ConcurrentHashMap in Java
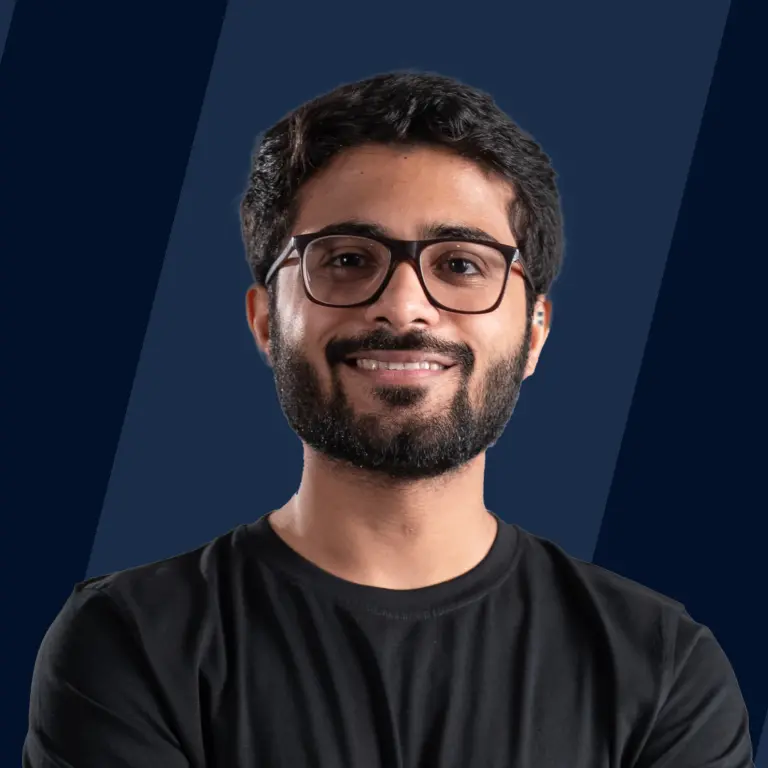
Overview
ConcurrentHashMap, in java.util.concurrent, improves performance by using a different locking strategy than HashTable or Synchronized HashMap. It's thread-safe, allowing multiple threads to work on a single object. The map can be initialized with various options, such as initial capacity, concurrency level, and load factor. The class provides methods for safe insertion and deletion of elements, as well as bulk operations like foreach(), search(), and reduce(). It implements the Serializable interface and ConcurrentMap.
Introduction to Java ConcurrentHashMap
The ConcurrentHashMap class, which is new in JDK 1.5, is a part of the java.util.concurrent package, which also implements the Serializable interface and ConcurrentMap. ConcurrentHashMap is an improvement on HashMap because it is well known that HashMap is not a good option when dealing with Threads in our application due to poor performance. Key points of ConcurrentHashMap:
- The Hashtable data structure, is a key component of ConcurrentHashMap
- Because the ConcurrentHashMap class is thread-safe, several threads can work together seamlessly on a single object.
- Unlike HashMap, which lacks the ConcurrentHashMap object, any number of threads may be active during a read operation.
- According to the concurrency level, the Object is divided into a number of segments in ConcurrentHashMap.
- ConcurrentHashMap's default concurrency level is 16.
- Any number of threads may execute retrieval operations on a ConcurrentHashMap at once, but in order to change an object, a thread must lock the specific segment in which it wishes to operate. Segment locking or bucket locking are two names for this kind of locking mechanism. Consequently, threads are able to handle 16 update actions concurrently.
- It is not feasible to insert null objects into ConcurrentHashMap as a key or value.
Declaration
ConcurrentHashMap is inherited from AbstractMap.That means that AbstractMap is the parent class and ConcurrentHashMap is the child class. All methods of AbstractMap can be used in ConcurrentHashMap.ConcurrentMap also implements ConcurrentMap and Serializable interfaces.
Hierarchy of ConcurrentHashMap
ConcurrentHashMap implements Serializable, ConcurrentMap<K,V>, Map<K,V> interfaces and extends AbstractMap<K,V> class.
Constructors of ConcurrentHashMap
Concurrency-Level: It is the number of threads that are simultaneously updating the map. The implementation tries to handle this many threads by performing internal scaling.
