num1 and num2, then we use in-built min and max functions to compute the minimum and maximum of the two numbers respectively.
- Program for using std::abs
Output:
Explanation:
First, we declare and initialize an integer variable num1, then we use the in-built abs function to calculate the absolute value of num1.
Other Math Functions
The cmath c++ header file has additional functions like sqrt (square root), round (rounds a number), and log (natural logarithm)
Example:
Output:
Conclusion
- cmath c++ is a header file which consists of many pre-defined mathematical functions.
- To include the cmath header file in your code write #include <cmath>.
- Some commonly used Types of cmath c++ include abs, min and max.