What is an Extern in C++?
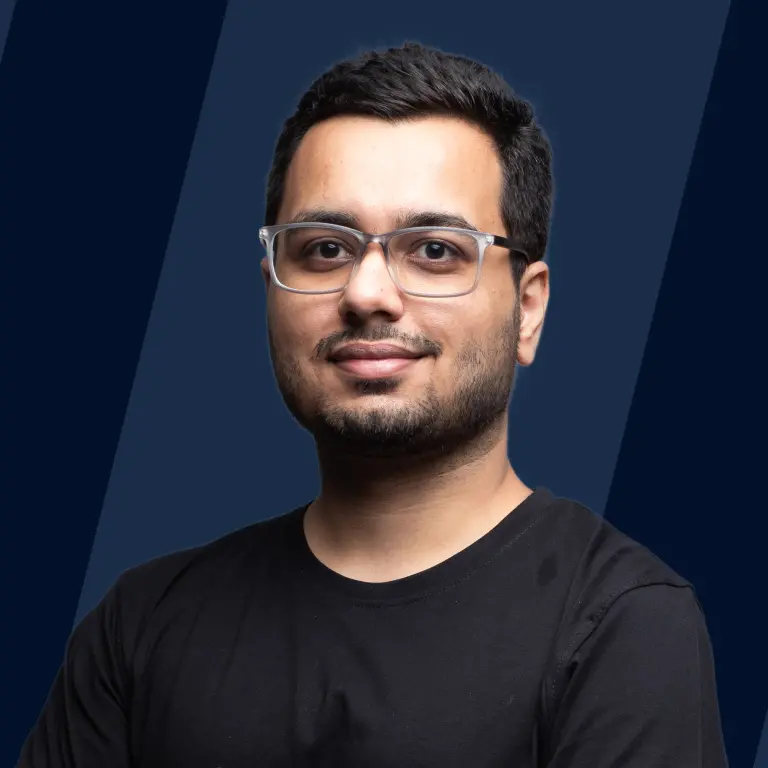
The extern keyword in C++ is used to declare a global variable or function which can be accessed from any part of the program or from other files included in the program's header. The variables or functions declared with the extern keyword will have a larger scope than normally declared variables. Those variables can be accessed from any part of the program.
It is important to note that the C++ extern keyword can only declare a variable and can't define it.
Declaring a variable implies that the variable is introduced to the compiler. Currently, no space is allocated for the variable, whereas defining a variable is allocating space for the variable.
The variables declared using the extern keyword should also be defined.
The syntax to declare a variable with the extern keyword in C++ is,
The data_type represents the data type of the variable named variable_name.
The variable_name has to be defined to get the required space allocated for the variable after declaring with the normal syntax,
The extern variable can also be defined and initialized when declaring the variable; in such cases, no separate definition is required for the variable. The following syntax is used to define and initialize the variable at the time of declaration,
The above property is not possible in C programming language and was only introduced in C++.
The syntax to declare a function with an extern keyword in C++ is,
The return_data_type is the data type of the variable or value returned by the function named function_name.
Functions can also be declared global functions without using the extern keyword, as the compiler treats normal function declaration as a global function.
Working of C++ Extern
- The variable declared using the C++ extern keyword are linked externally by external compiler linking.
- The extern keyword instructs the compiler that the variable is declared here and defined elsewhere in the program. This helps the compiler prevent redeclaring the variable declared with the extern keyword.
- This makes them global and accessible from any part of the linked program. We can link two program files by including the name of the file with the following header syntax,
- The variable's lifetime is as long as the program runs and can be known as having static storage duration.
C++ Header File Without Extern
- The header files in C++ has the declaration or definition of variables that will be used in the program.
- The header file also has the prototypes of the function that will be defined in another file.
- The header file in C++ ends with the .hh extension.
