What are Constants in Python?
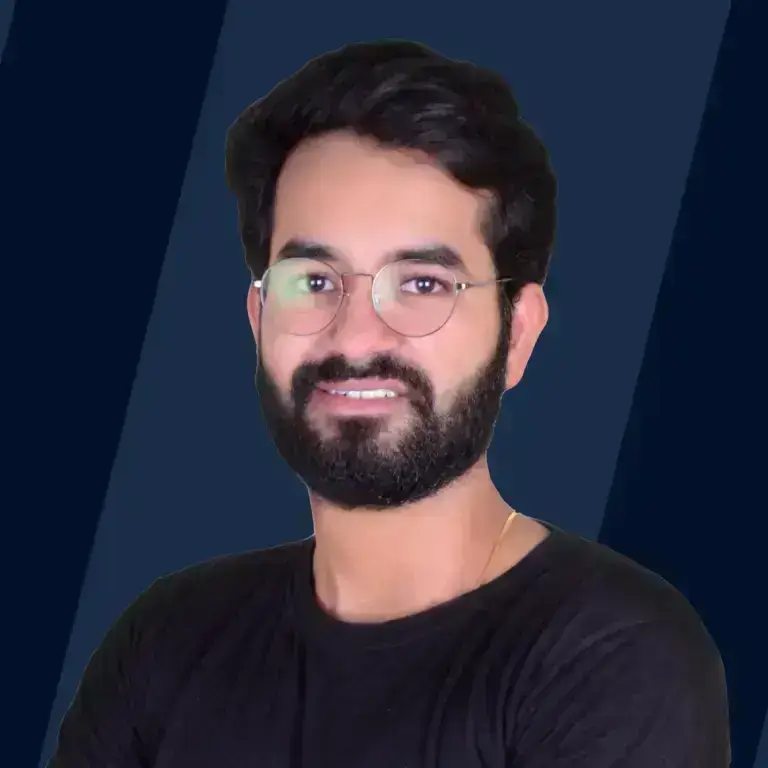
The term "constant" in programming refers to names denoting values that don't change while a program is being executed. Python developers frequently use constants, which are fundamental ideas in programming. Python doesn't, however, have a specific syntax for defining the constants. Constants in Python are simply static variables in actuality.
Programming constants have two components, just like variables: a name and a corresponding value. The constant's identification will be made very clear in the name. The value associated with a specific constant can be of any data type, just like with variables. So you can specify constants for integers, floating points, characters, strings, and more.
Once a constant has been defined, you can only perform one operation on it. The constant's value cannot be changed over time, you can only access it. A variable, on the other hand, enables you to access its value and reassign it.
How to Define Constants in Python?
The unfortunate news is that constants are not supported by Python. To get around this, you can name a variable in all upper case letters to signal that it should be handled as a constant. For example:
Keep in mind that you created these constants in the same manner as you would variables. The assignment operator (=) and the precise value of the constant were utilized along with an illustrative name.
You can convey that the current name is intended to be considered as a constant—or more accurately, as a variable that never changes—by using only capital letters. As a result, other Python programmers will be aware of this and hopefully refrain from assigning anything to the relevant variable.
When to use Constants in Python?
There are many cases of constant values, or values that never change, in life and science. Some examples include:
- 3.141592653589793: A number symbolized by π, or Pi in English, that shows the ratio between a circle's circumference to its diameter.
- -273.15: A constant used to denote the temperature of absolute zero, or 0 kelvins on the Kelvin scale, in degrees Celsius.
- 2.718281828459045: It is Euler's number, which is a constant symbolized by the letter e and is intimately related to the natural log and compound interest.
The constant values mentioned above are all ones that are frequently used in life and science. These and numerous other similar numbers that you can think of and treat as constants are things that you'll frequently encounter when programming.
Therefore, a constant can be used to represent any type of data that is expected to remain constant throughout its existence, such as a quantity, magnitude, parameter, etc.
How to Handle Constants in Python with real-world projects?
Now it's time to learn how to manage and arrange constants in a practical project now that you understand how to generate them in Python. To do this, you might use several methods or tactics. You may, for instance, enter your constants in:
- The same file as the code that uses them
- Dedicated module for project-wide constants
- Configuration file
- Few environment variables
There are a few real-world examples that illustrate how to effectively handle constants:
Putting Constants Together With Related Code
To organize and manage your constants, the simplest and perhaps most natural approach is to define them simultaneously with the code that utilizes them. By using this method, the constants will be defined near the top of the module that holds the relevant code.
