C++ Accumulate
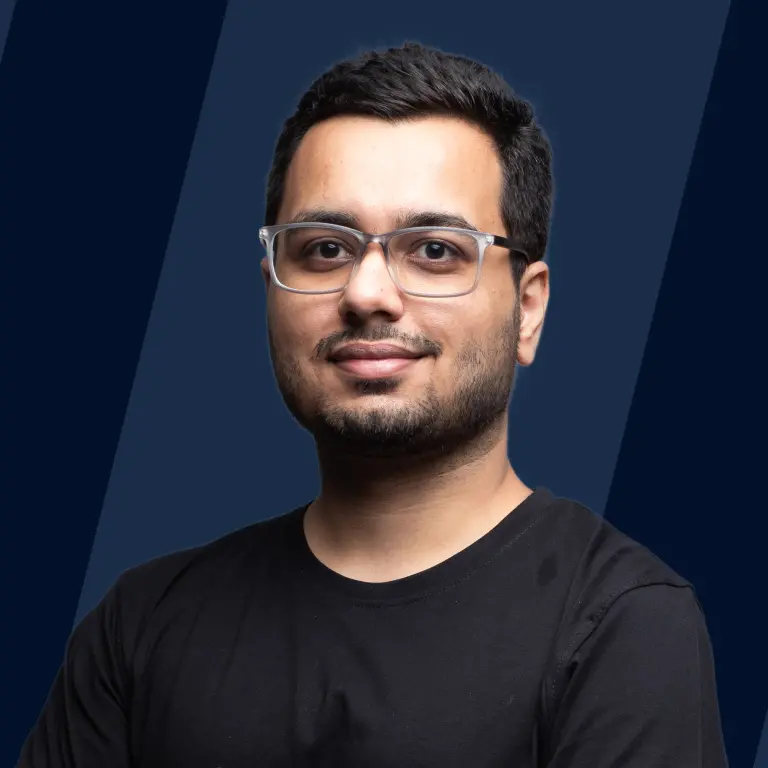
Overview
The C++ programming language provides useful built-in functions to ease our work as programmers. One such function is std::accumulate(), defined inside the standard numeric header file and generally used to calculate the sum of a wide range of different numerical values.
Use-cases of accumulate() function
The C++ accumulate() function calculates the sum of numerical values under a certain range. It can also be used to effectively iterate and compute the sum of an entire array or a vector with ease.
Syntax of C++ accumulate() function
The general syntax of c++ accumulate() function is as follows
But when used with the user-defined binary predicate, the syntax becomes
Parameters of C++ accumulate() function
The accumulate() function in c++ generally accepts three compulsory parameters, named
- startRange - specifies the position in the series from which we want the operation to start
- endRange - specifies the position in the series up to which we want to perform the specific operation
- initialValue - the initial value is given to the accumulate() function. For example - in the case of calculating the sum, we will pass initialValue as 0 because that is the natural additive identity for additions, or if we want, we can also give some else value to it. Then the addition process will be operated over that value as the start.
There is an optional parameter to the accumulate() function, where we can specify the type of operation we want to perform. The operation must always be separate from addition. We can do multiplication, division, or anything we want. The fourth parameter accepts a function that tells what operation to perform to the accumulate() function. We can create any custom function we want and can pass that.
The syntax of the accumulate() function with additional parameters is as follows.
Return Value of C++ accumulate() function
The accumulate() function in C++ returns the accumulated value of the specified range passed as the argument. It depends on the fourth parameter, the function we pass to instruct the function, and the work it has to perform, be it addition, multiplication, or anything as per the function definition.
If nothing is specified, it generally returns the numerical sum of the specified range in the case of arrays or vectors.
Exceptions of C++ accumulate() function
The C++ accumulate() function may show undefined behavior upon calling it with invalid arguments.
How does C++ accumulate() function works?
The C++ accumulate() function takes a range of values as input along with the initial value and performs operations on them. The default operation in case nothing is specified, the function performs addition and returns the added sum.
However, we can call the C++ accumulate() function with the additional fourth parameter and specify explicitly the operation type we want the function to perform.
Accumulate function with Predicates:
Predicate means a function returning a boolean value. Followed by binary predicate means a function that takes 2 arguments (hence binary) and returns a boolean value.
Let's understand this with the help of an example.
With Built-In binary predicate
In this example, we will calculate the product of a vector using a built-in binary predicate.
Program to calculate the product of a vector using accumulate() function
Output
With Custom binary predicate
In the below example, we will be printing a pattern on a vector series with the help of a user-defined binary predicate.
Program to print a pattern on a series of numbers using accumulate() function
Output
Examples of C++ accumulate() function
Let's understand more about accumulate() functions with the help of certain examples.
1. Calculating the Sum of a Vector Series using the accumulate() Function in C++
In the below example, we will be calculating the sum of a vector series using the accumulate() function in C++
Output
2. Calculating the Product of a Vector Series with Varying Initial Values
In the example below, we will calculate the product of a set of numbers by varying the initial values passed into the accumulate() function, and we will see how the output changes.
Output
3. Printing String Patterns using accumulate() Function in C++
In the example below, we will print some string patterns using the accumulate() function in C++.
Output
Conclusion
- The accumulate() is a standard library function in C++ language that is primarily used to calculate the sum of values over a specified range
- Syntax : accumulate(startRange, endRange, initialValue);
- It takes three compulsory parameters: the position in the series from where we want to start computing the sum, the position till which we want to calculate, and the initial value to be used as the base of the sum.
- It also takes an optional fourth parameter in which we can specify the type of operation we want the accumulate() function to perform.
- C++ accumulate() function is not only limited to calculating the sum of a series, but we can also use it to calculate the product of a series, inserting any specific pattern into the values of the given series, etc.
- The function usually returns the sum of the values of the specified range. Still, if we explicitly have mentioned what we want it to perform using its fourth parameter, then it will return the value concerning that specific operation.