Python Counter Object
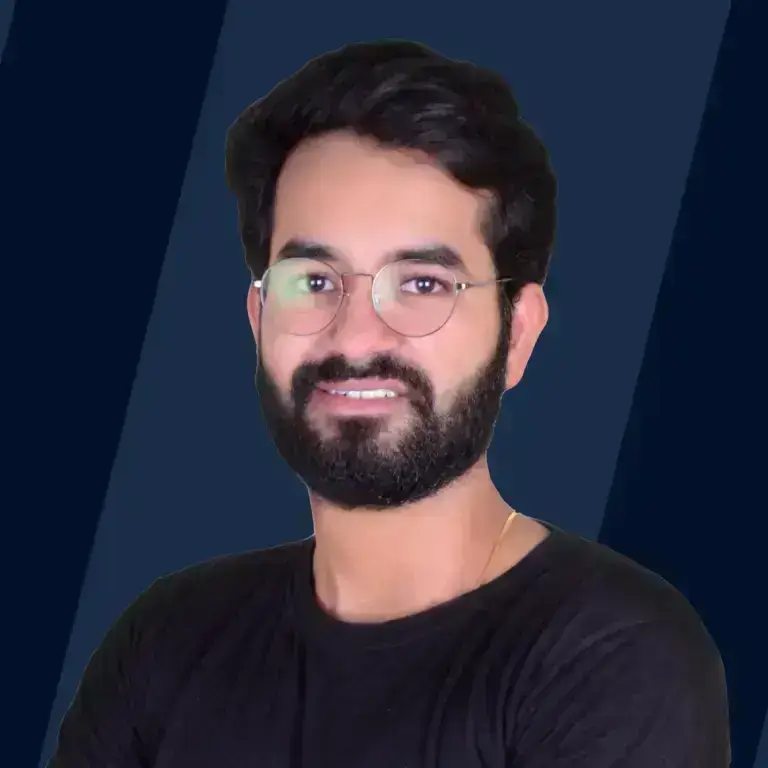
Overview
In Python, Counters are containers imported from the collections module, they are used to count elements (frequency of each element) of an iterable or remove zero and negative values in a dictionary.
We can assign, update, or even delete elements of a Counter object using its methods. We can also perform arithmetic operations on Counter objects.
What is Counter in Python?
While programming, you will be required to do some tasks that involve counting the elements in a container object, like counting word frequencies in a text or finding the most frequently used number in a list.
For such cases, Python has a container called Counter in its Collections module. The Counter is a sub-class of the dictionary class (dict). It is an unordered collection that consists of key-value pairs, like a hash table, where keys are mapped to the values, like in a dictionary) wherein, the elements will become keys and their counts will become values.
We will now learn about the syntax of Counter in Python to understand how it works.
Syntax
To use Counter in Python, we first have to import Counter from the collections module. Then we pass an iterable or keyword argument to the Counter() function.
Counter() function has a single parameter in case of passing an iterable; Counter(iterable) or multiple parameters in case of passing keyword arguments;
The iterable can be a list, string, tuple, or dictionary.
Let's understand this better using examples.
At first, we will have a look at its syntax when passing an iterable
Output
In the above example, we are passing an iterable (here, list) as an argument in the Counter, as you can see, it was a single argument because we cannot pass more than one iterable to the Counter() function.
Now we will have a look at its syntax when passing keyword arguments:
Output
In the above example, we are manually telling Counter() function the count using keyword arguments. It can be seen that multiple arguments can be passed to Counter() in the case of keyword arguments.
Why Use Counter in Python?
The main reasons for using Counter in Python are:
- The Counter holds the data in an unordered collection as key-value pairs, where items are keys and counts are values, which makes it easier (using Counter, we won't need to iterate over an iterable again and again to find its element's count) and less time taking to access the count of an item.
- The Counter can be used with iterables like list, string, tuple, and dictionaries.
- Arithmetic operations like addition, subtraction, intersection, and union can be easily performed on a Counter.
Creating Counter Object in Python
Before using the Counter Object, we will need to import Counter from the collections module.
Counter objects are created using the Counter() function, we then pass an iterable (list, tuple, string, or a dictionary), or keyword arguments (with values as counts), or it can be just left empty to create an empty Counter object.
Creating an empty Counter object:
Output
In the above example, we haven't passed any argument to the Counter() function to create an empty Counter object, which can be seen in the output.