Let us create the following file named globals.hh,
Then let us have the main file, main.cpp with the function definition and the main() function,
The output of the above program is,
Here we use the globals.hh file as an intermediate file to access the common global values. This method is less flexible when accessing variables from other files. The next method shows how the same process can be done with the extern keyword.
C++ Extern Without the Header File
Now, we can remove the header file and use two program files in which a program file contains the declaration of the program, and another file has the main() function.
The file func.cpp with function definition is as follows,
The main.cpp file is,
In the above example, the variable in other files is accessed by declaring the variable with the extern keyword.
Constant and Extern
A constant variable can't be changed or modified once the variable has been initialized with a value. A constant variable can be defined with the const keyword with the following syntax,
- The constant variable can't be declared without initializing it with a value. That is, const data_type variable_name; is not allowed and will result in a compilation error.
- On declaring a constant variable with the extern keyword, the variable can be declared without initialization. The syntax is as follows,
Then the variable's value can be defined in another file or any part of the program with the normal constant declaration syntax.
Consider the following simple program with two files. The first file is conants.cpp as follows,
The second file is main.cpp and has the main() function.
The output of the above program is,
Extern and static
The C++ static keyword is used to declare a variable, the compiler will allocate the required memory for the variable only once, and the variable can be accessed only within the file in which the variable is declared. The following syntax is used to declare a static variable,
The declared variable is not bounded by any scope within the file in which the variable is declared.
When a function is declared using the static keyword, only one instance of the function will be created throughout the program. The syntax to declare a function as static in C++,
Static functions are useful when a function is defined within a class but must be accessed without creating an object for the class. It is important to note that only static variables can be used inside a static function.
The C++ extern keyword makes a variable accessible through the program, and the C++ static keyword makes a keyword accessible through the file in which the variable is declared. Unlike the extern keyword, defining the variable with the static keyword is unnecessary.
When to use C++ Extern
- To declare a global variable and global function that is accessible through the program.
- To create libraries in C++ through which the extern functions and variables of the library can be used.
- To avoid redundant or duplicates of variables.
Examples of C++ Extern
Let us consider the header file globals.hh, which has all the global extern variables used in the program,
Let us have another file, definitions.cpp, which defines the variable declared with the C++ extern keyword and also has other function definitions,
Now, let us have the main file called main.cpp, which accesses both the global variable and function to modify the global variable,
The output of the above program is,
FAQs
Q. What are the reasons for the following error message while working with the extern keyword, and how can the error be resolved?
A. This error may be caused by not defining the variable that was declared with the extern keyword. Therefore, this error can be solved by defining the variable declared with the extern keyword using the following syntax,
Another reason for the above error may be not importing the files or header in which the variable is declared or defined. This can be solved by importing the file in which the variable is declared using the following syntax,
Q. What are the reasons for the following error, and how can the following error be resolved?
A. This error occurs due to defining the variable multiple times or importing multiple files containing the same variable's declaration.
For example, suppose the header file has the declaration of the variable global_var; it is imported in the second file, second.cpp, and the main.cpp file. In that case, the variable will be duplicated in both the files, second.cpp and main.cpp.
The above main program will cause an error, as the variable gloabl_var is imported in both second.cpp and also header.hh.
Related Articles
- Static members are allocated memory only one time in a program. Learn more about static keyword in C++
- When constant variables are defined, these variables can't be changed. Learn more about constants in C++
- The object-oriented programming concepts of C++ are important as these concepts are used to solve real-life problems. Learn about function in C++
- The header file is used to declare all the global variables and is used to follow the software principle of Single point of truth(SOP). Learn more about header files
Conclusion
- The C++ extern keyword is used to declare a variable or functions as global variables that can be accessed through the program.
- The variables in the header file can be made as extern variables from any part of the program.
- The C++ extern keyword can be used with the constant keyword to declare a variable without initializing the variable with a value.
- The static variables can be accessed from anywhere in a file but can't be accessed outside the file.
- The C++ extern keywords can be used to reduce the duplication of variables as global variables.