What is the Use of the C++ Continue Statement?
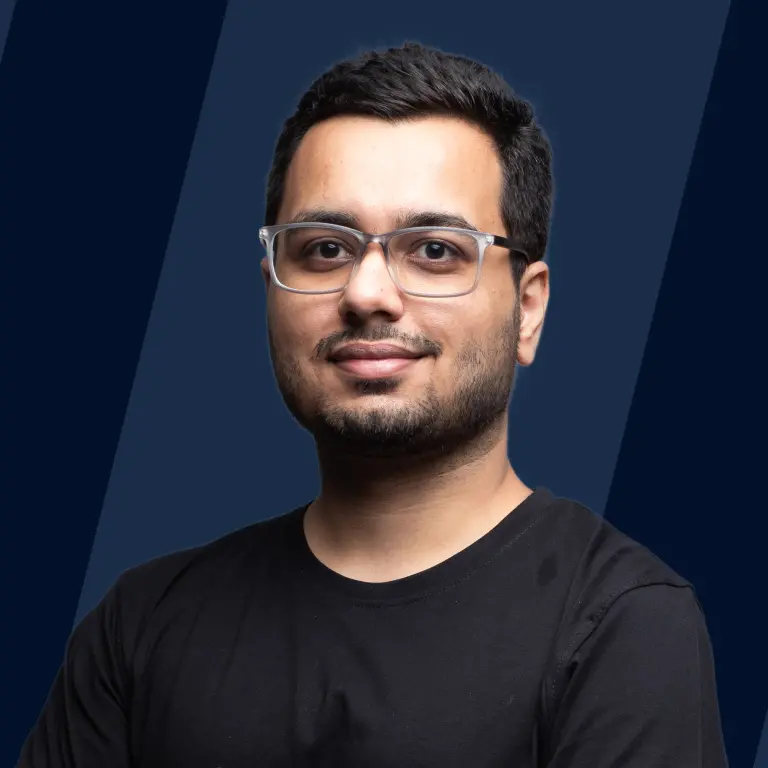
Continue is a loop control statement in C++, and the continue statement forces the program to execute the next iteration of the loop. The continue statement skips the current iteration of the loop and passes the program control to the loop condition. Using the continue keyword inside the loop forces the program to run the next iteration of the program.
C++ Continue Statement
Continue keywords are used inside loop conditions in C++ to skip the program's current iteration, and the program control reaches to check the looping condition. The continue statement passes the control to the nearest conditional test of the loop, where the program checks if the looping condition is satisfied or if there are iterations left.
Syntax of C++ Continue Statement
The syntax of a continue statement in C++ is:
In the above syntax, we placed the continue keyword inside the while block, but the continue keyword can be used with while, do-while, or for loops.
Example of C++ Continue Statement
Let us see an example where we want to print all the numbers between ranges but skip all the divisible numbers by six. We need to solve this problem only using one loop statement.
Output
Here, we use a for loop that starts with the lower limit l and runs through all the numbers until r. When we encounter a number divisible by six, we use the continue statement to skip the iteration because the program control reaches to check the for loop condition. This way, we can print all the numbers between l to r that is not divisible by six.
Uses of C++ Continue Statement
With the continue statement in C++, any remaining statement in the iteration is not executed. This way continue statement is used to bypass the code section inside the loop that follows it and begins the next iteration of the loop.
Continue statements are helpful when the condition or the result we require from the iteration is found, and we don't require commands following it to be executed. In such conditions, we use the continue statement to skip to the next iteration.
Working of C++ Continue Statement
The continue statement skips a particular iteration in the loop and does not stop the complete execution of the loop. The continue statement in C++ only skips the current iteration and continues with the next iteration.
Using the flow diagram, we can understand how the continue statement is in C++. Here, the execution for the iteration is skipped when the looping condition is met inside the loop body. If the condition is not met, the execution continues like a standard loop. The following image demonstrated the same thing with the actual while and for loop in C++.
In the above image, we can see that when the condition in the if block satisfies because of the continue keyword, the program execution skips to the beginning of the loop (both the while and for loop). The looping condition is rechecked, and if the condition still holds, the program execution goes inside the loop.
Examples of C++ Continue Statement
Now that we know the syntax and how the continue statement works in C++, let us see examples of how we can use the continue statement in C++.
C++ Continue with "for" Loop
If we use the continue statement in C++, the current iteration is skipped, and the program control reaches the update expression for a loop.
Output
In the abovementioned program, we use the for loop to print all the numbers between zero and four, except the number three.
Here is the condition:
This means that when the value of i equals 3, the continue statement skips the current loop iteration and starts the next iteration. Because the value of i is not equal to 3 for all the iterations, the continue statement is not executed, and the value of i is printed.
C++ Continue with While Loop
In the case of the while loop, the continue statement skips the current iteration, and the program control reaches back to the while condition.
Let us see a program where we print an extra output for all the odd numbers from 1 to 4.
Output
In the above example, we use a while loop to iterate all the numbers from 1 to 4. When the value of i is even, we use the continue statement to skip the loop condition to the next value. This is why we do not see the Output after the continue statement for all the even values of i.
We can use a similar approach to use continue statements in the program to debug the programs to find what conditions inside the loop cause the unrequired result.
C++ Continue with Nested Loop
When the continue statement is used inside the nested loops, it only skips the current iteration of the inner loop. To understand this, take a loop at the example below.
Output
When the continue statement executes inside the second statement, the inner loop is skipped, so we don't see i = 1 and j = 2 or i = 2 and j = 2 in the program output.
C++ Continue Statement with Two Different Types of Loops
Nested Loops
This case is called a nested loop when more than one loop is used inside another. When the continue statement is used inside nested loops, the nearest loop iteration is skipped. Let's look at an example to understand this case.
Output
In this example, we print the sum from 0 to i for each for loop iteration. We are using a continue statement whenever i is even. This skips all the even values in the for loop.
Infinite Loops
When we work with loops and continue statements, we should ensure that we are not creating a condition that will lead to the loop running indefinitely. For example, take a look at the example mentioned below:
Output
When the above program executes, the program runs indefinitely and never terminates because we are not incrementing the variable i inside the if block where we wrote the continue statement. This happens because as soon as the values of i become three, the program control reaches back to the beginning of the loop, ending in an infinite loop.
FAQs
Q: How is a "Continue" Statement Different from "break" statements in C++?
A: It is easy to confuse the break and continue statements because both are used in a loop. Let us see the difference between C++ break and continue statements.
break statement
- Break statement in C++ terminates the loop.
- Break statement can be used with both the loops and the switch statement.
- The control exists in the loop statement when the break statement is used.
- Break is used to break loop execution when a condition is met.|Continue is used to skip loop execution when a condition is met.
continue statement
- Continue statement skips the current iteration of the loop and doesn't terminates the loop.
- The continue statements can only be used with loops.
- The program control in case of a continue statement stays within the loop.
Related Articles
Now that you know about the continue statement in C++, you should read more about Jump statement in C++.
Conclusion
- Continue statements are used inside loop conditions in C++ to pass control to condition test, skip, and move to the next iteration of the loop.
- Continue statement passes the control to the nearest conditional test in while or do-while loop, or the condition check of for loop.
- The continue statement skips a particular iteration in the loop and does not stop the complete execution of the loop.
- The continue statement can help debug the program to find errors in the loop conditions.
- When the continue statement is used inside the nested loops, it only skips the current iteration of the inner loop.