Convert Dictionary to JSON Python
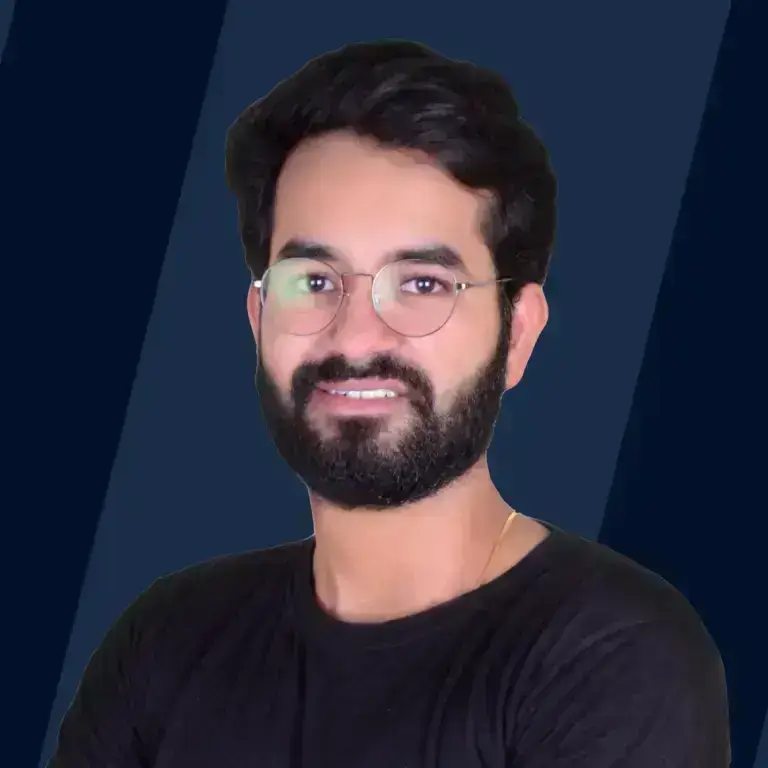
Overview
While executing any program, we need data to work with. This data is either provided at the time of execution or built into the program. To store data in the most organized and easy-to-handle manner, we have the JSON format. While in python, the most formatted manner to store data is dictionaries, where data is stored as a sequence of elements like a map data structure containing the key: value pairs. In the below article, we shall be understanding how we can make use of json.dumps() function by utilizing its various parameters to convert the dict to JSON python.
What is a Dictionary in Python?
While coding we might have come across scenarios where we are required to convert dict to JSON python and store elements in pair format where one represents the key and the other represents its value. To simplify the coding experience, python has a dictionary for the same.
We define a dictionary as an unordered collection of data values where we can store data values like a map. A dictionary holds the elements in the form of pair elements where one is the key while the other corresponds to values in the key: value structure. This key: value structure is marked within the curly {} braces and separated by ‘comma’ to make out the different key: value pairs. One important point to note here is that the dictionary is the case- sensitive that is, in a dictionary, the values can be of any data type and even duplicated, while we need to keep the keys unique and immutable. An empty dictionary also exists where we can create it by placing curly braces{}.
Syntax:
The syntax of a dictionary is a pair of key: value pairs as shown below:
Dictionary = {1: 'Hello', 2: 'Hi', 3: 'Hey'}
As seen above in the 'Dictionary' we have three distinct keys with three values.
Parameter:
The parameter that we need to pass while creating a dictionary is the key: value pairs marked by the curly braces. As shown above, we have three distinct keys and their respective values marked by the curly braces as the collection of data values representing the dictionary.
To know more about the dictionary in python, please read dictionary in Python blog to expand your knowledge!
What is JSON in Python?
While writing files in JSON format, a good structure format is followed, which is quite easy to handle. JavaScript Object Notation, also abbreviated as JSON is the most popular, organized, and easy-to-handle data format that is used for representing the data structurally. We often transfer and receive data between a web browser and a server in JSON format. The JSON is a simple text that is written in the javascript object notation mostly used as a syntax for storing and exchanging data.
While working with Python, we can make use of this JSON format by simply importing the built-in JSON package that can be used to work with JSON data. The below diagram represents what a JSON format looks like.
With the help of the JSON python module, we can work with JSON objects including parsing, serializing, deserializing, and many more. It also enables us to convert the dict to JSON python and work on it efficiently.
Syntax:
The syntax for a JSON which in python exists as string format:
As seen above in the 'JSON' we have two distinct keys with values against them and it's pretty common to store the JSON keys in file format.
