Java Convert String to Date
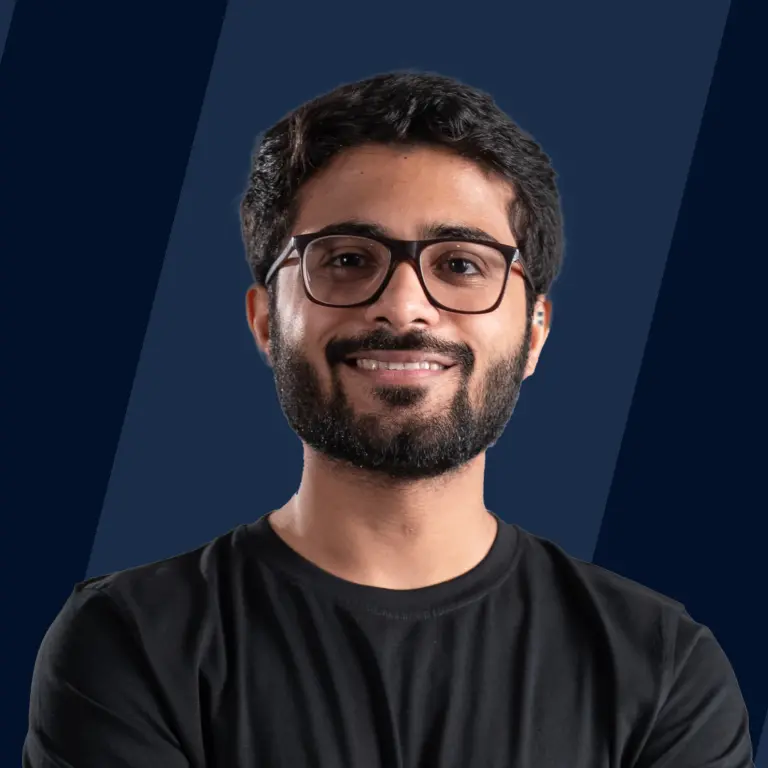
There are different scenario where we have to convert string to date in java.example- fetching any weather api as JsonObject and then manipulating the data. We can convert String to Date in Java using the parse() method of LocalDate, Instant, and SimpleDateFormat classes. To specify the date-time pattern used in the input string, we can use DateTimeFormatter and SimpleDateFormat classes.
Java String to Date Examples
When working with dates in Java, it's common to need to convert strings representing dates into Date objects. There are several ways to achieve this, including using the SimpleDateFormat class, the DateTimeFormatter class, and the Instant class. Let's explore each method:
- Simple Code Example to Convert String to Date in Java
- Using SimpleDateFormat Class
- Using DateTimeFormatter Class
- Using Instant Class
1. Simple Code Example to Convert String to Date in Java
Predefined formatter is the standard ISO_DATE format by the ISO that looks like yyyy-MM-dd. It is defined in java.time.format.DateTimeFormatter class in Java. Below are the steps that need to be followed to convert String to Date object by parsing the given input string using parse() method of LocalDate class in Java.
Steps for Conversion from String to Date:
- The input String holding the date should be in the pattern yyyy-MM-dd. For example: 2021-08-23.
- Create a LocalDate object with a name date.
- Convert the String to Date object using the LocalDate.parse() method
- In the arguments of parse() method, the first argument is the input string and the second argument is the DateTimeFormatter.ISO_DATE. This method takes the given input string in the standard ISO format and converts it to a date object.
- If the conversion is successful, print the date.
- If the String pattern is invalid, then IllegalArgumentException is thrown.
- If String is not converted successfully, then DateTimeParseException gets thrown.
Code:
Output:
Explanation:
- Import the classes LocalDate and DateTimeFormatter.
- Store input string in a string variable.
- Now use the parse method of LocalDate to parse the given input string and convert it into Date object, for the format here we use the predefined ISO_DATE format (yyyy-MM-dd) present in DateTimeFormatter class.
- Output the Date object so created.