Load-Factor: It is a threshold that regulates resizing. When the average number of elements per bin exceeds this limit, resizing may be done. This means if the load factor of Concurrent HashMap is 0.50 mean if our Map is filled 50% then values will be moved to a new ConcurrentMap with double size.
Initial Capacity: Initial Capacity of Map. The map can be dynamically resized if more of the initial capacity is filled in the map. If the capacity of this map is 5. It means that it can store 5 entries.
1. ConcurrentHashMap():
Generates a new, empty map with the default initial concurrency level (16), load factor (0.75), and capacity (16).
Syntax
Example In this example, we are going to create a ConcurrentHashmap with the constructor that we just discussed above.
Code Explanation Above Code will create a ConurrentHashMap with the default initial concurrency level (16), load factor (0.75), and capacity (16).
2. ConcurrentHashMap(int initialCapacity):
Generates a new, empty map with the given initial capacity, the default load factor (0.75), and the concurrencyLevel (16).
Syntax
Example
In this example, we are going to create a ConcurrentHashmap with the constructor that we just discussed above
Output
Code Explanation
Above Code will create a ConcurrentHashMap with an initial capacity of 1. Note that 1 will not be the final capacity of ConcurrentHashMap. As in the above code we have created ConcurrentHashMap with initial capacity 1 but adding 2 elements is perfectly valid.
3. ConcurrentHashMap(int initialCapacity, float loadFactor):
The function generates a new, empty map with the given initial capacity, load factor, and concurrencyLevel (16).
Syntax
Example In this example, we are going to create a ConcurrentHashmap with the constructor that we just discussed above
Code Explanation
In Above code we have created a ConcurrentHashMap With initial capacity of size 1 and loadfactor 0.20.
4. ConcurrentHashMap(int initialCapacity, float loadFactor, int concurrencyLevel):
creates a new, empty map with the given capacity, load factor, and concurrency level that are supplied.
Syntax
Example In this example, we are going to create a ConcurrentHashmap with the constructor that we just discussed above
Code Explanation
In the Code Above we have created a ConcurrentHashMap with an initial capacity 1 load factor of 0.20 and concurrencyLevel 10.
5. ConcurrentHashMap(Map m):
Generate a new map with the same mappings as the specified map. Syntax
Example
In this example, we are going to create a ConcurrentHashmap with the constructor that we just discussed above. First, we will create a Map and then create a ConcurrentHashmap from Map.
Output
Code Explanation In the Code Above we have first created a Map with some values. Then Created a ConcurrentHashMap by passing HashMap that we just created.
Table for ConcurrentHashMap Class Methods
Methods | description | code |
---|---|---|
put() | add the given key/value mapping to the map | map.put(key,value) |
putAll() | add all the entries from given map to this map | ConcurrentHashMap.putAll(AnotherMap); |
putIfAbsent() | add the given key/value mapping to the map if the given key is not present in the map | ConcurrentHashMap.putIfAbsent(key, value); |
entrySet() | returns a set of all the key/value mapping of the map | Set<Entry<String,String>> entry = map.entrySet(); |
keySet() | return a set of all keys in map | Map.keySet() |
values() | return a set of all values in map | Map.values() |
get() | returns the value corresponding to the key supplied. If the key cannot be located, it returns null. | map.get(value); |
getOrDefault() | returns the value corresponding to the key supplied. If the key cannot be located, it returns the default value. | map.getOrDefault(key, value); |
remove(key) | returns and deletes the entry from the map that corresponds to the key supplied. | value=Map.remove(key); |
remove(key, value) | only removes the entry from the map if the key and value match and returns a boolean value. | boolean result = Map.remove(key, value); |
forEach() | The supplied function is carried out by the forEach() method while iterating through our entries. | map.forEach(4, (k, v) -> System.out.println("key: " + k + " value: " + v)); |
search() | The search() method looks up the element in the map using the given function, then it returns it. | map.search(4, (k, v) -> {return v == 3 ? k: null;}); |
reduce() | Each entry in a map is accumulated (combined) via the reduce() method. When we need to add all the values in a map, for example, this can be used to add all the entries. | map.reduce(4, (k, v) -> v, (v1, v2) -> v1 + v2) |
Operations on ConcurrentHashmap
Adding Elements
1. put() adds a key-value pair with the provided values to the map. Example
In the code below we are going to create a concurrent hashmap and add some elements to the map using the put method.
Output
2. putAll() adds every entry from a given map to current map.