This was just the creation of a counter object, but to initialize it as well, we will have to pass an iterable, or keyword arguments to it.
Initializing a Python Counter object
To initialize a Python Counter, we use the Counter() function to which we pass an iterable or keyword arguments.
Using Counter with Lists
In Python, lists are iterable objects that have a sequence of elements enclosed in square brackets "[]".
When a list is passed to the Counter, the elements in it will be converted into hash-table objects (keys are mapped to the values, like in a dictionary) wherein, the elements will become keys and their count will become values.
Let's look at an example for using a list in the Counter:
Output
In the above example, we are first importing Counter from the collections module. Then we are initializing a Python list and a Counter object using the Python list, and at last, we are printing the Counter. It can be seen in the list that "a" has occurred two times, "1" has occurred one time, and the tuple "(1, 2, 3)" has also occurred one time.
While using Counter, keep in mind that it cannot count unhashable objects like lists, sets, and dictionaries.
Using Counter with Dictionaries
A dictionary has key-value pairs as elements which are enclosed in curly brackets "{}" separated by commas ",".
On passing the dictionary to the Counter, its elements will become hash-table objects (key-value pairs), where the key will be the element and value will be its count.
Output
In the above example, we are initializing a dictionary and a Counter object using the dictionary and at last, we are printing the Counter.
It can be seen in the dictionary that the key "a" has the value "2" so its count is 2 in the counter, the key "b" has occurred two times, first its value was "1" then it changed to "3" (as dictionary can only have unique keys), so its count is 3 in the counter and the key "c" has occurred one time so its count is 1 in the Counter.
Using Counter with Keyword Arguments
Keyword arguments are key-value pairs (key=value), when passing them into Counter, the key becomes the element and its value becomes its count. They are used to manually tell Counter() the count of items.
Note that the value in the key-value pair should be an integer (as values are mapped to count, and count can't be anything but an integer), otherwise, the program would return an error.
Let's look at an example for using keyword arguments in the Counter:
Output
In the above example, we are passing keyword arguments to the Counter() function to manually tell Counter() the count of each item. In the end, we are printing the Counter object "my_counter".
Using Counter with Strings
In Python, strings are a sequence of characters. They are iterables.
On passing a string to the Counter() function, it will count the frequency of each character in the string.
Output
In the above example, we are initializing a string and a Counter object using the string. The Counter then counts the occurrence of every character (including blank spaces) in the string. According to the counter object, there are 3 "a" characters, 3 "b" characters, 2 blank spaces, and 2 "c" characters in the string.
Using Counter with Tuples
In Python, tuples are iterable collections of items enclosed in parentheses "()". They are similar to lists except for they are immutable (can't be modified), whereas lists are mutable (can be modified).
When a tuple is passed to the Counter, the items in it will be converted into keys, and their counts will be the values of the keys, which results in key-value pairs like in a dictionary or a hash-table object.
Let's take a look at an example for using a tuple in the Counter:
Output
In the above example, we are initializing a Python tuple and a Counter object using the same tuple, and at last, we are printing the Counter. It can be seen in the tuple that "z" has occurred two times, "x" has occurred one time, "1" has occurred one time, and the tuple "(1, 2, 3)" has also occurred one time.
Accessing Counts in Python Counter
To access the count of a particular item in a Counter object, we can simply use the item to refer to its count in the Counter object.
Syntax: When returning the item of a Counter object by using it as an index, we get the count; count = my_counter[item]
This can be understood better using an example:
Output
In the above example, we are using values as keys to access their counts in a counter. We have indexed the characters "a" and "b" in the Counter to access their counts respectively.
If we index a character that is not in the counter, its count will return as zero.
Let's take a look at an example for this:
Output
In the above example, we are using the value "x" to find its count in the Counter object, which is returning its count as 0 (instead of raising a KeyError), as it is not in the list passed to the Counter() function.
The elements() Method
The elements() method is used to access the elements in the Counter object in Python. It returns an iterator value for all the known elements in the Counter object.
Syntax: The elements() method is used with a Counter object to return all the elements of a Counter object in their frequencies; my_counter.elements().
We will understand this better with a proper demonstration:
Output
In the above example, we are using the elements() method to iterate over the counter object, and print all its elements in the number of their counts.