2. Using SimpleDateFormat Class
In this method, we make use of the class java.text.SimpleDateFormat. This class works similar to the DateTimeFormatter, where we take an input string in date and specify the format/pattern of the input string for conversion. Below are the steps that need to be followed to perform the conversion using SimpleDateFormat class by parsing the input string with the parse() method of SimpleDateFormat class:
- Select the input date format and create a SimpleDateFormat object for the required format by specifying the pattern in the constructor object, take a look at the below examples to understand how it works:
- The input string of the date must match with the pattern selected in the SimpleDateFormat object.
- Create a Date object.
- Convert the String to Date object using the parse() method of the SimpleDateFormat class.
- In the arguments of parse() the first argument is the input string and the second argument is the SimpleDateFormat object created using a pattern.
- If the conversion is successful, print the date.
Let us see the above steps for conversion implemented in a simple Java Program.
Java Program To Convert String to Date Using SimpleDateFormat Class
Output:
Explanation:
- Store the input string into a string variable
- Now create a SimpleDateFormat object named format. With the same pattern as the input string. For example, in the above Java code the input string is “Wed, Aug 30 2021”, so the format pattern should be “E, MMM dd yyyy” as we can see specified in the argument while creating the format object.
- The pattern should match the input string otherwise an exception is thrown.
- Now, create a Date object date and parse the input string using the parse() method providing the arguments as the input string and format object created in Step 2.
- Output the date object to print the date.
3. Using DateTimeFormatter Class
In this method of conversion, we create the Date format instead of using the standard ISO format. The class used is java.time.format.DateTimeFormatter.
Steps to create the format:
- Select a format and create a DateTimeFormatter object for the required format using the method DateTimeFormatter.ofPattern() method.
Below examples are of some parseable date patterns that can be parsed using the parse() method of LocalDate class.
Eg:
Steps to perform the conversion:
- The input string holding the date must match with the pattern selected in the DateTimeFormatter object.
- Create a LocalDate object date.
- Convert the String to Date object using the LocalDate.parse() method
- In the arguments of parse() the first argument is the input string and the second argument is the DateTimeFormatter object specifying the format of the date.
- If the conversion is successful, print the date.
- If the String pattern is invalid, then IllegalArgumentException gets thrown.
- If String is not converted successfully, then DateTimeParseException gets thrown.
Code:
Output:
Explanation:
- Import the classes LocalDate and DateTimeFormatter.
- Store the input String in a string variable.
- Create an object format of DateTimeFormatter class with the input String date pattern using the ofPattern() method of the DateTimeFormatter class.
- Since the input string date is 30 August, 2021 the pattern will be d MMMM, yyyy. If the wrong pattern is given the input string cannot be parsed.
- Create a LocalDate object with name date.
- Parse the input string with LocalDate method parse(). In the second argument of the parse method mention the format object created above.
- Output the date object.
4. Using Instant Class
This method of conversion is similar to the Date object but gives slightly better accuracy in terms of displaying time. It gives nanosecond accuracy. Below are the steps that need to be followed to convert a string to date using java.time.Instance class by parsing the input string with the parse() method of Instant class.
Steps for Conversion using Instant class:
- Select an Input string that needs to be converted
- Create an empty Instant timestamp object and convert the input string using the method Instant.parse().
- If the conversion is successful, print the Instant timestamp object
- If the conversion is not successful, then DateTimeParseException is thrown
Code:
Output:
Explanation:
- Store the input string in timestamp format into a string variable.
- Create a Instant object named timestamp and parse the input string using the parse() method of the Instant class.
- Output the timestamp object.
Conclusion
- Java provides various classes like SimpleDateFormat, DateTimeFormatter, and Instant to parse strings into date objects, offering flexibility in handling different date formats.
- While SimpleDateFormat is a legacy class, still widely used and straightforward for simple date parsing tasks, the modern DateTimeFormatter class, introduced in Java 8, offers better thread-safety and additional functionalities.
- Regardless of the method used, proper exception handling is essential to manage cases where the input string does not match the expected format or cannot be parsed correctly. Both IllegalArgumentException and DateTimeParseException should be handled appropriately.
- Understanding the input date format and selecting an appropriate pattern for parsing is crucial. The pattern specified in SimpleDateFormat or DateTimeFormatter should match the format of the input string to avoid parsing errors.