Convert List to Array Python
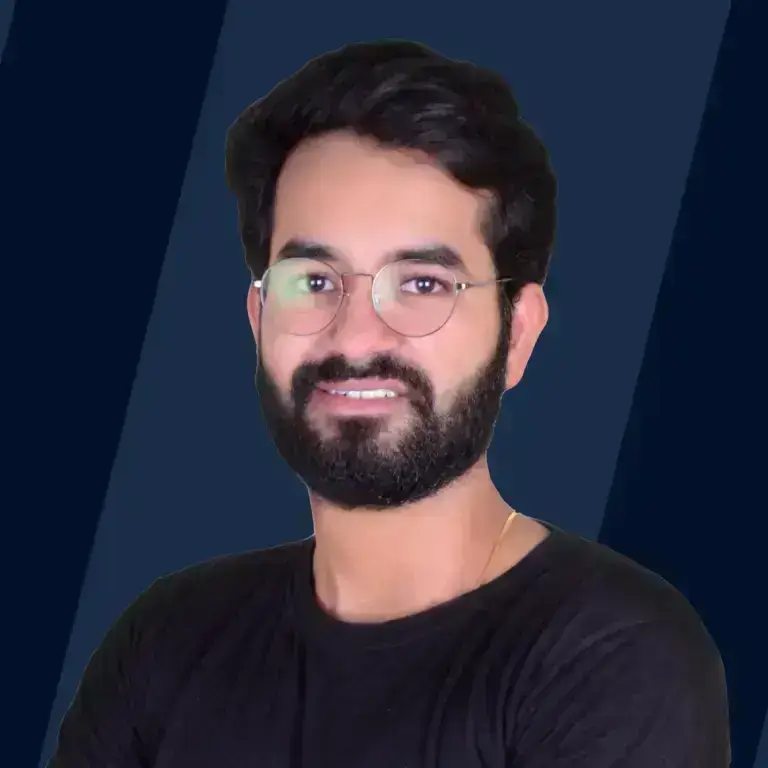
Overview
A list in python is a linear data structure that may hold a variety of elements of different types. A NumPy array is a data structure that can hold homogeneous (same type) items. There are three methods to convert list to array in python and these are, by using the array(), by using the numpy.array(), or by using the numpy.asarray().
Convert List to Array in Python
Before moving to how we can convert a list to array in python, let's have a brief on the list and array in python.
A list in python is a linear data structure that may hold a variety of elements. By varied it can store strings, objects, integers, etc. in a single list. But on the other hand, a NumPy array is a data structure that can hold homogeneous items. So if an array is storing integers, it can only store integers. We can not add data such as string, or object to that integer array.
But you may wonder where can we use such a data structure?
The answer to that is there are some cases when we need to have a data structure that can hold only homogeneous items. Consider the case where we want to store the contact numbers. Have you seen a contact number as "98oi35hj"? No, we have contact numbers in form of integers as 1234567890. Therefore we limit the data type of the array to an integer. Hence if the user tries to enter a contact number as "98ghijo" we can simply return that contact number only accepts integers.
Methods to Convert List to Array Python
There are three methods to convert list to array in python. Let's understand them one by one,
Using array() + data type indicator
Python provides us with an inbuilt function array() that can be used to convert list to array in python. We have to pass the "data type" and the list to the function. This function will convert the list to the provided "data-type". For integers, we pass "i" as the "data-type".
Syntax
Parameters
-
typecode - This is a mandatory parameter that specifies the type of array that we have to create. For integer typecode is "i", for float it is "f", for double it is "d" and so on.
-
initializer - This is an optional parameter that is used to initialize the values of the array. This can be a value, a list, or an iterable of the proper type.
Let's see the program now,
Program
Output:
But what if we provided a string such as "h" to the list? Will it convert it to an array or will return an error? Let's also see an example of this.
Output:
As you can see in the output, we got an error stating that the function was expecting an integer but received a string. This is because in the list there's a string "h" and because of this string we received the error that "an integer is required (got type str)".
Using numpy.array()
In the second method, we can use the array() function provided by the numpy library. This function accepts a list and returns the array containing all the elements of the list.
Program
Output:
Let's do the same test we did above, passing a string to the list.
Output:
You can see in the output, that the numpy.array() converted the list to an array. But that array is storing "string" items.
Using numpy.asarray()
Let's see the definition of numpy.asarray() function,
Parameters
- a This is a mandatory parameter that specifies the input that has to be converted to the array. This can be lists, lists of tuples, tuples, tuples of tuples, tuples of lists and ndarrays.
- dtype This is an optional parameter that specifies the data type of the array. By default, this is inferred from the input data.
- order This is also an optional parameter that specifies whether to use the C-style(row-major) or Fortran-Style(column-major) memory representation. By default, it is set to C-style.
As you can see in the definition that the numpy.asarray() is calling the numpy.array() function. But if it is calling the numpy.array() then what is difference between numpy.array() and numpy.asarray()? Try to think of it by looking at the definition of the numpy.asarray() and we will discuss it in the next section.
Program
Output:
numpy.array() vs numpy.asarray()
Have you guessed the difference between numpy.array() and numpy.asarray()? If not, let's look at the definition of numpy.asarray() again,
Notice, while calling the numpy.array() function it is passing copy=False. This is because by default the value of copy in numpy.array() is true and this will create a copy of the object and then it will convert this object to an array. But on the other hand numpy.asarray() will not create a copy of the object and will convert the given list to an array. Hence we should use numpy.asarray() when we want to save memory.
Related Articles
Conclusion
- A list in python is a linear data structure that may hold a variety of elements. But on the other hand, a NumPy array is a data structure that can hold homogeneous items.
- There are three methods to convert list to array in python and these are, by using array(), by using numpy.array(), or by using numpy.asarray().
- The main difference between numpy.array() and numpy.asarray() is that numpy.array() creates a copy of the object and then converts it to the an array. But the numpy.asarray() will convert the given list to array without creating a copy.