Convert String to Dictionary in Python
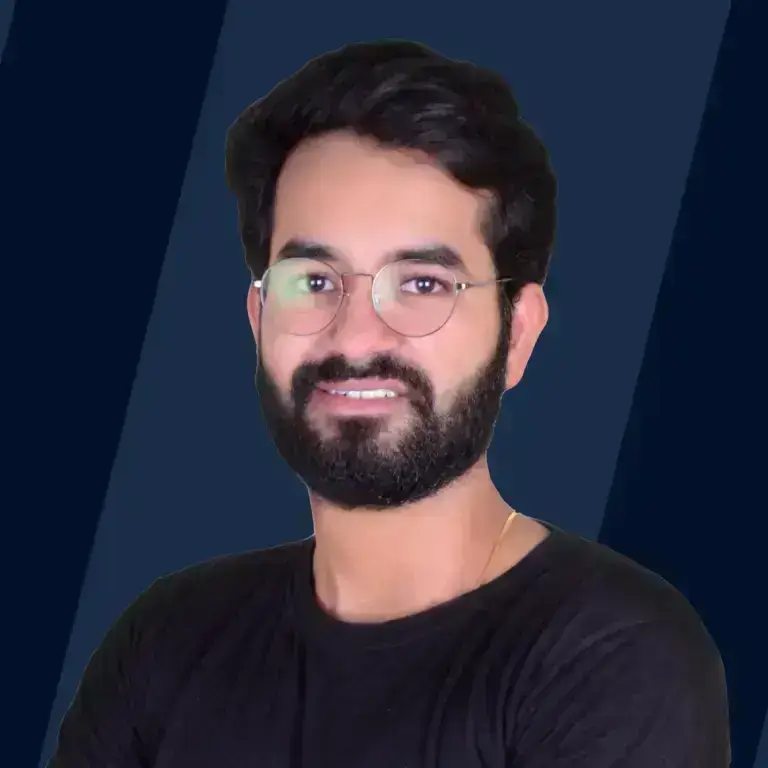
Overview
We have worked on different kinds of python problems, and one of the most frequent problems in python is to convert one data type to another. It is crucial to deal with while converting the data types because if there will a mistake, it might spoil our data. One such type of converting data type is converting the string into dictionaries. We have a detailed guide with different approaches to making this conversation effective and efficient. But before jumping on the methods, let us quickly recall the python string and dictionary in detail.
Dictionary: A dictionary is an unordered collection of data elements that is mutable. Python dictionary stores the data in the form of key-value pair.
String: Like other programming languages, Python strings are arrays of bytes representing Unicode characters. It is an immutable collection of data elements. In Python language there is no such data type of character data type, a single character is simply a string with a length of 1.
How to Convert String Into Dictionary in Python?
Before understanding the concept of converting string data type into a dictionary data type. We need to know about dictionary and string data types. A string is an immutable collection of data elements, whereas a dictionary is an unordered collection of data that is stored in key-value pairs.
Example: The below defines the string, and how a string is stored in python and shows some of the slicing operations of string which are pretty much similar to list slicing operations.
Code:
Output:
The below code explains the example of the dictionary, where a dictionary dictionary is defined which contains key-value pairs like (A,1), (B,2) and so on. This example also explains how the dictionary is implemented in python.
Code:
Output:
Converting one data type into another is an essential task because if there is one minor mistake in the code, our data might get corrupted and even spoiled, so we have a few different methods that can make our task(convert string to dictionary in Python) easy and fast.
Some of the methods we will see how to convert string to dictionary in Python are:
- json.load()
- ast.literal.eval()
- Using generator expression
Method 1: Using json.loads()
In this method, we will be using the JSON library which is available in python. We can easily convert python strings to the dictionary by using some inbuilt functions available in the JSON library of python. To be specific, we will be using the loads function that will convert our string into a dictionary. Before using this method, you have to import the JSON library in python using the import keyword.
Syntax:
Parameters:
- s: A string object that contain a JSON document to a Python object.
- object_hook: An optional parameter that will be called with the result of any object literal decoded.
- parse_float: It is an optional parameter that will be called with the string of every JSON float to be decoded.
- parse_int: It is an optional parameter that will be called with the string of every JSON int to be decoded.
- object_pairs_hook: It is an optional parameter that will be called with the result of any object literal decoded with an ordered list of pairs.
- Return Type: It will return a dictionary object that have been converted from the input object that is string.
Code:
Output:
Method 2: Using ast.literal.eval()
In this method, we will be using ast package that is available in python to convert our string into a dictionary. The ast.literal.eval() is an inbuilt python library function that efficiently converts the string to a dictionary. Before using this function, we will need to first import ast package, and then import the literal.eval() method that is available in the ast package.
Syntax:
Parameters:
- node or string: A string that contains dictionary-type data in it, so that the output will be a dictionary-like object.
- Return Type: It will return a dictionary type object.
Code:
Output:
Method 3: Using Generator Expression
Generators in Python are used to create iterators and return a traversal object. It helps in traversing all the items one at a time. In this method of converting python string into a dictionary, there are a few steps that we need to follow:
- Declare the string values paired with a hyphen or comma.
- Next, use the strip() in python and split() method of string manipulation in the for loop to get the dictionary-like format.
Within 2 steps, we have converted our python string to a python dictionary.
TIP: This method is not recommended as the conversion takes a lot of time to get the results.
- Syntax:
Parameters: It removes characters from both left and right based on the argument.
characters: It is an optional argument. It is the set of characters to remove as trailing characters.
Return type:
It returns a copy of the string with both leading and trailing characters stripped
- Syntax:
Parameters:
- separator: It is an optional argument, which specifies that the separator to use when splitting the string. By default, any whitespace is a separator.
- maxsplit: It is an optional argument, which specifies how many splits to do. Default value is -1, which is "all occurrences".
Return Type: It will return the splits a string into a list.
Code:
Output:
See Also
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.
Conclusion
- String and Dictionary are two of four major data structures in python. In this article, we have seen how to convert the string data type into a dictionary data type.
- There are 3 different types of methods that we have seen to convert a string into a dictionary i.e.,
- Method 1: Using json.loads()
- Method 2: Using ast.literal.eval()
- Method 3: Using generator expression