Working with elements() method on a Counter object with 0 and negative counts:
Output
In the above example, we have passed a dictionary to the Counter() function and then we are using the elements() method to iterate over the Counter object. There were elements with 0 and negative counts in the dictionary passed into the Counter, but as you can see in the output, it only has characters "a" and "c". That's because the elements() method only considers the elements with positive counts and ignores elements with 0 or negative counts.
Accessing the Most Common Values in Python Counter
In Python, to access the n most common values (values with the highest number of counts) in the Counter object, we use the most_common() method.
When we pass a number (n) to the most_common() method, it returns n most common values (the values with the n highest count/frequencies, n can be one or more than one), but when we call it without passing an argument, it returns all the elements (same as calling it with an argument with a value equal to the number of elements in the Counter) in the Counter object.
Syntax of most_common(): We use the most_common() method with a Counter object. The default parameter of most_common() is 1, which can be changed by passing any positive integer; my_counter.most_common() or my_counter.most_common(n)
Let's take a look at an example:
Output
In the above example, we are using the most_common() method to return the 3 (as we have passed "3" to the most_common() method) most common elements in the Counter object.
Reassigning Counts in Python Counter
In Python, Counters are mutable (can be modified), and we can reassign count values just by assigning a new value to the count of a particular value in the Counter object.
Syntax: The count in a Python counter is reassigned by simply assigning it to another integer; my_counter[item] = new_count.
Output
In the above example, we are reassigning a count value in the Counter object by assigning a new value to it. As you can see in the output, before reassigning, the count of character "a" was 3, but after reassigning, its count is now 10.
Updating a Python Counter
In Python, we can update a Counter object using the method update() on it. The update() method takes iterable or keyword arguments and then updates their count into the Counter object.
We can also use the update() method to populate an empty Python Counter.
Syntax: The update() method is used with a Counter object, and then an iterable or keyword arguments are passed to it; my_counter.update(list) or my_counter.update(key1=value1, key2=value2,...)
Let's look at an example for this:
Output
In the above example, we are initializing an empty Counter, to populate it using the update() method, we are then updating its values using the update() method.
Methods Available on Python Counter
These are some methods available on Python Counter:
Getting Count of Elements from a Python Counter
We can get the count of elements from a Python Counter using its values as keys, which returns that value's count.
Syntax: By returning the item of a Counter by using it as an index, we get the count; count = my_counter[item].
To get the count of elements from a Counter object, do as follows:
Output
In the above example, we are getting the count of elements by referring to the elements by their keys, returning their counts. Here, we are getting the count of each element using this method.
Setting Count of Elements in a Python Counter
We can set the count of elements in a Python Counter by assigning the element (referred to by the key) a value (count).
Syntax: A value is assigned to an item of Counter object; my_counter[item] = value.
To set the count of elements in a Counter object, do as follows:
Output
In the above example, we are setting the count of elements in the Counter object by assigning them values. Here, in the original Counter, the count of 'a' was 2, 'c' was 3, and 'e' was not present in the Counter, so its count was 0, but after assigning the count of 'a' to be 5, count of 'c' to be 2, and count of 'e' to be 1, we have modified the original Counter, and that can be seen in the output.
Deleting an Element from Python Counter
In Python, we can delete an element in the Counter object using del.
Syntax: del keyword is written before the Counter object's element; del my_counter[item].
It will be easier to understand by looking at an example:
Output
In the above example, we are deleting an element in the Counter object (my_counter) using del. The statement "del my_counter['b']" deletes the element "b" and its count from the Counter, which can be seen in the output.
Arithmetic Operations on Python Counter
When using Python Counters, we can use some arithmetic operations too, just like with numbers. However, only elements with a positive count are returned with these operations.
We can perform addition, subtraction, intersection, and union between two Counter Objects.
Addition of Counter Objects in Python
The addition of Counter objects returns a Counter object, with the total count of each corresponding item. If an item is not present in a counter object, it is understood that its count in it is 0. It keeps only positive counts.
Syntax: Counters are added using the "+" arithmetic operator; sum_counter = counter1 + counter2 + ...
Let's look at an example for adding Counter objects.
Output
In the above example, we are adding three Counter objects. The count of elements in the sum Counter is equal to the total frequency of them in the Counters being added. It can be seen that the count of element 'b' in counter1 is 4, in counter2 is 1, and in counter3 is '0', therefore the count of 'b' in the sum counter is 4+1+0=5.