Parameter:
The parameter that we need to pass while creating a JSON string is the object and the corresponding value that is marked by the square brackets and the JSON string is enclosed with curly braces. As shown above, we have two distinct keys and their respective values marked by the square braces as the collection of data values representing the JSON string.
To know more about JSON in Python, please refer to this blog and expand your knowledge!
Difference between Dictionary and JSON
Listed below are a few key differences you can find between a Dictionary to JSON Python. We have managed to put the differences in an easy-to-understand tabular format as it will distinguish between the two and we shall learn where we can leverage the dictionary or JSON as per our use case.
JSON | Dictionary |
---|---|
The keys in JSON can be only strings. | The keys in the dictionary can be any hashable object. |
In JSON, the keys are sequentially ordered and can be repeated. | In the dictionary, the keys cannot be repeated and must be distinct. |
In JSON, the keys have a default value of undefined. | Dictionaries do not have any default value set. |
IN JSON file format, the values are accessed by using the “.”(dot) or “[]” operator. | In the dictionary, the values are mostly accessed by the subscript operator. For example, if 'dict' = {'A':'123R' ,'B':'678S'} then by simply calling dict['A'] we can access values associated. |
We are required to use the double quotation for the string object | We can use either a single or double quote for the string objects |
The return object type in JSON is a ‘string’ object type | The return object type in a dictionary is the ‘dict’ object type |
Python Program to Convert Dictionary to JSON
Now as we learned theoretically about the dictionary and JSON, we shall now walk through some scenarios where we shall explore various code examples to see how we can convert a dictionary to JSON python.
Method 1: Using dumps() Function
In python, we have an inbuilt module called 'JSON' which has an in-built function called dumps(). The dumps() function is used to convert dict to JSON python. We can do this by simply importing the "JSON" module which helps to easily parse the JSON strings that contain the JSON object.
Syntax: The syntax for the dumps() function is as below: json.dumps(dictionary, indent)
Parameters: As seen above, we have two parameters that are being passed that is, dictionary and indent. For the dictionary, we need to put the name of the dictionary which we want to convert into the JSON object. For indent, we mention the number of units for indentation. It is mandatory to always mention the dictionary parameter to tell in which dictionary should the dumps() function work. The indent parameter is optional.
Code:
Output:
Explanation: As seen above, we have started by importing the inbuilt JSON module from the python library. Then we are creating a dictionary and store some key: value pairs in it. We then implement the dumps() function to convert this dictionary into JSON format where we pass the dictionary and the indentation value as 8. Finally, we print the JSON object using the print statement and hence achieve the goal to convert the dictionary to JSON python.
Method 2: Converting Nested Dictionary to JSON
A nested dictionary in python can be created by simply declaring new dictionaries in the default dictionary. To convert dict to JSON python, we can use the dumps() function itself. Here, we have used indent=3, which refers to the space at the beginning of the code line:
Code:
Output:
Explanation: As seen above, we have started by importing the inbuilt JSON module from the python library. Then we are creating a dictionary named dictionary_employees and store some key: value pairs in it. We then print the dictionary before we can actually convert dict to JSON python. Then, we implement the dumps() function with the dictionary and indent as parameters to convert dict to JSON python-format where we pass the dictionary and the indentation value as 9. Finally, we print the JSON object using the print statement and hence achieve the goal to convert dictionary to JSON python.
Method 3: Convert Dictionary to JSON Quotes
Now we are heading towards a scenario where we can convert dict to JSON python quotes. JSON quotes return the output in double quotes in JSON format as shown below.
Here we start by declaring a class. This class is used for the string representation to convert a dictionary into a JSON object. Then, by using the str(self) method and the variables 'assemble' and 'output' are declared which will help to assign with the class and convert the dictionary mentioned into the JSON object.
Code:
Output:
Explanation: In the above example, we are converting the dictionary to JSON quotes. Here we start by declaring a class called vegetables. This class is used for the string representation to convert the dictionary into a JSON object. Then, by using the str(self) method, we return the JSON object by using the dumps() function on self. We have declared the variables 'assemble' and 'output' that helps to assign with the class and convert the dictionary mentioned into the JSON object. Finally, we print our JSON object and achieve our goal to convert dict to JSON python.
Check out this article, also to learn more about Self in Python.
Method 4: Convert Dictionary to JSON Array
The JSON array is represented in an array format where we make use of for loop to print the key and value pair after validating them as shown below.
