Context Manager Python
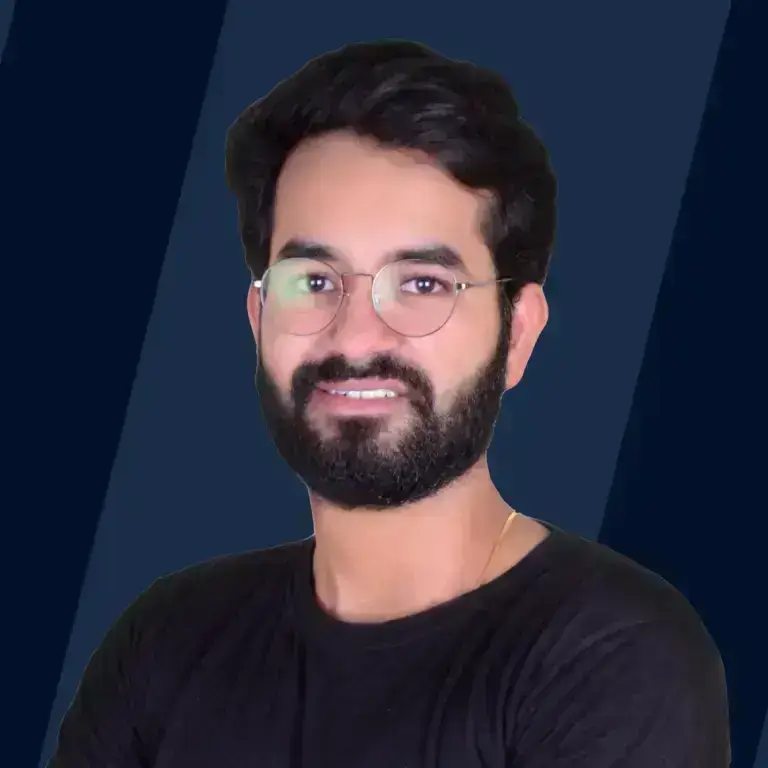
In Python, managing resources like files or network connections efficiently is crucial. Context managers are a game-changer for this task. They help you allocate and release resources precisely when you need them. Think of it as borrowing a book from the library: you take it when needed and return it once done. This not only makes your code cleaner but also prevents common pitfalls like memory leaks.
What is a Context Manager in Python?
A context manager in Python elegantly manages resources, ensuring setup and teardown operations are automatically handled. Commonly used with the with statement, it neatly manages resources, such as files or database connections, mitigating the risk of common errors like leaks or unreturned resources.
For instance, handling files without a context manager could lead to complexities, especially in error scenarios, potentially causing a file to remain open unintentionally. This manual management is error-prone, often leading to overlooked cleanup operations.
Python's context management protocol, utilizing __enter__ and __exit__ methods, automates this process. When entering a with block, __enter__ executes to set up the resource (like opening a file), and upon exit, __exit__ ensures proper teardown (like closing the file), even if exceptions occur within the block. This automation streamlines your code, enhancing readability and safety by removing repetitive, error-prone setup and cleanup tasks. It's a testament to Python's design philosophy, offering a concise, robust solution for resource management, and elevating code quality by focusing on the core logic rather than the mundane details of resource handling.
Managing Resources using Python context manager
Python simplifies resource management, like handling files, through context managers, using the with statement. This approach ensures resources are automatically managed, eliminating the need for extensive try-except-finally blocks found in other languages. Whether dealing with exceptions or complex code paths, context managers guarantee the clean allocation and release of resources. Created via classes or decorated functions, they streamline resource handling, making your code more robust and readable by taking care of the setup and teardown processes for you.
Create a Custom Context Manager in Python
Crafting your own context managers in Python offers a robust solution for better handling of resources like files, network connections, and, most notably, database connections. It guarantees that these resources are utilized effectively and securely in your code, significantly reducing the potential for leaks or similar problems. Python enables the development of context managers via two principal techniques: those based on classes and those utilizing generators. Each strategy is designed to make resource management more efficient, thereby boosting the durability and upkeep of your code. Let's dive into both methodologies.
Create a Class Based Context Manager in Python
A class-based context manager in python involves defining a class that implements two critical methods: __enter__ and __exit__. The __enter__ method is called at the start of a with block, where it initializes the resource and returns it (or an object representing it). This method is where you would typically open a database connection or a file. On the other hand, the __exit__ method is invoked when the with block is exited, either normally or because of an exception. It is responsible for cleaning up the resources, such as closing the database connection. It accepts three arguments related to the exception, if any occurred, allowing you to handle exceptions gracefully or perform additional cleanup steps.
Consider the following example of a class-based context manager for a database connection:
In this example, the DatabaseConnection class manages a SQLite database connection. It opens the connection in the __enter__ method and ensures the connection is closed in the __exit__ method, regardless of whether an exception was raised within the with block.
Create a Generator Based Context Manager in Python
The generator-based approach leverages the contextlib module, specifically the contextmanager decorator, to create a context manager from a generator function. This method is often more concise than class-based context managers and can be more readable for those familiar with Python's generators. The generator function performs the setup before yielding the resource to the with block and handles cleanup after the yield statement. This approach is particularly convenient for simple resource management tasks.
Here's an example of a generator-based context manager for a database connection:
This function creates and yields a database connection that is automatically closed once the with block is exited, even if an exception occurs.
File management using Python Context Manager
We have seen a basic code for how to implement the context manager in python. Now let's move to create the context manager python to handle or manage a file.
In the above code, we have created a class FileManager and in which we have defined three methods which were discussed above: init, enter, and exit.
- The init function will just initalize the file variable and will set its mode as well.
- In the enter function, the file will be opened in the mode defined in the init function using open method.
- At last, in the exit method by using the close method the current file will be closed which is the main purpose of the context manager.
How to Manage Database Connection by using Context Manger and with statement in Python?
We have seen the implementation of the context manager in python for the databases using class. Now let's create an object for the above-defined class and use the ‘with’ statement to close the file automatically after the use. Let’s see its code first to see the implementation of managing a database using python context manager and the with statement:
In the above code, we have implemented a connection manager for the MongoDB database. First, we have imported the MongoClinet from the pymongo library to work with the MongoDB database.
In the class MongoDBConnectionManager(),
- First we implemented the init function for taking the parameter for the connection making.
- Then after implemented the enter function, in which we have made the connection using MongoClient() and returned it.
- At last in the class, we have defined the exit function to close the connection using close() method.
We have used the with statment to make connection with localhost at port 27017 and collected some data from it.
Conclusion
- Context managers in Python automate resource management, ensuring efficient handling of files and connections, even amid errors.
- They streamline complex code, reducing error risks and simplifying maintenance.
- Utilization clarity improves with context managers, enhancing code readability and resource management.
- Python offers versatile creation methods for context managers, providing flexibility for developers.
- They enhance error management and cleanup by ensuring resource release during exceptions.