Coupling in Java
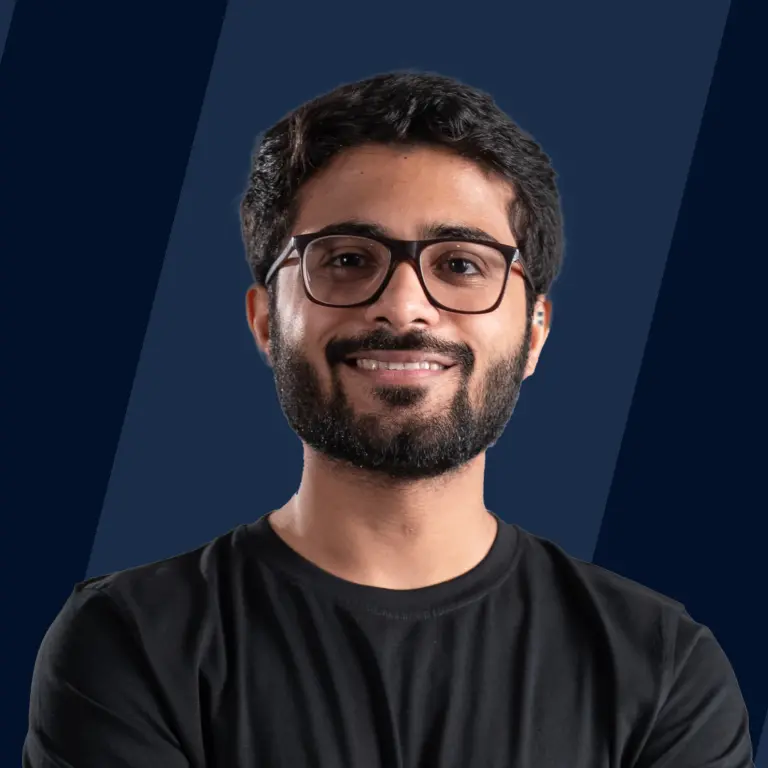
In the realm of Java software design, coupling plays a pivotal role in determining system quality. To craft modular, adaptable, and robust applications that stand the test of time, your code should have low coupling. But what exactly is coupling in Java? This article delves into the details of coupling, highlighting its impact on code maintainability and extensibility. It explains the types of coupling - from loose to tight and their consequences on the stability of software components. Let's dive in!
What is Coupling in Java?
Coupling in Java refers to the degree of interdependence between different modules or classes in a software system. It measures how closely classes or components are connected. In a well-designed software system, it's generally desirable to have low coupling, which means that classes or components are relatively independent of each other. This promotes modularity, reusability, and maintainability.
Consider the situation where we are creating a metadata collector application. The function of this application is to gather metadata on our behalf. It acquires metadata presented in XML configuration, subsequently transferring the acquired metadata to a CSV file. This initial strategy, as evident from the design, includes:
The responsibility of fetching, processing, and exporting data is managed by our module. Nevertheless, this design is not optimal, as it violates the single responsibility principle. To enhance our initial design, a separation of concerns is necessary. After this change, our design looks like:
Presently, our design has been divided into two distinct modules: XML Fetch and Export CSV. Simultaneously, the Metadata Collector Module relies on both of these modules. Although this design represents an improvement over our initial approach, it remains an ongoing refinement. In the upcoming segments, we will observe how we can further enhance our design by adhering to good coupling principles.
Types of Coupling in Java
There are two types of coupling in Java.
Loose Coupling in Java
Loose coupling in Java refers to designing classes and components in a way that minimizes their interdependence. In a loosely coupled system, classes or modules are independent to the extent that changes in one module do not require changes in other modules. This promotes modularity, ease of maintenance, and code reusability.
A loosely coupled design is achieved by:
- Defining clear and well-defined interfaces for communication between modules.
- Minimizing direct dependencies between modules.
- Avoiding tight relationships that can lead to ripple effects when changes are made.
Tight Coupling in Java
Tight coupling in Java refers to a situation where classes or modules are highly interdependent, meaning that changes in one module often require changes in other modules. It implies that the internal details of one class are tightly integrated with the internal details of another class. Tight coupling can lead to code that is difficult to maintain, as modifications in one part of the system can inadvertently affect other parts.
Tightly coupled designs often suffer from:
- Reduced modularity:
Changes in one module can have ripple effects across the application. - Difficulty in maintenance:
Making changes to one part of the system can result in unexpected issues in other parts. - Limited reusability:
Tightly coupled code is harder to reuse in different contexts.
Now let's look at a few examples to understand loose coupling in Java and tight coupling in Java.
Example - 1: Online Shopping Application
Consider building a simple online shopping application. You have two main modules: Cart and Payment. The Cart module manages the items selected by the user, and the Payment module handles the payment process. You want these modules to be loosely coupled.
Without Loose Coupling:
Code:
Explanation:
In this example, the Cart class directly creates an instance of Payment, which tightly couples the two modules. If the payment process changes, you might need to modify the Cart class.
With Loose Coupling:
Code:
Explanation:
In this example, an interface PaymentProcessor is defined, representing the payment functionality. The Cart class now relies on this interface rather than a concrete Payment class. During initialization, you inject an instance of Payment (which implements PaymentProcessor) into the Cart. This decouples the Cart and Payment modules, as changes in the payment process won't impact the Cart class directly.
Example - 2: Messaging System
Consider a messaging application where users can send messages to each other. You want to implement a notification system for new messages.
Without Loose Coupling:
Code:
Explanation:
In this scenario, the MessageService directly creates an instance of NotificationService, leading to tight coupling between message sending and notification.
With Loose Coupling:
Code:
Explanation:
In this design, the Notifier interface is introduced to represent notification functionality. The MessageService class relies on this interface, allowing you to easily swap out the notification service implementation without impacting the message-sending process.
Example - 3: Customer Management Application
Consider a scenario where you're building a customer management application. You've two classes: Customer and Order. The Order class needs information from the Customer class to process orders.
Tightly Coupled Example:
Code:
In this example, the Order class creates a new instance of the Customer class within its constructor. This creates a tight coupling between the two classes. If the structure of the Customer class changes, the Order class might also need modification.
Code Example with Loosely Coupled Design:
Code:
Explanation:
In this design, the Order class relies on a Customer instance passed to it during construction. This is a step toward loose coupling. By injecting the Customer instance, you separate the creation of Customer objects from the Order class, making the Order class less dependent on the specific implementation details of the Customer class.
By reducing tight coupling, you create a design where classes have well-defined responsibilities and changes to one class have fewer unintended consequences on other classes. This enhances maintainability, reusability, and flexibility in your codebase.
Loose Coupling vs. Tight Coupling in Java
Here are the key differences between Loose Coupling in Java and Tight Coupling in Java.
Modularity:
Loose coupling in Java enforces well-defined responsibilities for components, enhancing system understanding and ease of maintenance. In contrast, tight coupling in Java can lead to a lack of clear boundaries and increased complexity.
Flexibility:
Loose coupling in Java permits efficient adaptation to changes by containing their impact, while tight coupling in Java results in changes often propagating across multiple modules, making the system rigid and prone to errors.
Code Reusability:
Loosely coupled components are easily reused in various scenarios due to minimized dependencies, whereas tightly coupled components are less portable and adaptable.
Testing:
Loose coupling in Java facilitates independent unit testing, contributing to better software quality, while tight coupling in Java complicates testing by requiring consideration of interconnected components.
Dependency Management:
Loose coupling in Java minimizes dependencies between modules, simplifying maintenance and fostering parallel development. Conversely, tight coupling leads to strong interdependencies, making maintenance and concurrent work more challenging.
Maintainability:
Loose coupling in Java enhances code maintainability by reducing unintended side effects, whereas tight coupling in Java results in complex interactions that can lead to difficulties in tracking and resolving issues.
Which is Better?
Loose coupling in Java is superior to tight coupling due to its numerous advantages. Loose coupling promotes modularity by defining clear responsibilities for components, hence making maintenance and understanding easier.
Code reusability thrives in loosely coupled systems, ensuring components can be reused across diverse contexts. Testing is streamlined as independent unit testing becomes feasible, resulting in higher software quality. Additionally, loose coupling in Java simplifies dependency management, supports parallel development, and contributes to enhanced maintainability.
Tight coupling in Java can lead to security vulnerabilities by increasing the attack surface and making it easier for security issues in one component to cascade. When components are tightly interdependent, vulnerabilities or breaches in one component can potentially propagate to connected components, increasing security incidents. In contrast, loose coupling enhances security by isolating components and reducing the direct dependencies between them.
Java frameworks like Spring and Hibernate also promote loose coupling. Spring's Dependency Injection (DI) and Aspect-Oriented Programming (AOP) separate concerns and facilitate the injection of dependencies, reducing tight coupling and enabling the development of modular components. Hibernate abstracts database interactions, isolating the application from specific database implementations.
Conclusion
- Coupling in Java refers to the level of interdependence between classes or components within a software system. Coupling in Java is of two types: Tight coupling and Loose coupling.
- Loose coupling in Java indicates a design where classes or modules have minimal interdependence, allowing changes in one part to have fewer ripple effects on others, promoting modularity and maintainability.
- Tight coupling in Java refers to strong interdependence between classes or modules, where changes in one part often require modifications in others, making the system less flexible and maintainable.
- Loose coupling in Java aligns with fundamental design principles like the Single Responsibility Principle, Separation of Concerns, and Open/Closed Principle, fostering better overall architecture.
- Loose coupling in Java is crucial for building high-quality Java software. Its benefits, such as modularity, flexibility, and code reusability, lead to more maintainable, adaptable, and reliable systems.
- The advantages of loose coupling in Java in enabling parallel development and team collaboration contrast sharply with tight coupling's tendency to hinder concurrent work due to intricate interdependencies.
FAQs
Q. What is tight coupling in Java?
A. Tight coupling in Java refers to strong interdependence between classes or modules, where changes in one part often require modifications in others, making the system less flexible and maintainable.
Q. What is loose coupling in Java?
A. Loose coupling in Java indicates a design where classes or modules have minimal interdependence, allowing changes in one part to have fewer ripple effects on others, promoting modularity, testability, and maintainability.
Q. What strategies can reduce coupling in Java applications?
A. To reduce coupling, strategies include defining clear interfaces, encapsulating implementation details, using dependency injection, adhering to design patterns, and employing the principles of separation of concerns and single responsibility.
Q. How does loose coupling in Java improve software design?
A. Loose coupling enhances software by promoting modularity, flexibility, code reusability, ease of testing, and better maintainability, which collectively lead to more robust and adaptable applications.