Comparable Interface in Java with Examples
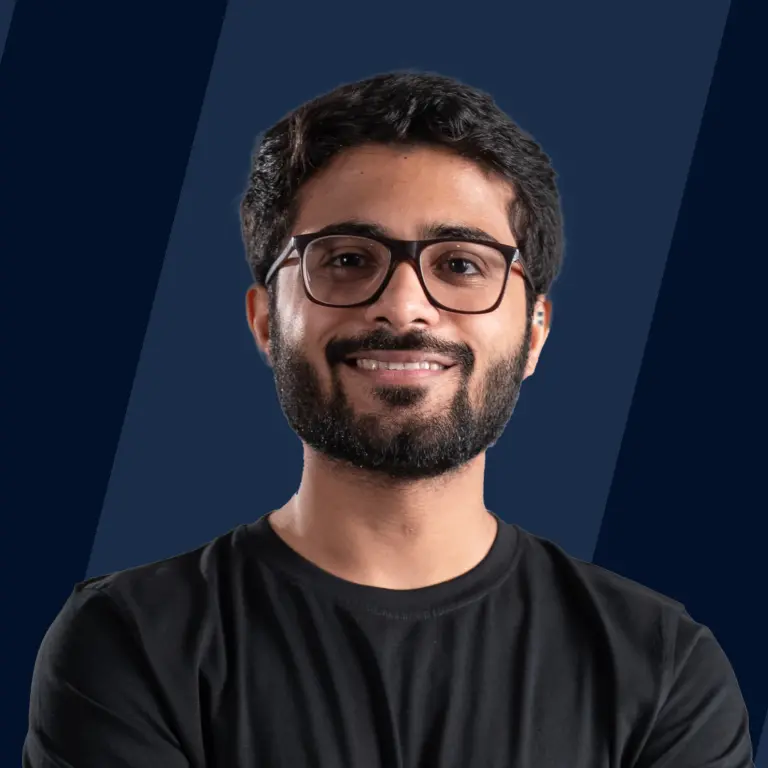
Overview
The Comparable interface compares an object of the same class with an instance of that class. The Java Comparable interface assesses the user-defined class's objects. This interface has only one method, compareTo(Object) and may be found in the java.lang package. It simply offers one sorting sequence, allowing you to arrange the items according to a single data member. It could be your name, roll number, age, or anything else.
What is a Comparable Interface in Java?
The Comparable interface provides data ordering for objects of the user-defined class and compares an object of the same class with an instance of that class. The class has to implement the java.lang to compare its instance; a comparable interface provides the compareTo method that takes a parameter of the object of that class.
Example
Given is an array of pairs that each has two fields of type integer and string. If two strings are identical, sort the array based on their numeric value and then in ascending Lexicographical order.
Output:
Explanation:
The Java code defines a class CustomPair, implementing the Comparable interface to enable custom sorting. Each CustomPair consists of a string key and an integer value. The code creates an array of CustomPair objects, populates it with fruit names and quantities, and then sorts the array first by keys and, if keys are equal, by values. Finally, it prints the sorted pairs to the console using a custom print method. This code demonstrates creating custom sorting logic for data pairs based on both keys and values.
The CompareTo Method
public int compareTo(Object obj): The current object and the provided object are compared using this method. It follows
- If the current object exceeds the given object, it is a positive integer.
- If the current object is smaller than the target object, a negative integer.
- 0 if the present item matches the requested object exactly.
We can arrange each element of:
- String objects
- Wrapper class objects
- User-defined class objects
How to Use Comparable Interface in Java?
Different ways to use Java Comparable Interface:
- We will implement the Comparable interface from the java.lang Package in the Pair class in this method.
- The compareTo method, which determines the order of the components, is part of the Comparable interface.
- The Pair class's compareTo method should be overridden.
- Make an array of Pairs and fill it with data.
- To sort the array, use the Arrays.sort() function.
Examples of Comparable Interface in Java Let's look at the Comparable interface's age-based list element sorting example.
An array of pairs is given, each containing two strings for the first and last names. You must order the array based on the first name in ascending Lexicographical order, and if two strings are identical, call it using their last name.
Output:
Explanation: This Java program demonstrates sorting a custom Person class using the Comparable interface. Let's break down the code step by step to understand what it does:
- Person class: This class represents a person with two attributes, firstName and lastName. It also implements the Comparable
interface, which means instances of this class can be compared to each other for sorting purposes. - String firstName: Represents the first name of the person.