Consider the situation when you need to employ math constants such as Pi, Euler's number, and a few others when building a custom computation module. In this situation, you could take the following actions:
Output
Note:
A leading underscore (_) can be added to a constant's name to indicate that it should only be used within its containing module. You may enter _PI = 3.141592653589793 as an example. The name is marked as non-public by the leading underscore, so the user's code shouldn't use directly.
Creating a Dedicated Module for Constants
Making a dedicated module to hold all of your constants is another popular method for managing and organizing them. This approach is suitable for constants that are used across a project in numerous modules and even packages.
The main goal of this approach is to provide constants with a clear and distinctive namespace. Create a Python package with the following files to use this approach with your example calculations:
The calc directory will become a Python package because of the __init__.py code. You may then include the following information in your constants_.py file:
Now you can import this module whenever you want to utilize any one of your constants after adding this code to constants.py:
Output
Storing Constants in Configuration Files
Let's imagine that you wish to externalize the constants of a particular project in more detail. It could be necessary to keep all of your constants out of the source code for your project. You can accomplish this by using an external configuration file.
An illustration of how you can move your constants to a configuration file is shown below:
INI file formats are used in this document. Using the configparser module from the standard library, you can read this kind of file.
Return to calculations_.py now and make the necessary updates so that it looks like the following:
In this example, the configuration file is first read by your code, and the resulting ConfigParser object is then saved in a global variable called constants. This variable can also be called CONSTANTS and used as a global constant. To read the constants from the configuration object itself, you then change your computations.
The configuration parameters are stored as strings in ConfigParser objects, thus you must use the built-in float function for converting the values to numbers.
This method may be useful if you're developing a graphical user interface (GUI) program and need to define specific parameters, such as the size and form of the app's windows when the GUI loads and is displayed.
Handling Constants as Environment Variables
Defining your constants as environment variables on macOS or Linux or system variables on Windows is another useful technique for managing your constants.
The configuration of deployment in various settings is frequently done using this method. Constants that suggest security issues that shouldn't be explicitly committed to the source code can likewise be stored in environment variables. These constants include, for instance, API access tokens and authentication credentials.
Note: While using environment variables for sensitive data, you should be careful because they could be unintentionally exposed in logs or to child processes. All cloud service providers provide some form of more secure secret management.
To use this technique, your operating system must first export your constants as environment or system variables. At least two methods exist for doing this:
- Export the constants manually from the current shell session.
- Add the constants to a shell's configuration file.
The first method is fairly quick and useful. It can be used to quickly test your code. Let's take the scenario where you must export an API token as a system or environment variable. Then you simply need to issue the following command:
Your constants will only be accessible from the command-line session in which you defined them, which is the biggest disadvantage of this method. Making your operating system load the constants each time you launch a command-line window is a far better strategy.
You may access the configuration file for your shell in your home folder if you use Linux or macOS. Open that file, and after it is finished, add the command given below:
# .bashrc
export API_TOKEN="593086396372"
The environment dictionary now contains your API TOKEN constant. Therefore, with just two lines of code, you can see them below:
Exploring Other Constants in Python
Python defines several internal identifiers that might be regarded as constants in addition to user-defined constants. Some of these names are strict constants, which means that once the interpreter is running, you cannot modify them. This is true, for instance, of the __debug__ constant.
You'll discover several internal Python names in the parts that follow, which you can use as constants in your code. You will first go through various built-in constants and their values.
Built-in constants
In Python Boolean values True and False are the first two constants provided in the documentation. Both of these numbers are examples of int. The value of True is 1, whereas the value of False is 0:
None, which in Python is equivalent to the null value, is another significant and widely used constant variable.
Another constant value in Python is the ellipsis literal (...). Ellipsis can be used as a stand-in for unwritten code. It may also be used in place of the pass statement.
As you have learned just at beginning of this part, the built-in constant __debug__ is another interesting and maybe practical constant. The default value of the Boolean constant __debug__ in Python is True. Because you cannot alter its value once your interpreter has started, it is a strict constant.
The assert statement and the constant __debug__ are closely the same. In essence, all of your assert statements will execute if __debug__ is True. Your assert statements will not run at all if __debug__ is set to False. This functionality might help your production code run a little bit faster.
Conclusion
- You now understand what constants are, how and when to apply them in your code, as well as their purpose.
- As a naming convention, the Python community utilizes uppercase letters to indicate that this variable should be referred to as a constant. Other developers will not alter constant variables thanks to this naming policy.
- In a real-world project, managing constants can be done in a variety of ways.