C++ Program to Make a Simple Calculator
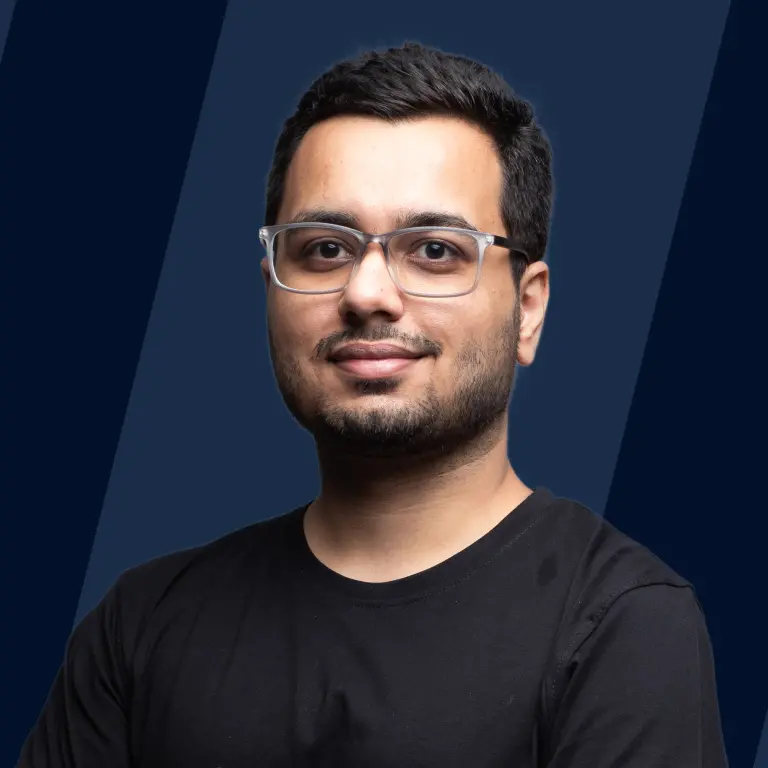
Overview
Making a basic calculator in C++ is a great place to start for new programmers. This project entails applying core C++ ideas to basic arithmetic operations such as addition, subtraction, multiplication, and division. We develop a user-friendly interface for input and display using variables, functions, and conditional expressions. A clear step-by-step structure and error management ensures a strong program. This calculator project serves as a basic exercise, allowing students to comprehend important C++ ideas, making it an excellent starting point for those new to programming.
Simple Calculator Program Using Switch Statement
Simplicity is frequently equated with effectiveness and practicality in the world of programming. When writing a basic calculator program in C++, a switch statement provides a simple and elegant solution. This section will lead you through the stages of creating a simple calculator program capable of doing fundamental arithmetic operations such as addition, subtraction, multiplication, and division.
Before you begin, have a C++ compiler installed on your machine. You can use well-known ones such as GCC or Visual C++.
Code:
Output:
Explanation:
- Variables are declared for the operation, two integers, and the outcome.
- Users enter the operation as well as two numbers.
- The switch statement examines the action and calculates the result.
- To handle division by zero and incorrect operators, error handling is implemented.
Validating user input is crucial in a C++ program, especially in a calculator application, to ensure the program operates correctly, remains robust, and avoids crashes due to unexpected input. Input validation helps maintain data integrity and provides a better user experience.
To validate user input:
- Data Type Check:
Ensure the input matches the expected data type (e.g., numeric). You can use functions like isdigit() for character inputs or cin.fail() for numeric inputs. - Range Check:
Verify if the input falls within acceptable ranges, like ensuring a denominator is not zero in division. - Error Handling:
Implement error handling to gracefully handle invalid inputs. For instance, when encountering non-numeric input, you can display an error message and request input again.
Example:
You have effectively used C++'s switch statement by creating this straightforward calculator program. It's an essential part of any C++ programmer's toolset since it's a vital tool for designing user-friendly and efficient programs. Feel free to extend this program to add extra functions and improve your coding abilities.
Calculator Program Using Function
In the world of programming, mastering the fundamentals is crucial. One such fundamental is using functions, which allow you to organize your code into reusable blocks, making it easier to read and maintain. This section will explore how to create a simple calculator program in C++ using functions.
Functions are like mini-programs within your main program. They take inputs (arguments), perform specific tasks, and return outputs. Our calculator program will define addition, subtraction, multiplication, and division operations functions.
Let's start by declaring our functions:
With our functions defined, we can now use them in our main program to perform calculations:
Output:
Breaking down our calculator's functionality into functions makes the code more modular and easier to understand. Functions in C++ allow for code reuse and maintainability, making them an essential tool in any programmer's toolkit. With this simple example, you're on your way to mastering C++ functions and building more complex programs in the future.
Calculator Program Using do-while Loop
In the world of programming, efficiency and user-friendliness are paramount. One way to achieve these goals is by using loops to control the flow of your code. In this article, we'll explore how to create a simple calculator program in C++ that utilizes the versatile do-while loop. This loop structure allows us to repeatedly perform calculations until the user decides to exit the program, making it an excellent choice for interactive applications.
Code:
Let's dive into the code. We'll create a menu-driven calculator that enables users to perform basic arithmetic operations: addition, subtraction, multiplication, and division. We'll use a do-while loop to keep the program running until the user chooses to exit.
Output:
Explanation:
In this C++ program, we've used the do-while loop to construct an interactive calculator. Users can repeat computations until they decide to quit the program. Understanding loop patterns like do-while is critical for developing user-friendly and efficient computer programs. Please modify and build on this code to meet your requirements.
Conclusion
- Creating a basic calculator program in C++ demonstrates the language's usefulness and adaptability. C++ enables efficient code execution, making it an ideal choice for developing such applications.
- The calculator application demonstrates the significance of building user-friendly interfaces in software development. Developers may improve the overall user experience by offering a clear and straightforward user interface.
- The modular architecture of the program, with distinct routines for each operation, emphasizes the need for code modularity and reusability. This method makes maintenance and future improvements easier.
- To ensure the calculator's dependability, input validation procedures must be included. Developers can prevent mistakes and improve the program's resilience by verifying user inputs.
- Despite its simplicity, this program is a basis for more advanced calculator applications. Developers can build upon this codebase to create advanced calculators with scientific, financial, or engineering capabilities.