- String lastName: Represents the last name of the person.
- compareTo method: This method is required to implement the Comparable interface. It defines the logic for comparing two Person objects for sorting. The method first compares the firstName of the current object with the firstName of the other object. If they are not equal, it returns the result of comparing the two first names. If they are equal, it compares the lastName of the current object with the lastName of the other object.
- Main class: This class contains the main method, where the program execution starts.
Inside the main method:
- An array of Person objects named people is created to hold information about different individuals.
- Five instances of the Person class are created and assigned to the people array, each with a first name and a last name.
- The Arrays.sort method is used to sort the array of Person objects. The sorting is based on the natural ordering defined by the compareTo method in the Person class.
- The sorted array is then printed using the printPeople method.
- printPeople method: This method takes an array of Person objects and prints each person's information.
Overall, the code aims to demonstrate how to define a custom class, implement the Comparable interface to establish a natural sorting order, and use the Arrays.sort method to sort an array of custom objects based on the implemented comparison logic. In this case, the Person objects are sorted first by their first and last names. The sorted List of people is then printed to the console.
Reverse order Let's look at the same Comparable interface example that reverses the order of the list elements.
Output
Explanation In this example, I've overridden the compareTo method in the Person class to reverse the comparison logic for both the lastName and firstName attributes. This will sort the Person objects in reverse order based on the last name and then the first name. When you run the code, you will see the output with the Person objects sorted in reverse order.
Collections Class
The Collections class provides static methods for sorting collection elements. We can use TreeSet or TreeMap if a collection's elements are of the Set or Map type. However, we are unable to sort List's parts. The Collections class provides methods for sorting elements of List type elements.
Methods
public void sort(List list) : It orders the List's parts. The Comparable type must be used for list elements.
Note that the Comparable interface is, by default, implemented by the String class and Wrapper classes. As a result, by default, the objects of string or wrapper classes stored in a list, set, or map will be Comparable.
FAQs
Q. What is the purpose of the Comparable interface in Java?
A. The Comparable interface defines a natural ordering for objects of a class. By implementing the Comparable interface, a class can provide a method to compare its instances, enabling them to be sorted and ordered.
Q. How do you implement the Comparable interface in a class?
A. To implement the Comparable interface, a class must provide an implementation of the compareTo method. This method takes another object of the same class as a parameter. It returns a negative integer, zero, or positive integer depending on whether the current object is less than, equal to, or greater than the provided object.
Q. When is the compareTo method used?
A. The compareTo method is used when objects need to be compared for ordering, such as sorting algorithms like Arrays.sort() or Collections.sort(). It defines the logic for comparing two objects to determine their relative positions in a sorted sequence.
Q. What is the contract for the compareTo method?
A. The compareTo method should follow these guidelines:
- It should return a negative integer if the current object is "less than" the other object.
- It should return zero if the current object is "equal to" the other object.
- It should return a positive integer if the current object is "greater than" the other object.
- The comparison logic should be consistent with the equals() method, meaning that if a.compareTo(b) == 0, then a.equals(b) should return true.
Q. How can I use the Comparable interface to sort custom objects?
A. To sort custom objects, implement the Comparable interface in the class of the objects you want to sort. Override the compareTo method to define the comparison logic based on the attributes you want to sort by. Once implemented, you can use sorting methods like Arrays.sort() or Collections.sort() to sort arrays or collections of your custom objects.
Q. Can I sort objects in reverse order using the Comparable interface?
A. Yes, you can achieve reverse sorting by simply reversing the comparison logic within the compareTo method. If the original logic returns a positive integer for "greater than," switch it to return a negative integer, and vice versa.
Q. Can a class implement multiple comparison logics using the Comparable interface?
A. No, the Comparable interface is meant to define a single natural ordering for a class. If you need to support multiple comparison logics, consider using the Comparator interface instead.
Q. What's the difference between Comparable and Comparator?
A. The Comparable interface is implemented by the class of the objects being compared to define a natural ordering. On the other hand, the Comparator interface allows you to define separate comparison logic in a separate class without modifying the original class. This is useful when you need to support multiple sorting orders or when sorting classes that you can't modify directly.
Conclusion
- We learned about the Comparable interface in this course.
- With its simple interface implementation, this interface assists in forcing a natural order on things.
- We also learned how to sort a list of strings, an array of strings, a list of integers, and an array of integers.