Example
In the code below we are going to create a concurrent hashmap and add elements of other map by using putAll() method.
Output
3. putIfAbsent() if the key supplied does not already exist in the map, puts the specified key/value mapping into the map.
Example
In this example we are going to create a ConcurrentHashmap and only add elements if they are not present in the map using putIfAbsent() method.
Output
Access ConcurrentHashMap Elements
1. entrySet() Returns a set of all of the map's key/value mappings.
Example In this example we are going to print Set of Entries in ConcurrentHashMap using entryset method.
Output
2. keySet() provides a Set of all the map's keys.
Example In this example we are going to print Set of Keys in map using keyset() method.
Output
3. values()
returns a Set of all the map's values.
Example In this example we are going to print set of values in ConcurrentHashMap using values() method.
Output
4. get()
Returns the value corresponding to the key supplied. If the key cannot be located, it returns null.
Example In this example we are going to print the value corresponding to key.
Output
5. getOrDefault() Returns the value corresponding to the key supplied. If the key cannot be found, returns the provided default value.
Example
In this example we are going to print the value corresponding to key using getOrDefault() method.
Output
Remove ConcurrentHashMap Elements
1. remove(key returns and deletes the entry from the map that corresponds to the key supplied.
Example In this example we are going to remove element from ConcurrentMap using remove() method.
Output
2. remove(key, value)
Only removes the entry from the map if the key and value match and returns a boolean value.
Example In this example we are going to remove element from ConcurrentHashmap using remove() method.
Output
Bulk ConcurrentHashMap Operations
Different bulk operations can be securely applied to concurrent maps using the ConcurrentHashMap class.
1. forEach() Method
The supplied function is carried out by the forEach() method while iterating through our entries.
There are two parameters in it.
The parallelismThreshold sets the number of items at which operations in a map begin to run concurrently. transformer: Before the data is delivered to the specified function, it will be transformed.
Example In this example we are going to print each key value pair using foreach method.
Output
In the programme mentioned above, parallel threshold 4 was given. This indicates that if the map has four entries, the procedure will be carried out simultaneously.
2. search() The search() method looks up the element in the map using the given function, then it returns it.
The entry to be searched in this case is decided by the supplied function.
Additionally, parallelThreshold, an optional parameter, is included. The parallel threshold indicates how many map components must pass before an operation is carried out in parallel.
Example In this example we are going to search for key from ConcurrentHashMap having value 3.
Output
3. reduce() Method Each entry in a map is accumulated (combined) via the reduce() method. When we need to add all the values in a map, for example, this can be used to add all the entries.
There are two parameters in it.
The parallelismThreshold sets the number of items at which operations in a map begin to run concurrently. Transformer: Before the data is delivered to the specified function, it will be transformed. Example In this example we are going to find the sum of the map using reduce method.
Output
Code Explanation
4 is a parallel threshold here (k, v) -> v is a transformer function. It transfers the key/value mappings into values only. (v1, v2) -> v1+v2 is a reducer function. It gathers together all the values and adds all values.
In this case, (k, v) -> v is a transformer function, and 4 is a parallel threshold. The key/value mappings are converted into values alone. A reducer function is (v1, v2) -> v1+v2. It compiles all the values and adds them all.
have to work on formatting
ConcurrentHashMap vs HashMap
Parameter | ConcurrentHashMap | HashMap |
---|---|---|
Synchronization | Synchronized | Not synchronized |
Thread Safety | Thread-safe | Not thread-safe |
Null values | Not allowed (NPE) | Allowed |
Performance | Slower than HashMap | Faster than ConcurrentHashMap |
Java Version | Since 1.5 | Since 1.2 |
Multi-threaded Environment | Performs better | Not scalable |
Single-threaded Environment | Slightly lower | Slightly better |
Conclusion
- ConcurrentHashMap is a part of the java.util.concurrent package
- It also implements the Serializable interface and ConcurrentMap
- Because the ConcurrentHashMap class is thread-safe, several threads can work together seamlessly on a single object.
- ConcurrentHashMap provides variety of constructor by which we can initialize map with a given initial capacity,Concurrency-Level,Load-Factor.
- ConcurrentHashMap provides different method by which we can insert,delete elements
- The ConcurrentHashMap class provides different bulk operations that can be applied safely to concurrent maps.
- Different bulk operations can be securely applied to concurrent maps using the ConcurrentHashMap class.