Convert String to Date in JavaScript
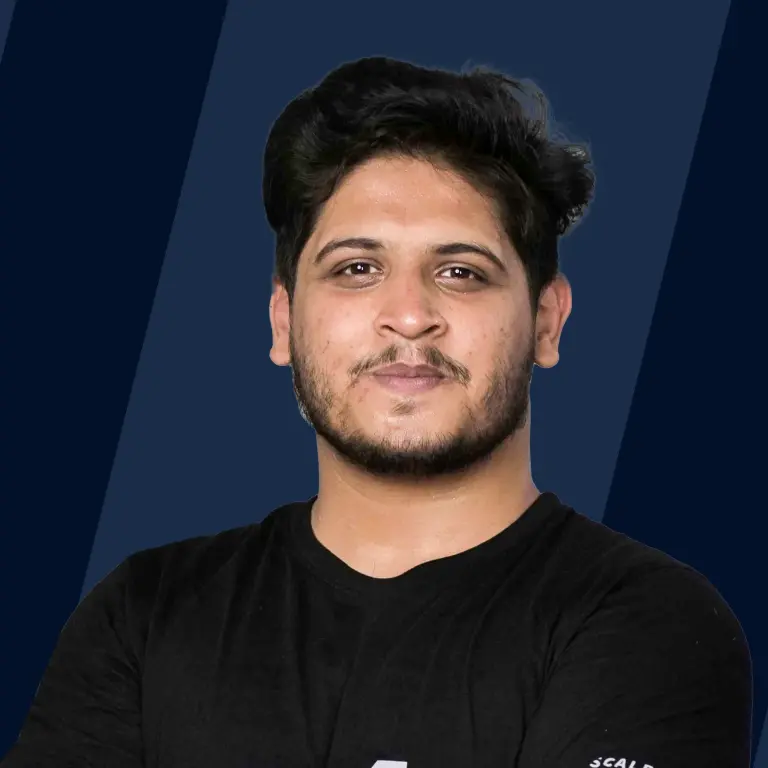
In JavaScript, converting a String to a Date involves using the Date class's constructor in two ways or its Parse method. Familiarity with the ISO 8601 format is essential. This article explains these methods, highlighting their importance in programming and web development, and aligns with JavaScript's date-handling capabilities.
ISO 8601 Calendar Date Extended Format
The ISO 8601 calendar date extended format is the standard format maintained by the International Organization for Standardization (ISO). The standard was first published in 1988, with the latest update in 2019. This standard provides a well-defined and unambiguous method of representing calendar dates and times in worldwide communications to avoid misinterpretations. The standard is as follows:
- Date is represented as yyyy-mm-dd
- Eg: 2022-05-19 would represent 19 May 2022
Convert String to Date in JavaScript Using the new Date() Function
There is a class in JavaScript called the Date class. You can use this function to get the current date and time of the local system. You can also use this class's constructor to convert a date value from string data type to Date data type. Let's look at this aspect briefly.
Syntax
Parameters
The parameter passed to the Date class's constructor should be a string representing a date in the ISO 8601 calendar date extended format.
Return Value
Retrun type: number
The return type is in the Date format. More precisely, the return value contains a number which is just the number of milliseconds since January 1, 1970, 00:00:00 UTC, and the date obtained by parsing the given string representation of the date. If the parameters are not in the appropriate format then this function returns "Invalid Date".
Example
Output:
The example given above shows how to convert a date from a String to a Date object using the Date class's constructor.
Convert String to Date in JavaScript using the Date.parse() Function
The Date class also provides us with another way to convert a date from string to Date format. The Date class has a static method called parse. You can use this method in the same way that you would use the Date class's constructor. You can pass the date in string format, and the function will return the date in the form of number which represents the number of milliseconds since January 1, 1970, 00:00:00 UTC.
Syntax
Parameters
The parameter passed to the Date class's parse function should also be a string representing a date in the ISO 8601 calendar date extended format.
Return value
Return type: number
It returns a number which is just the number of milliseconds since January 1, 1970, 00:00:00 UTC, and the date obtained by parsing the given string representation of the date. You can assign the returned value to any Date object and perform any operation. If the parameters are not in the appropriate format then this function returns NaN.
Example
Output:
The example given above shows how to convert a date from a String to a Date object using the Date class's static method : parse.
Split the string and convert it into Date Format
Up until now, we have seen two functions, but both functions ask that the input passed to them should be in the ISO 8061 extended format. But we can't always be sure that the input will always be of the ISO 8061 extended date format. So what to do when the input is not of the standard format. This is the solution to those situations.
We can again use the Date class to convert the String to the Date format. The Date class also has another constructor that takes parameterized input.
Syntax
Parameters
All the parameters should be of integer data type.
- Year value: The value should conform to the ISO 8061 standards. You should enter all 4 digits in the year. If you just entered 22 to mean 2022, it will be assumed as 1922.
- IndexOfMonth: The index value starts with 0. So you should subtract 1 from the month value. For example, May should be entered as 4 and not 5. So the accepted value range is 0 to 11.
- Day value:, Unlike the value for the month, you don't have to subtract 1 from the date. You can enter the date, just in the usual way. You can enter the date value from 1 to 31.
- Hours: The hour of the day in Railway time, enter 13 to denote 1:00 PM.
- minutes: The minutes past an hour. The value can be from 0 to 60.
- Seconds: The second’s value past a minute. Similar to the value of the minutes, the value of seconds can also be from 0 to 60.
Return Value
The return is an object of the Date class. The output follows all the same conditions as mentioned earlier for converting a date value from String to Date format using the Date class, Because both of them are constructors to the Date class and return an Object of the Date class.
Example
Output:
The example given above shows how to convert a date from a String to a Date object using the Date class's constructor when the input is not of the ISO standard.
Ready to dive into the world of web development? Join our JavaScript course and unlock the secrets of building interactive websites.
Converting Date Object to String Using the toDateString() Method
The above three methods we have discussed earlier are about converting a date value from String data type to that of Date data type. But we might also sometimes need the reverse. One common and more repetitive scenario is when we may face a situation where we need to store a date in a database. As already mentioned, Almost all of the databases store the date value as a String data type. So we will finally discuss one method that will help you achieve this -- Turn the date value from the Date data type to the String data type. The date class also has a method for this purpose.
Syntax
Parameter
The toDateString() method takes no parameter. It works on the object from which it was invoked.
Return Value
The return value is a string representing the date object in English. The format of the returned string is as follows:
- First three letters of the weekday name
- First three letters of the month's name
- Two-digit day of the month, padded on the left a zero if necessary
- Four-digit year (at least), padded on the left with zeros if necessary
Example
Output:
Supported Browsers
Almost all of today's modern browsers support all three methods discussed above.
Conclusion
- We can use the Date class to convert string to date in javascript data type.
- We can achieve this via 2 methods if you are sure that the value is of the ISO 8061 standard date extended format.
- We can either use the constructor of the Date class or use the parse method in the Date class.
- If we know that the input is not of the standard ISO 8061 date extended format, then we will have to manually split the date and pass the individual values as parameters to the Date class's constructor.
- All three methods discussed in the article are supported by almost all of today's modern browsers.