Continue Statement in C
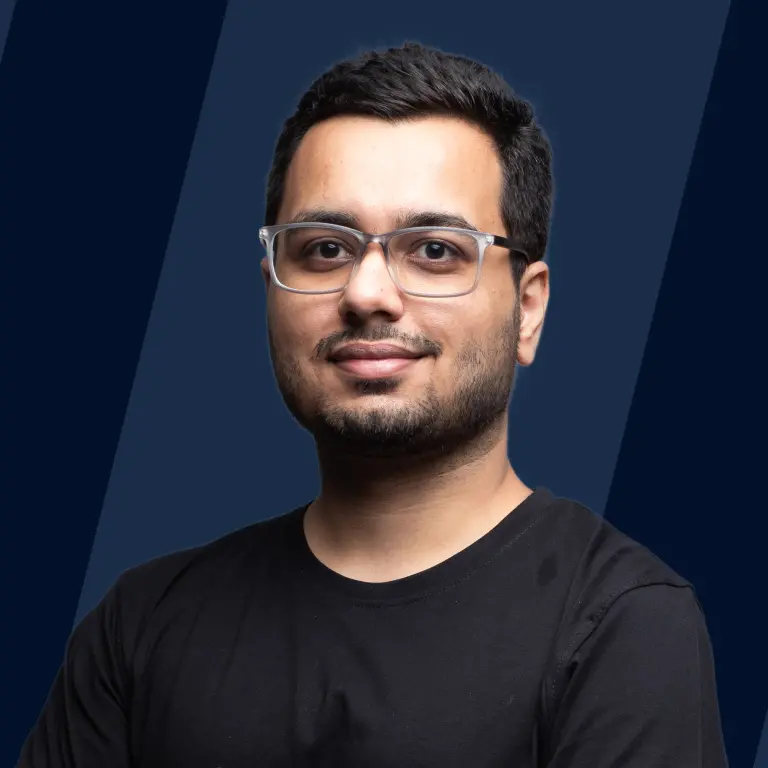
The continue statement in C is used within loops to skip the current iteration and proceed to the next one. It helps control the flow of the program and is often used to skip specific iterations based on a condition.
What is continue in C?
The continue statement in the C programming language is a control statement used primarily within loops (such as "for" and while loops) to alter the flow of program execution. When encountered, continue immediately jumps to the next iteration of the loop, bypassing any code that follows it within the current iteration. This is particularly useful when you want to skip specific parts of a loop based on a certain condition. For example, you might use continue to avoid executing a block of code for certain loop iterations. By doing so, it enables more efficient and fine-grained control over loop execution, making it a valuable tool for managing program logic and iteration in C.
Syntax of continue in C
In C, continue is reserved as a keyword, and the syntax of the continue statement is as follows -
Use of continue in C
- Skip Current Iteration: The continue statement is used to skip the remaining code within the current iteration of a loop.
- Control Loop Flow: It allows for fine-grained control of loop execution by bypassing specific iterations.
- Conditional Skipping: You can use continue in combination with conditional statements to skip iterations based on certain conditions.
- Efficiency: It helps improve the efficiency of your code by avoiding the execution of unnecessary code during specific loop iterations.
- Common Use Cases: It is often employed to handle exceptional cases or to exclude specific values from loop processing.
- Versatility: continue is compatible with different loop types, such as "for," "while," and "do-while" loops.
- Programming Logic: It is a valuable tool for managing program logic and ensuring that the code executes as intended within loops.
- Careful Usage: Care should be taken when using continue to avoid potential infinite loops or unintended logic errors.
Examples of Continue Statement in C
Let us look at a simple example to understand how the continue statement is used in C.
Output
Here, the above code prints the variable number when the value of number is greater than five and skips the iteration using the continue statement when the value of number is less than five.
How Continue Statement Works?
- Encounter "continue": When a continue statement is encountered in a loop, it immediately skips the remaining code within the current iteration and moves to the next one.
- Skips Code: All code following continue in the current iteration is ignored.
- Conditional Use: It is often used with conditional statements to skip specific iterations based on conditions, allowing fine-grained loop control.
Flowchart of continue in C
Now that we understand, the continue statement is used to skip iterations in a loop. Let us see a flow diagram to visualize how continue works in a loop.
From the flow chart mentioned above, you can observe that as soon a continue statement is encountered inside a condition, the execution of code skips the conditions following the continue statement, and the next iteration of the loop begins till the loop condition is met. The loop condition continues till the condition of the loop is satisfied, and the program exists loop when the condition becomes false.
Notice the code below the continue statement only executes when the continue statement is not encountered inside the loop body.
Difference between break and continue Statements in C
Aspect | break Statement | continue Statement |
---|---|---|
Usage | Used to exit the loop prematurely and proceed with the code outside the loop. | Used to skip the remaining code within the current iteration and continue with the next iteration of the loop. |
Effect | Terminates the entire loop when encountered. | Skips the code following the continue statement in the current iteration, but the loop continues with the next iteration. |
Control | Provides an abrupt exit from the loop structure. | Provides a means to control loop execution with fine-grained skipping. |
Common Use Cases | Typically used to exit a loop early based on certain conditions. | Commonly used to exclude specific iterations of a loop based on specific conditions. |
Scope | Affects the entire loop structure. | Affects only the current iteration of the loop. |
Learn more about Difference between break and continue Statements in C.
Conclusion
- Continue statement in C passes programs control to the next iteration of the loop, i.e., nearest enclosing do-while, while, or for statement skipping any remaining statement in the loop body.
- The syntax of the continue statement in C is - continue;.
- Continue statement in C can be used with for, while, and do-while loop.
- Continue statement in a for loop causes evaluation of loop condition of the for loop statement. The code re-evaluates the conditional expression, and depending on the result, the loop either terminates or continues iteration to the loop body.
- For both the while and do-while loops, the continue statement skips the iterations and re-evaluates the loop's conditional expression.