Subtraction of Counter Objects in Python
The subtraction of counter objects returns a counter object with the count of elements in the first Counter after getting subtracted from its corresponding values in the following counters. It only keeps positive counts.
Syntax: The difference of counter objects is found using the "-" operator; difference_counter = counter1 - counter2 - ...
Let's look at an example for subtracting Counter objects.
Output
In the above example, we are subtracting counter2 from counter1. It can be seen that counter1 has an element 'b' of count 4, 'b' in counter2 has count 1, this is why the count of 'b' in the final Counter is 4 - 1 = 3. Similarly, the element 'c' in counter1 and counter2 has counts -3 and 4 respectively, and after subtracting them, we get -3 - 4 = -7, this is the reason of it getting ignored in the output, as Counter only keeps positive elements.
Union of Counter Objects in Python
The union of Counter objects will return a counter object with all the maximum values from the given counter objects. We can find the union of two or more counter objects. It keeps only the positive values.
Syntax: Union of counter objects is found using the "|" operator; union_counter = counter1 | counter2 | ...
Let's take a look at an example for finding the union of Counter objects.
Output
In the above example, we are finding the union of counter1 and counter2. It can be seen in the source code that the maximum count of 'a' among the given counters is 3, 'b' is 4, 'c' is 4, and 'd' is 1, all these elements with positive counts can be seen in the final Counter.
Intersection of Counter Objects in Python
The intersection of Counter objects will return a counter object with all the minimum values from the given counter objects. We can find the intersection of two or more counter objects. It keeps only the positive values.
Syntax: Intersection of counter objects is found using the "&" operator; intersection_counter = counter1 & counter2 & ...
Let's take a look at an example for finding the intersection of Counter objects.
Output
In the above example, we are finding the intersection of counter1 and counter2. It can be seen in the source code that the minimum count of 'a' among the given counters is 2, 'b' is 1, 'c' is -3, 'd' is 0. Among these, 'c' and 'd' are ignored as their minimum count among the given Counters is 0 or less than 0. Elements 'a' and 'b' are in the final Counter due to their positive counts.
Miscellaneous Operations on Python Counter
These are some operations we can perform on Python Counters:
Sum of Counts of a Python Counter Object
We can find the total sum of a counter object using the sum() function in Python.
Syntax: We pass the counter_object.values() to the sum() function; sum(counter_object.values()).
Let's take a look at an example:
Output
In the above example, we are finding the sum of total counts of a Counter object by using the sum() function on my_counter.values() (values here are all the counts of elements in the Counter). It can be seen that 'a' has a count of 5, 'b' of 1, 'c' of 4, and 'd' of -2, their total being 5+1+4+(-2) = 8, and that can be seen in the output.
Removing Zeroes and Negative Values from a Python Counter Object
We can remove zeroes and negative values from a Counter object by adding it to an empty Counter object. As we know, when we add two Counter objects, all the items with zero or negative counts get ignored, In this way, we can remove a Counter object's zero and negative counts by adding it to an empty Counter object.
Syntax for Removing Zeroes and Negative Values:
We add an empty Counter object to the original Counter object; my_counter += Counter().
Here's an example, to understand this better:
Output
In the above example, we are removing the zeroes and negative values from the Counter object using the "+" sign. It can be seen in the output, that elements 'b' with a count of 0 and 'd' with a count of -4 are removed and elements only with positive counts are present in the output.
Deleting all the Elements of a Python Counter Object
We can delete all elements of a Counter object using the clear() method. The Counter will then be empty.
Syntax of clear() method: We use the clear() method with a Counter object; Counter_object.clear().
Here's an example, to understand this better:
Output
In the above example, we are deleting all the elements of a Counter object using the clear() method. It can be seen in the output, that after deleting all its elements, only an empty Counter object is left.
Conclusion
- Counter in Python is used to count the number of occurrences of a value.
- It is initialized using the Counter() function.
- Counter objects can be used with lists, strings, tuples, dictionaries, and keyword arguments.
- We can perform arithmetic operations like addition, subtraction, union, and intersection on Counter objects.
- We can update a Counter object using the update() method, find the most common values in it using the most_common() method, delete an element in it using del, and delete all its elements using the clear() method.