How To Compare Two Dates in JavaScript?
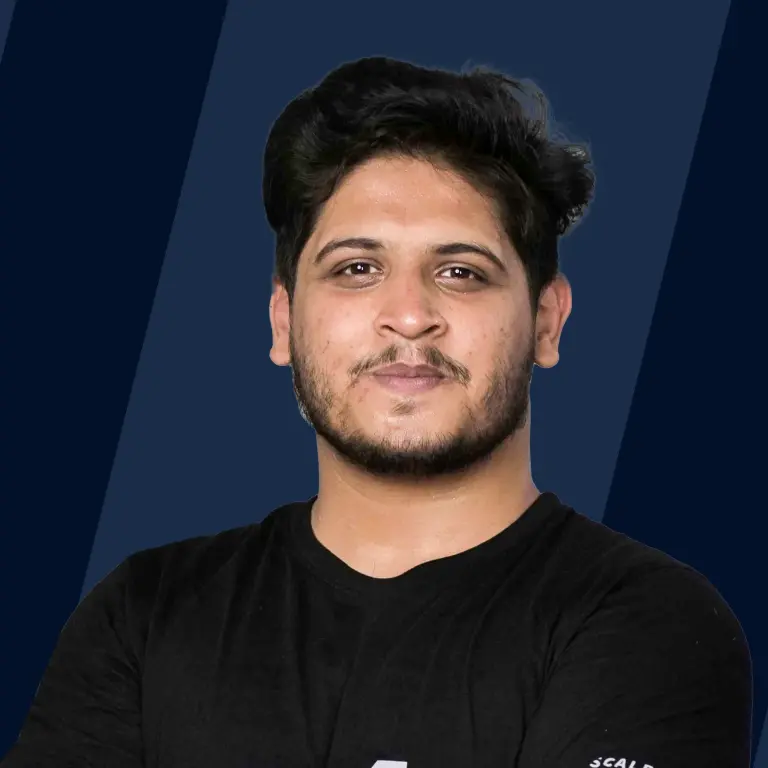
There are some predefined ways to compare dates in javascript. To check the date, the first step is to create a date object using the Date() constructor. Then, we can check them using various ways- either using the comparison operators > or <, or by using the in-built getTime() function, which can directly check the two dates.
How to Perform Date Comparison With the Date Object in JavaScript
The JavaScript Date object is used to work with date and time. It allows us to create a new date with the specified date and time. The date can be in any format like YYYY, MM, DD or YYYY-MM-DDTHH:MM: SSZ, etc.
Syntax-
Using the comparison operators > or <, we can compare two dates in JavaScript. We can also use the getTime() function to compare dates in JavaScript. In the next section, we will discuss the above methods.
The date can be compared with the other date using > or <. We will check which date is before.
Output-
How to Perform Equality Comparison With JavaScript
When comparing dates, we often use the getTime() method to ensure accuracy down to the millisecond. However, if we need to compare specific date components like day, month, or year, we rely on methods such as getDate(), getMonth(), and getFullYear().
Consider this example:
Output:
How to Perform Specific Date Comparisons
If we want to compare specific date values, like the day of the week, we can use the .getDay() date method. This method returns the day of the week as a number, where Sunday is 0, Monday is 1, and so on, up to Saturday is 6.
Output:
Examples
Basic Date Comparison in JavaScript
When comparing dates in JavaScript, the foundational approach involves utilizing the Date object alongside the getTime() method. This combination allows you to manipulate dates and times effectively by returning the number of milliseconds since the Unix Epoch, which serves as a reference point for date calculations.
Output:
Advantages and Potential Pitfalls
While this method is effective for basic comparisons, it may overlook nuances like time zones or diverse date formats. Let's dive into strategies to address these intricacies in the following section.
Handling Time Zones and Formats
In JavaScript, when you're comparing dates, it's essential to remember time zones and various date formats. You can handle this using methods like toISOString() and toLocaleDateString().
Output:
Dealing with Different Formats
The toLocaleDateString() method takes a Date object and returns a string representing the date portion, formatted according to the specified language and options. If no locale is provided, it defaults to the user's locale.
For instance, consider this example:
Output:
Using Moment.js for Date Comparison
Moment.js simplifies date handling in JavaScript by providing functions to compare dates easily. Whether you're working with past, present, or future dates, Moment.js makes it straightforward to compare and determine relationships between them.
Output:
Using date-fns for Date Comparison
date-fns is like a Swiss Army knife for working with dates in JavaScript. It offers a range of handy functions that make it easy to compare, manipulate, and format dates. Whether you need to check if one date is before another, add or subtract time intervals, or format a date in a specific way, date-fns has got you covered. It's a modern and reliable tool for anyone dealing with dates in their JavaScript projects.
Output:
JavaScript Date Methods
JavaScript provides several helpful methods for working with comparing dates. These methods allow you to manipulate, format, and extract information from date objects.
