Cout in C++
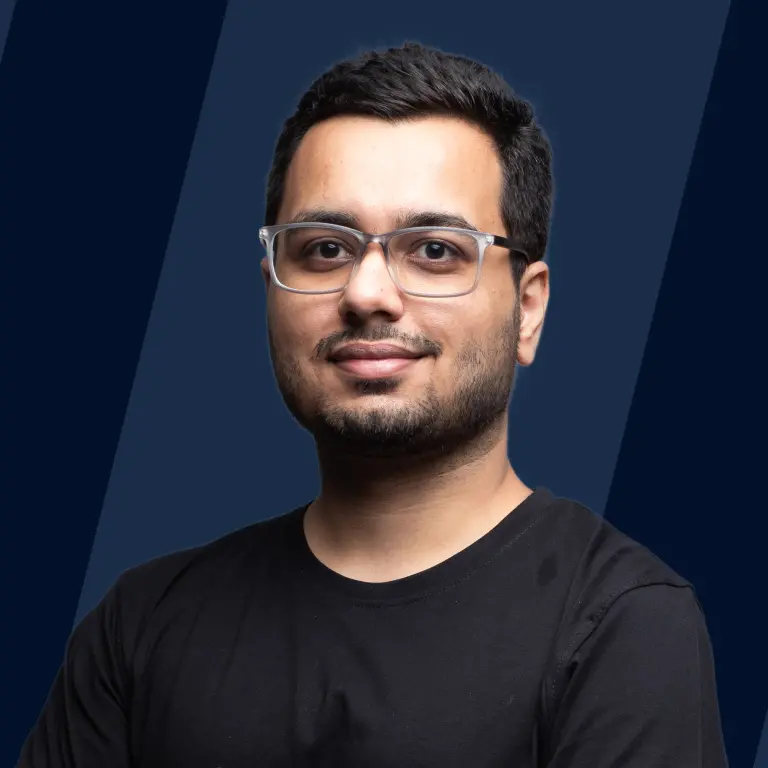
Overview
cout in C++ is used to display output to the screen. The 'c' in cout stands for character, and 'out' stands for output. Thus cout refers to character output. We use the insertion operator along with cout to output formatted data. cout in C++ is defined in the iostream header file. To use cout, we must include namespace std in our program. Without it, we would have to write std::cout instead of cout in our code. In this article, we will cover cout in further detail.
Syntax of Cout in C++
The syntax of cout in C++ is as follows:
Here << is the insertion operator used in C++ to print the output. It is followed by the output that is to be printed on the screen. This output might be some variable, constant, array element, or element of containers such as map, set, etc.
Exceptions For Cout in C++
Using cout, we can only give the output of primitive data types. We will get an exception if we try to pass some non-primitive data types such as structures, classes, etc. To avoid the error, we have to overload << operator.
Example
In this example, we will demonstrate the use of C++ by assigning a value to a variable and printing that variable.
Output:
We named our variable a in the above program and assigned it the value 5. We printed the variable to the screen by using cout. Thus the value of the variable, i.e. 5 appeared in the output.
What is Cout in C++
To understand cout in-depth, we'll have to understand what streams are.
In C++, streams refer to a sequence of bytes. They flow in and out of the program. The stream classes handle the various input and output operations in C++. These classes are stored in the <iostream> header file.
Streams can be of two types - input and output streams. The input stream is handled by the stream class, and the output stream is handled by the ostream class. In the output stream, data bytes flow from the program to the device screen.
ios class is the base class for both the istream and ostream classes. A cout object is an object of the ostream class in C++.
As discussed earlier, cout is used to display the output to the screen, i.e., the standard output device. Since the ostream class is a part of the iostream header file, cout also belongs to this same library. Hence we have to include the isotream header file in our program whenever we have to use the cout object.
cout can be used with both formatted and unformatted data. Formatted data is data in which bytes are converted into some data type, such as int, float, etc., whereas unformatted data is one in which bytes remain unconverted. For using cout with formatted data, we use the insertion operator (<<) for using cout with unformatted data member functions (e.g. - put()) are used.
Let us now see how we can make our output more presentable. We use manipulators, along with cout, to format our output. Let us go through the most commonly used ones:
- setprecision: Used to set the precision for decimal/float values.
- setw: Used to set the field width on output operations.
- setfill : Used to fill the stream with a specified character.
All of the above manipulators are included in the <iomanip> header file, so be sure to include it in your program if you use them.
More Examples of Cout in C++
Let us go through other examples demonstrating how we use cout in C++.
Program 1: Write a Program to Print All the Elements of an Array
Input:
Output:
Explanation :
We take input from an array of size n and print its elements to the screen.
Program 2: Write a Program to Depict the Usage of cout.precision()
Output:
Explanation:
cout.precision(int N) sets the decimal precision to N as depicted in the above program.
Program 3: Write a Program to Depict the Usage of cout.write()
Input:
Output:
Explanation:
cout. write(char arr, int N) prints the first N elements of the character array. This is depicted in the above program.
Program 4: Write a Program to Depict Multiple Insertion Operators
Input:
Output:
Explanation:
We can use more than one insertion operator to print multiple outputs on the screen. This is known as cascading of insertion operators.
Program 5: Write a Program to Depict the Usage of cout.put()
Output:
Explanation:
cout. put(c) prints the character stored in character variable c. This is depicted in the above program.
Conclusion
- cout is used to display output to the screen. It is an object of the class ostream and is defined in the iostream header file.
- For cout with formatted data, we use insertion operators (<<). In the case of unformatted data, we use member functions such as put() with cout.
- cout is also used with other member functions such as cout. precision(), cout. write() etc.
- To format our output and make it more presentable, manipulators such as set precision, setw, etc., are used with cout.
See Also:
You can also refer to the following topics: