Convert double to String in Java
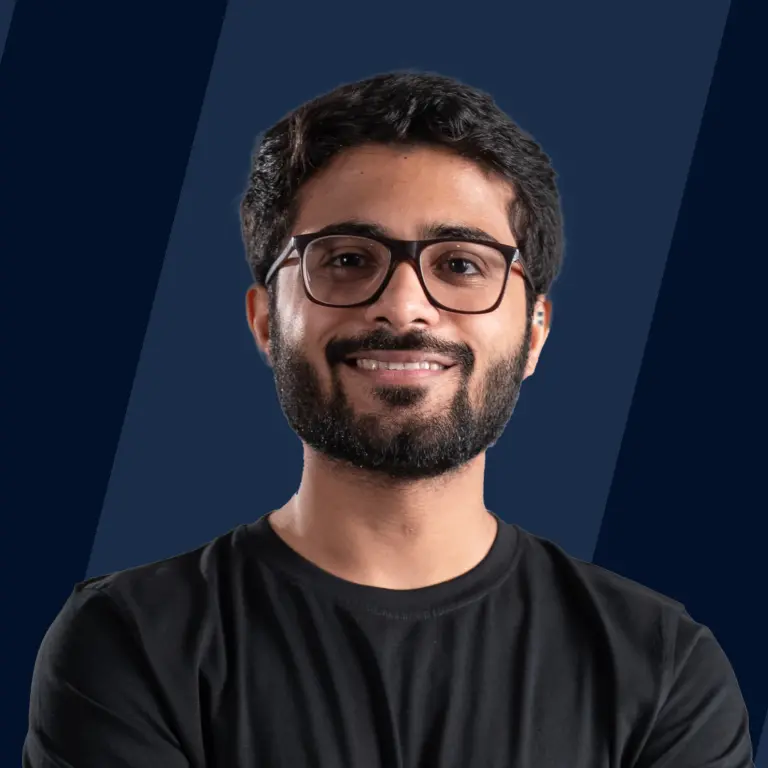
This exploration delves into Java's various methods for converting double to string, essential for efficiently managing extensive numeric data. The conversion is particularly useful when dealing with large streams of numbers that surpass the capacity of conventional data types.
Example 1: Converting from Double to String Using valueOf() Method in Java
String.valueOf() is an inbuilt method of String class in Java to convert different parameters to strings. It works with parameters like an object, float, char, double, int, long, and char[]. Since it is an inbuilt method, it is already present in the java directory which returns the string object.
Syntax:
- String output = String.valueOf(parameter);
Parameters: object, float, char, double, int, float, long, char[]
Let's discuss it with an example:
Example 1: The following code converts the double number to a string using the String.valueOf method.
Output:
Example 2 Let's have a look at another example taking a double value as user input.
Output:
In the above example, we have converted double variable to string using the valueOf() method of the String class. Also, the valueOf() method is the most used way of converting a double variable to a string in Java.
Example 2: Java Program to Convert double to String Using DecimalFormat.format()
Java program can be converted from double to string using the DecimalFormat.format() method by importing java.text.DecimalFormat package into the code. It is used to represent double as a string with a specified number of digits after the decimal and rounded values to decimal places.
Syntax:
OR
Let's see it with an example:
Example 1: The following example specifies the decimal values of the converted number to be 3.
Output:
Example 2: The following example specifies the decimal values of the converted number to be 4. As the digits after decimal in the original number were less than 4, another zero digits get added to satisfy the formatting.
Output:
Example 3: The following example specifies the decimal values of the converted number to be 2. Since the original number has 3 digits after decimal which is more than 2, it rounds off the number to two decimal places and then converts it to string.
Output:
Example 4: The above codes can also be written in a different format as:
Output:
Example 3: Java Program to Convert double to string using StringBuffer and StringBuilder.
We can also convert double to string using both StringBuffer and StringBuilder using the same steps. It first creates an instance, then appends the double value and converts it to a string. The append method accepts a value of any data type as a parameter and adds it to the current object.
Let's explore it using an example.
Syntax:
Parameter: boolean, char, double, float, int, long, string, char[]
Example of converting double to string using StringBuffer:
In this example, we will discuss how to convert double to string using the StringBuffer object. We append a double value to the StringBuffer object using the append() method and get the string value using the toString() method.
Output:
Example of converting double to string using StringBuilder:
In this example, we will discuss how to convert double to string using the StringBuilder object. We append a double value to the StringBuilder object using the append() method and get the string value using the toString() method.
Output:
Example 4: Java Program to Convert double to string using toString()
This is another method to convert double to string using the toString() method of the double class. It stores the primitive double value to the Double class reference variable and changes it to a string using the toString() method.
Syntax:
Example 1: In the following example, we convert the primitive double value by first reading it to the double class reference variable and then converting it using the toString() method.
Output:
Example 2: The double value can also be passed directly to the toString() method or can be taken as an input from a user and passed to the reference variable. Let's have a look at it using an example.
Output:
Here, double is a wrapper class in Java that allows us to convert primitive data types into objects and object into primitive data types.
A print statement in Java always converts other data types to strings using the toString() method of Object class whether called directly or not. When a user creates an object of any type, the toString() method gets overridden so that the print command calls the overridden toString() method. If no object is created by the user, the inbuilt function present in the Java directory automatically converts the double value to string.
Example 5: Java Program To Convert double to String Using + Operator
This method uses the concatenation property of strings to convert a variable of double datatype into the string. The + sign concatenates the string to the number changing it to string type.
Syntax:
Example: The following example helps us add numbers to string. The + operator concatenates the string with the number.
Example 6: Program to Convert double to String using format() method in Java
Java program can be converted from double to string using the String.format() method. It is used to represent double as a string with a specified number of digits after the decimal and rounded values to decimal places.
Syntax:
Example 1:
Output:
Example 2: This method helps us alter the digits after the decimal in the string or round it off to specified decimal places. Let's explore it through an example.
Output:
The above code helps us adjust the number of digits after the decimal to two in the output string. This method helps us in rounding decimals.
Conclusion
Java programs automatically get converted to string at the time of printing.
- If we want to do it for other purposes, we can convert it using the following methods:
- valueOf() - String.valueOf() is an inbuilt method of String class in Java to convert different parameters to strings.
- String.format() - It is used in java to return formatted strings with the specified format and arguments.
- toString() - toString() is an inbuilt function in Java to convert given value to string.
- DecimalFormat.format() - DecimalFormat.format() is a method in java.text.DecimalFormat package to convert double to string with specified number of decimals
- StringBuffer and StringBuilder - StringBuffer and StringBuilder are classes in Java to create modifiable chain and sequence of characters using the append() method.
- +operator - The +operator in Java is used to concatenate strings with two strings. It uses this property to convert double to string.
- Each method can be used for different purposes and places.
- The methods like String.format() and DecimalFormat.format() are used to alter the digits after decimal places and round off the values to their nearest ones.