Compare Two Dates in Java
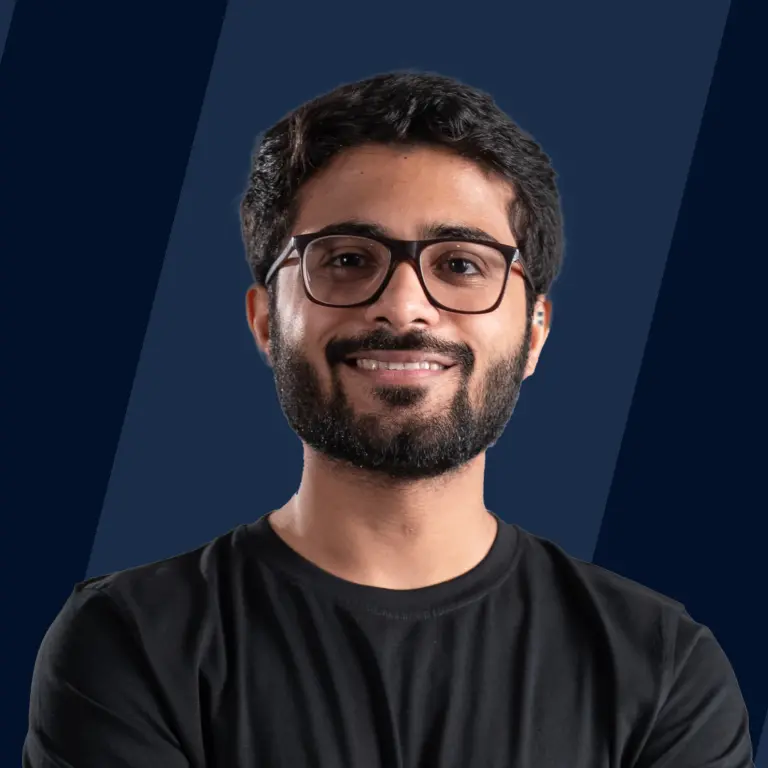
In Java, comparing dates requires specialized methods like compareTo(), before(), after(), and equals() due to their unique nature compared to numeric values. The Date class from the java.util package encapsulates specific moments with millisecond accuracy, implementing key interfaces like Serializable, Cloneable, and Comparable. This article elucidates how Java facilitates date comparison through these intrinsic methods, streamlining application date and time operations.
Example: Compare two dates Using Date Class in Java
Date class in Java has many useful functions like before(), after() and equals(). These functions help us to compare two dates.
To use these functions, we need to import the following:
- Date from the utility package
- SimpleDateFormat from the text package
- ParseException from the text package
Let's discuss these functions in detail:
after():
Syntax:
Return Type: Boolean
This function returns true if comes after strictly; otherwise, it returns false. Even if the dates are equal, it returns false.
before():
Syntax:
Return Type: Boolean
This function returns true if comes before ; otherwise, it returns false. Even if the dates are equal, it returns false.
equals():
Syntax:
Return Type: Boolean
This function returns true strictly if and are equal, else it returns false. The equals() method of Date class checks for equality by comparing the number of milliseconds elapsed since 1st January 1970, 00:00:00 GTM. It does it with the help of the getTime() function. getTime() function returns the number of milliseconds in long data type.
We will use the SimpleDateFormat class object to store dates. This will help us define the format of our date.
Syntax:
The format string in the parameter of the SimpleDateFormat object should have the pattern in which the date string should be parsed. This table has the syntax for this String. We use these letters in the given table to define our pattern string. See the example after the table for a clear understanding.
Letter | Representation | Type | Examples |
---|---|---|---|
G | Era designation | Text | AD |
y | Year | Year | 2022 or 22 |
M | Month of year | Month | March; Mar; 03 |
w | Week number of Year | Number | 12 |
W | Week number of Month | Number | 3 |
D | Day of year | Number | 074 |
d | Day of month | Number | 15 |
E | Name of the Day | Text | Tuesday; Tue |
a | AM/PM | Text | PM |
H | Hours of the day (0-23) | Number | 19 |
k | Hours of the day (1-24) | Number | 20 |
K | Hours in AM/PM (0-11) | Number | 07 |
h | Hours in AM/PM (1-12) | Number | 08 |
m | Minutes | Number | 37 |
s | Seconds | Number | 45 |
S | Milliseconds | Number | 456 |
z | Time zone | General time zone | Indian Standard Time or IST or GMT+05.30 |
Z | Time zone | RFC 822 time zone | +0530 |
X | Time zone | ISO 8601 time zone | +0530; +05:30 |
Example of the string:
Syntax for parsing:
For using Date Class in Java, we will require these packages:
- Date from the utility package
- SimpleDateFormat from the text package
- ParseException from the text package
Code:
Output:
Explanation:
Here, we are creating a SimpleDateFormat object, which will help us convert the given dates into a string type of "Date" type. We will convert using the parse function of the SimpleDateFormat class. Then we use equals(), after(), and before() functions to compare the given dates.
