Compare Two Strings in JavaScript
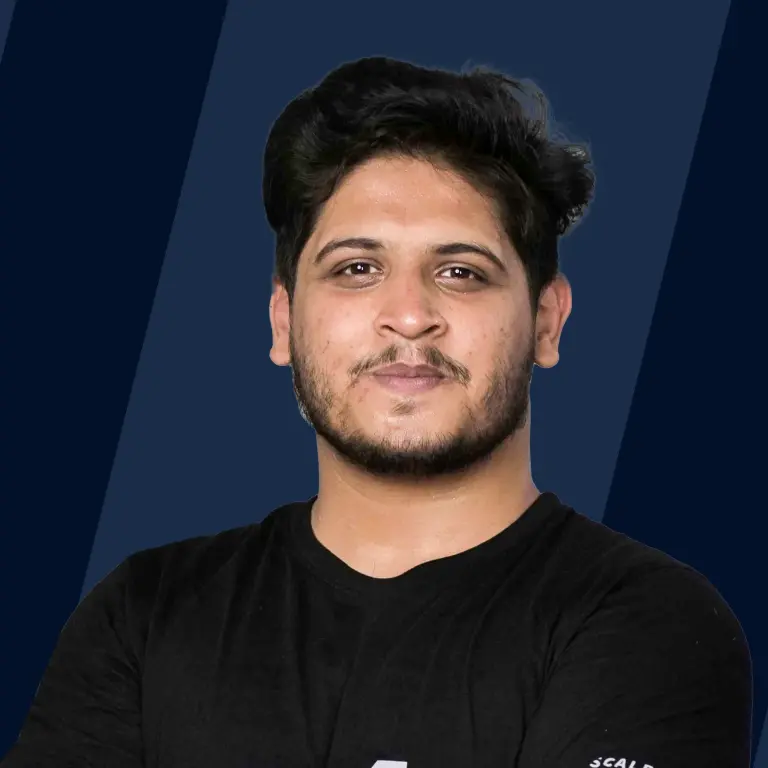
In JavaScript, comparing two strings is essential for tasks like login authentication. This involves verifying if a user-entered username matches the one in the database. We can compare strings using methods like toUpperCase(), localeCompare(), and RegEx. While JavaScript's strict equality operator is a straightforward choice, nuances like string length and alphabetical order can also be considered. Understanding these methods is key, as JavaScript, unlike humans, lacks comprehension skills and relies on literal string comparison to differentiate phrases like 'Let’s eat grandma' and 'Let’s eat, grandma'.
Case-insensitive String Comparison
Imagine we are taking name inputs from the users and checking if that name is present in our database. In such cases, the two strings need not be strictly equal, i.e., the strings can have a different case. Such situations are known as case-insensitive string comparison situations.
When comparing strings, case insensitivity refers to not taking into account uppercase and lowercase letters. The method toLowerCase() and toUpperCase() in javascript is used to determine case insensitive comparison of two strings in javascript.
Example
Output:
Explanation of the example:
In the above example, when str1 and str2 are compared after using the toUpperCase method, the expression JAVASCRIPT == JAVASCRIPT would return true as they are the same strings after both being in upper case. Here, the equality operator (==) is used to check if both the strings are the same.
In the second case, when str1 and str3 are compared after using the toUpperCase method, the expression javascript == javascript would return true as they are the same strings after both being in lower case.
In the third case, when str1 and str4 are compared, the expression Javascript == Typescript would return false as Javascript and Typescript are different strings.
Comparing the Length of JavaScript Strings
Imagine we have created an online forum where we are taking reviews from users. Now there needs to be a word limit, and we are supposed to send the user-customized message-based if the user crosses the word limit. Now, in order to do this, we need to compare the two.
In order to compare two strings based on their lengths, we need to find the length of the two strings and compare them using the greater than or smaller than operator.
How to find the length of the string in javascript?
- The string.length property is used to find the length of a string in javascript. It returns a number, which is equal to the length of the given string.
For example:
Another way to find the length of the string is to find iteratively. We can run a loop over a string and count the number of iterations in it.
For example:
Now we can compare these strings according to their lengths. If the length of a string is more than the length of the other string it is being compared to, our condition will return true otherwise, our condition will return false.
Example
Output:
Explanation of the example:
In the above example, the length of str1, str2, and str3 are 10, 6, and 7, respectively.
In the first case, the expression s1.length > s2.length will return true, thus example(str1, str2) will return 'String a is greater than string b'.
Similarly, In the second case, the expression s1.length > s2.length will return false, thusexample(str2, str3) will return 'String b is greater than string a'.
Ready to dive into the world of web development? Join our JavaScript certification course and unlock the secrets of building interactive websites.
Check if a String Contains Another String
The includes method in javascript is used to check whether the given string is present inside another string.
Note: If a string is present inside another string in javascript, it is known as the substring of the string.
The following diagram depicts the working of the includes method in javascript:
Syntax
Here we are checking if str2 is present in str1.
Parameters
- str: A string that has to be checked if it is present inside another string.
Return value
- boolean: If returns true if str2 is present in str1 otherwise it returns false.
Exceptions
The includes method in javascript is case-sensitive thus, it would return false if the case of the substring does not match with the passed parameter.
Example
Output:
Explanation of the example:
In the above example, s1.includes(s3) would return true as 'Javascript' is present in s1, whereas s2.includes(s3) would return false as 'Javascript' is present in s2. Use this Free Online JavaScript Compiler to compile your code.
Examples
JavaScript String Comparison Using toUpperCase()
As discussed earlier, The toUpperCase() method is used to return the calling string value converted into the uppercase.
Output:
Explanation of the example:
In the above example, we have changed the case of the string1 and string2 to uppercase. Now we will use the strict equality operator to compare both strings and return the result.
JavaScript String Comparison Using RegEx
In Javascript, the regular expressions are the patterns that are used to match character combinations in the javascript strings.
Output:
Explanation of the example:
In the above example, we are using the RegEx along with the test() method to perform case insensitive string comparison.
In the RegEx pattern, "g" syntax denotes global, and "gi" syntax denotes case insensitive comparisons.
JavaScript String Comparison Using String localeCompare() method
The localeCompare() method returns a number indicating whether a reference string comes before, after, or is the same as the given string in sort order.
Output:
Explanation of the example: In the above case, the localCompare() method returns 0, so the value of the result becomes 0, hence the above output is printed.
Conclusion
- The inbuilt javascript methods can be used to compare two strings.
- For case-insensitive string comparison, toUpperCase and toLowerCase methods are used, which compare the value of the string using the equality operator after converting to uppercase and lowercase, respectively.
- The str.length attribute of the string is used to get the length of a string.
- The includes method in javascript is used to check whether the given string is present inside another string.
- We can use RegEx to compare the equality of two strings in javascript.
- The localeCompare() method is used to compare two strings. It will return a number that does indicate if the string passes as a parameter comes before, after, or is the same as the given string in sort order.