Convert an Object to an Array in JavaScript
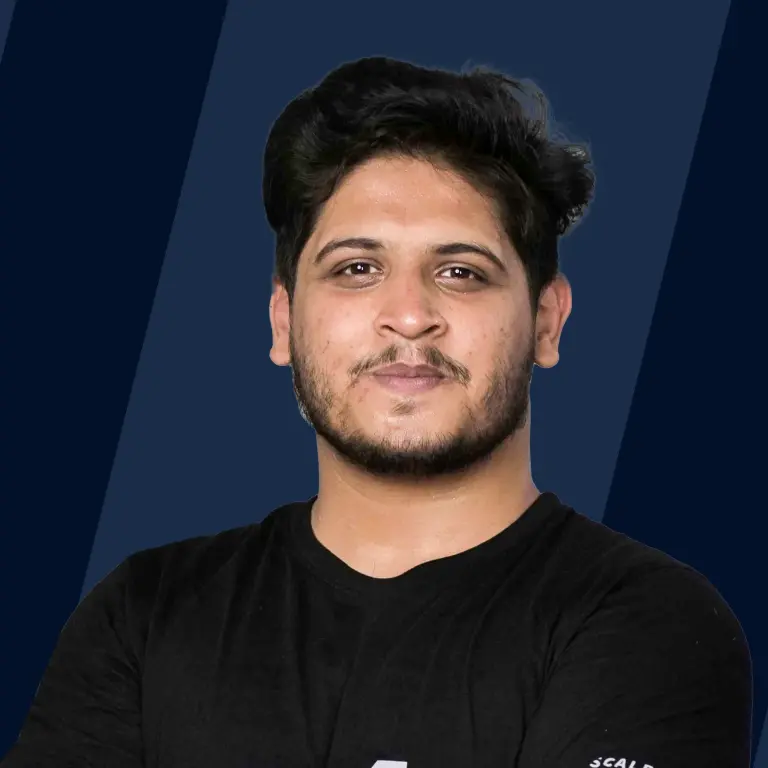
In JavaScript, dealing with an object having a long list of properties can be challenging, for example - printing all values into the object requires iterating over the object and printing the individual key-value. To make the object easy to use, we have Object methods that give the power to convert object to array javascript.
Earlier Approach
Before the introduction of the Object.keys method in the ES6 and Object.values, Object.entries methods in ES8, there were no available methods to convert the object to array javascript. So, the earlier approach was iterating over the object and pushing the individual object properties into a new array.
Example:
Output:
Above, we have the myObj object and an empty array of keys. To convert the myObj object keys into an array we are looping over the myObj using the for...in loop, and in each iteration pushing the individual properties key in the keys array. As a result, we will get an array having all keys of the myObj object.
Using this approach, converting object entries to an array will have time complexity of and space complexity , where N is the length of the object.
ES6 - Object.keys
An earlier approach to converting an object to array javascript was lengthy, to make it short and easy, ES6 introduces the Object.keys method. It returns an array of keys for the given object. To use the Object.keys method, we have to call it by passing the object as an argument.
Example:
Output:
Above, we are calling the Object.keys method on myObj by passing myObj as an argument. As a result, the Object.keys method returns an array of myObj keys which we stored in the myKeys variable and printed on the console. Here, time complexity is and space complexity , where N is the length of the object.
Using ES6 Object.keys method was okay, but it doesn't help other than generating an array of object keys. To perform other operations like- generating an array of object values or all object entries. To do so, ES8 introduces two new Object methods :
- Object.values method
- Object.entries method.
Object.values
Object.value method was introduced in the ES8 which gives the power to generate an array of all object values. To use the Object.values method, we have to call it on the object by passing the same object as an argument.
Example:
Output:
Above, we are calling the Object.values method on myObj by passing myObj as an argument. As a result, the Object.values method returns an array of myObj object values, which we stored in the myKeys variable and printed on the console. Here, the time complexity is and space complexity , where N is the length of the object.
Object.entries
Object.entries method was also introduced in the ES8 which gives the power to generate an array of all object entries. To use the Object.entries method, we have to call it on the object by passing the same object as an argument.
Note : Entries in the object are the key-value pairs separated by a comma.
Example:
Output:
Above, we are calling the Object.entries method on myObj by passing myObj as an argument. As a result, the Object.entries method returns an array of myObj object entries, we also stored the return value in the myKeys variable and printed it on the console. Here, time complexity is and space complexity , where N is the length of the object.
Object.entries + Destructuring
When we use the Object.entries method above, it returns a nested array of entries as a result. Here, each subarray holds an individual key and value separated by a comma. To convert this nested array into a plain one-dimensional array, we have to destructure the nested array. Let's take an example and understand it -
Output:
Above, we are applying the Object.entries method on the myObj object and assigning the return value in the myKeys variable, here return value is a nested array. So, to convert the nested array into a plain one-dimensional array we iterate over the myKeys array, in each iteration pushing the value of individual subarray key and value into the myKeysArr array. As a result, we will get an array myKeysArr having all entries in the object. Finally logging the myKeysArr on the console as output it prints ["name", "your name", "address", "your address"] on the console.
Browser Support
Object.keys method is a feature of ES6 which is supported in all browsers, including internet explorer. Object.values and Object.entries methods are introduced in ES8, so their browser support is not as good as the Object.keys method. But, It's supported in all major browsers browser excluding Internet Explorer.
Browser | Chrome | Firefox | Mozila | Edge | Opera |
---|---|---|---|---|---|
Object.keys | Yes | Yes | Yes | Yes | Yes |
Object.values | Yes | Yes | Yes | Yes | Yes |
Object.entries | Yes | Yes | Yes | Yes | Yes |
Conclusion
- Object methods can help to convert objects to array javascript.
- Object.keys method helps to generate an array of object keys.
- Object.values method helps to generate an array of object values.
- Object.entries method helps to generate an array of object entries, i.e., the key-value pair.