We can also change the format of the date using SimpleDateFormat.
Example:
Let's see what we should code to change the date format from dd/MM/yyyy to dd MMM yyyy.
Code:
Output:
Explanation:
Here we are creating Simple Date Format objects, 1 to parse the given date from String to Date type and the other to format it in the new format.
Example: Java Compare two dates Using Date.compareTo()
We can use the compareTo() function from the Date class to compare the two dates.
compareTo() function returns:
- 0 if both dates are equal
- 1 if date1 comes after date2
- -1 if date1 comes before date2
Syntax:
Return Type: Integer
For using Date.compareTo(), we need to import:
- Date from the utility package
- SimpleDateFormat from the text package
- ParseException from the text package
Code:
Output:
Explanation:
Here we are using SimpleDateFormat object to parse the given dates from String type to Date type, and then using compareTo() function of Date class to compare the dates.
Example: Java Compare two dates Using Calendar Class
Similar to the Date class, we have Calendar Class in Java, and it has functions like before(), after(), and equals() as well. Then you might think what is the difference between Calendar and Date Class?
The Date class has a fixed instance of time and calculates the difference between the dates with respect to that instant, while the Calendar class directly calculates the difference between the two dates.
The explanations of before(), after() and equals()can be refered from above.
We need to initialize two variables using the getInstance() method for the Calendar class in Java. getInstance() returns the current date and time using Locale and Timezone. We will use the setTime() function to set the dates. setTime() is used to set the required date. Calendar class compares time in milliseconds.
Syntax:
For using the Calendar class, we need to import:
- Calendar from the utility package.
- SimpleDateFormat from the text package
- ParseException from the text package
Code:
Output:
Explanation:
Here, we are using the SimpleDateFormat object to parse the dates. Then, we print the date using the parse() and format() methods. We have initialized 2 Calendar variables using the getInstance() function. getInstance () initializes them with the ** current time. ** Then, we have used the ** setTime () ** function to store the given dates. At the end, we compare the dates using the equals(), after(), and before() methods of the Calendar class.
Example: Java Compares two dates Using LocalDate Class
LocalDate Class was introduced in Java 8. It is a part of the time package in Java. It is used to represent Local dates without time and zone information. Therefore it is used when we do not have to specify any time or zone. It is perfect for storing dates of important events (for example, class attendance) or comparing two dates. LocalDate instances are immutable,, i.e., can not be changed once they are created.
We have methods like isAfter(), isBefore(), isEqual() and compareTo() in LocalDate Class. isAfter(), isBefore() and isEqual() are very similar to after(), before() and equals() methods of Calendar and Date class.
The default format of the date is yyyy-MM-dd. To change the format, we can use the DateTimeFormatter() class and parse the function of the LocalDate class.
Syntax:
We will have to import these packages:
- LocalDate from the time package
- DateTimeFormatter from the time.format package
- ParseException from the text package
Code:
Output:
Explanation:
Here, we are using the DateTimeFormatter object to parse the given dates from String type to LocalDate type. Then we use the isEqual(), isAfter(), and isBefore() functions to compare the given dates.
We can also use the compareTo() function of the LocalDate class to compare the Dates.
Syntax:
Return Type: Integer compareTo() function returns:
- 0 if both dates are equal
- 1 if date1 comes after date2
- -1 if date1 comes before date2
Code:
Output:
Explanation:
Here we are using DateTimeFormatter object to parse the given dates from String type to LocalDate type, and then using compareTo() function of LocalDate class to compare the dates.
Conclusion
- To compare Dates in Java, we can use the Date, Calendar, or LocalDate class.
- Real-life scenarios of comparison of Dates are storing attendance and comparing age.
- Date class compares every date with 1st January 1970, 00:00:00 GTM.
- Calendar and LocalDate are preferred compared to Date class because they do not have a fixed date and time for comparing the new dates. (Date class gets buggy around leap year as well)