Method of Date Object | Description |
---|---|
getDate() | This method returns the day of the month from 1-31 for the given date according to the local time. |
getDay() | This method is used to return the day of the week from 0-6 for the given date according to the local time. |
getFullYear() | This method returns the year, which is a 4-digit number for the given date according to the local time. |
getHours() | This method is used to return the hour of the specified date from 0-23 for the given date according to the local time. |
getMilliseconds() | This method is used to return the millisecond from 0-999 for the given date according to the local time. |
getMinutes() | This method is used to return the minutes from 0-59 for the given date according to the local time. |
getMonth() | This method is used to return the month from 0-11 for the given date according to the local time. |
get seconds() | This method returns the second of the given date from 0-59 for the given date according to the local time. |
getTime() | This method returns the numeric value of the given date as the number of milliseconds that have elapsed since January 1, 1970, 00:00:00 UTC. |
getTimezoneOffset() | This method is used to return the time zone offset in minutes. |
getUTCDate() | This method returns the day of the month from 1-31 for the given date according to the universal time. |
getUTCDay() | This method returns the day of the week from 0-6 for the given date according to the universal time. |
getUTCFullYear() | This method returns the year, which is a 4-digit number for the given date according to the universal time. |
getUTCHours() | This method is used to return the hour from 0-23 for the given date according to the universal time. |
getUTCMilliseconds() | This method is used to return milliseconds from 0-999 for the given date according to the universal time. |
getUTCMinutes() | This method is used to return the minutes from 0-59 for the given date according to the universal time. |
getUTCMonth() | This method is used to return the month from 0-11 for the given date according to the universal time. |
getUTCSeconds() | This method returns the seconds of the given date from 0-59 for the given date according to the universal time. |
getYear() | This method is used to return the year but in 2-3 digits so use getFullYear() method. |
setDate() | This method is used to set the day of the month from 1-31 for the given date according to the local time. |
setFullYear() | This method is used to set the year, a 4-digit number for the given date according to the local time. |
setHours() | This method is used to set the hours of the specified date from 0-23 for the given date according to the local time. |
setMilliseconds() | This method is used to set the millisecond from 0-999 for the given date according to the local time. |
setMinutes() | This method is used to set the minutes from 0-59 for the given date according to the local time. |
setMonth() | This method sets the month from 0-11 for the given date according to the local time. |
setSeconds() | This method is used to set the seconds of the given date from 0-59 for the given date according to the local time. |
setTime() | This method is used to set the numeric value of the given date as the number of milliseconds that have elapsed since January 1, 1970, 00:00:00 UTC. |
setUTCDate() | This method sets the day of the month from 1 to 31 for the given date according to the universal time. |
setUTCFullYear() | This method is used to set the year, a 4-digit number for the given date according to the universal time. |
setUTCHours() | This method is used to set the hour from 0-23 for the given date according to the universal time. |
setUTCMilliseconds() | This method is used to set milliseconds from 0-999 for the given date according to the universal time. |
setUTCMinutes() | This method is used to set the minutes from 0-59 for the given date according to the universal time. |
setUTCMonth() | This method sets the month from 0-11 for the given date according to the universal time. |
setUTCSeconds() | This method is used to set the seconds of the given date from **0-59 for **the given date according to the universal time. |
setYear() | This method is used to set the year but in 2-3 digits, so use the setFullYear() method. |
toDateString() | It is used to return the "date" portion in the form of the string which is human-readable. |
The Unix Epoch and Time Zones
JavaScript's Date object operates within the framework of the Unix Epoch, which marks the beginning of time as 00:00:00 UTC on January 1, 1970. This standardizes time computations across platforms.
Time zones define regions with the same standard time, and while JavaScript defaults to the local time zone, it can also handle UTC or any other time zone. Understanding these basics about the Date object and time zones will enhance your ability to compare dates effectively.
The Relevance and Applications of Date Comparison
JavaScript's date comparison capabilities extend beyond simply determining which date comes before or after another. Instead, it empowers developers to handle and manipulate time effectively, opening up a world of possibilities across various applications.
Scheduling Tasks
For instance, JavaScript's date comparison capabilities allow developers to automate processes based on specific dates and times when scheduling tasks. This feature is invaluable for ensuring the timely execution of critical operations.
Creating Calendars
Moreover, the creation of calendars heavily relies on date comparison. By accurately comparing dates, developers can determine essential calendar details such as the number of days in a month or the day of the week a month begins on. This functionality is crucial for building intuitive and user-friendly calendar applications.
Exploring Related Concepts
Furthermore, beyond simple date comparison, JavaScript also offers date manipulation and formatting functionalities. These capabilities enable developers to perform advanced operations, such as adding or subtracting days, months, or years from a date and displaying dates in various formats tailored to user preferences.
Conclusion
- JavaScript provides the Date object for working with dates and times, allowing us to create specific dates and times.
- Date objects in JavaScript offer methods such as getFullYear(), getMonth(), and getTime() to manipulate and format dates according to our requirements.
- Comparison operators like > or < can be used to compare dates in JavaScript, while the getTime() method directly compares two dates.
- The Date object provides versatility in handling date and time operations, making it a powerful tool for working with temporal data in JavaScript.