When we want to convert the dict to json python array, we first check the keys and values of the dictionary before converting it into a JSON object. Here we implement the for loop that will help to store the value. Then by using the dumps() function, we store the dictionary.
Code:
Output:
Explanation: In the above example, we are converting the dictionary into a JSON array. We first create a marks_dictuonary where we add some keys along with the values. Then we declare a marks_array where we check if we have respective keys and values for it, and we start to add them by using the for a loop. Then by implementing the dumps() function, we store the dictionary. Finally, we print our JSON array and achieve our goal to convert dict to JSON python.
Method 5: Convert Dictionary to JSON using sort_keys
The sort_keys is a parameter in json.dumps() function which allows us to sort the dictionary and convert dict to json python object if kept TRUE else not.
To convert a dict to JSON python using sort_keys, we use the sort_keys attribute inside the dumps() function to achieve the same. We need to set it to "True" to sort the dictionary and convert it into the JSON object. When we set it to False, then the dictionary will not be sorted to find the JSON object.
Code:
Output:
Explanation: As seen above, we can convert the dictionary to JSON using sort_keys. For this, we first need to import our inbuilt JSON module in python. Then we created a dictionary where we stored a few keys with their respective values. Then we used the dumps() function from the JSON module where we declared the dictionary (employee_dictionary ) along with indentation as 8 and gave the sort_keys attribute as TRUE. This attribute will now be able to sort the dictionary and convert it into the JSON object. Finally, we print the json object as seen from the output and achieve our goal to convert dict to JSON python.
Method 6: Convert Dictionary to JSON using Indentation
Here we shall be looking into the example where we shall be converting the dict to JSON python using the indentation. We learned before that the dumps() function needs one mandatory parameter after which it can take the dictionary object and convert it into a JSON string. With the following code, we shall create a dictionary named employee_dictionary containing the data of a particular employee record. Then we shall use the dumps() function with one argument which shall convert employee_dictionary into JSON data. Then, we make use of the dumps() function and pass two arguments where we give the employee_dictionary as one indentation value as 8 for JSON data. We finally see that with indentation the JSON output is different from that without indentation. One point to note is that the result of both the dictionary and JSON object is the same when no indentation is followed or used.
Code:
Output:
Explanation: As observed before, we have used the dumps() function to convert the dictionary to JSON format. Now here when we pass only one argument as its mandatory to pass at least one argument in the dumps() function then we see the output as a JSON string which is similar to the output we got from the dictionary and when we pass two arguments in the dumps() function then we see that the by utilizing the indentation value as 8 it got changed as can be seen above in the output and achieve our goal to convert dict to JSON python.
Method 6: Converting Empty Dictionary to JSON
With the below code example, we will be converting an empty dict to JSON python format. To implement so, we start by passing an empty dictionary and implementing the dumps() function to convert it into the JSON format. As nothing was mentioned in the dictionary no unknown value appears in the JSON format.
Code:
Output:
Explanation: As seen above, we have an empty dictionary which we pass on as the argument in the dumps() function which converts it into JSON format. As the dictionary was empty hence we get the output of the JSON as empty and achieve our goal to convert dict to JSON python.
Method 7: Converting Dictionary with Different Types of Values to JSON
With the below example, we shall be exploring how can we convert the dictionary with different types of values to JSON. As a dictionary consists of a key: value pair, in this example we shall take a dictionary with different datatypes of values and convert it into the JSON string by implementing the dumps() function.
To do so, we shall use the json.dumps() function and pass the dictionary as a parameter. Finally, we obtain a JSON format with different types of data types.
Code:
Output:
Explanation: As seen above, we have a dictionary where we have key-value pairs with different data types. The first key: value pair is of string data type, while the second is of integer data type and the third is a float data type. While we use the dumps() function to convert the dictionary into a JSON format as shown above even when the datatypes in the dictionary are different.
Conclusion
-
A dictionary is an unordered collection of data values that can store data values in a map-like format. The dictionary holds the elements as pair of values in the [Key: Value] structure.
-
Dictionary = {1: 'Hello', 2: 'Hi', 3: 'Hey'}
-
JavaScript Object Notation, also abbreviated as JSON is the most popular, organized, and easy-to-handle data format that is used for representing the data structurally.
-
The syntax for a JSON which in python exists as string format:
`
- We learned above under the heading - Difference between dict and JSON about some key differences between the two to leverage the positives of dictionary or JSON as per our use case.