find() Function in C++
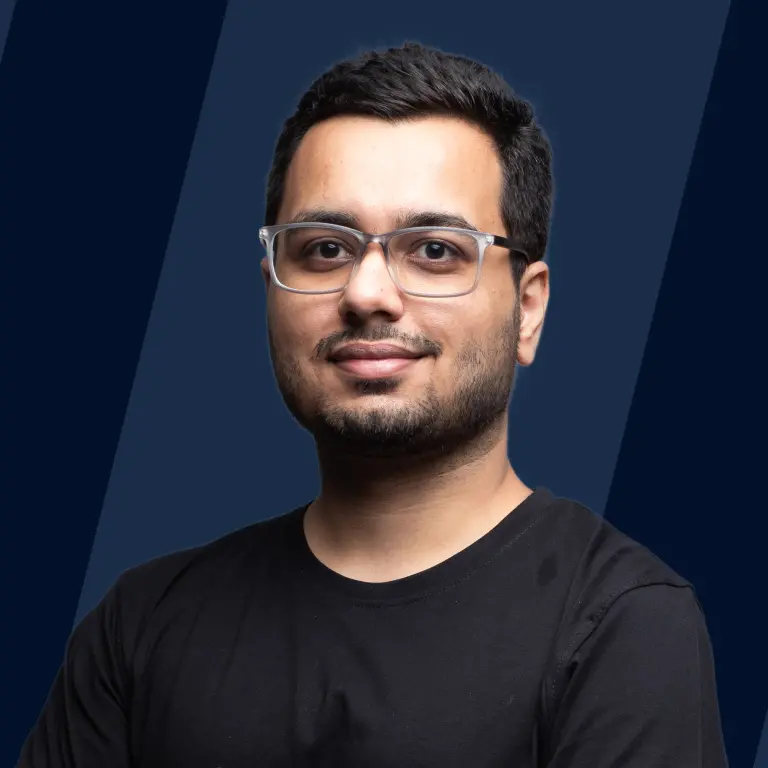
Overview
find() in C++ is a function that helps to search an element and returns the first occurrence of the element searched inside a specified range. It starts the search from the first element of the range and goes till the last one, if the element is not found after all possible comparisons then it returns the last element of the specified range. The find() function in C++ is defined in the <algorithm.h> header file. If any unessential action is performed by the find() function then it throws an exception that is not required by the programmer. find() function in C++ uses ==(Comparison operator) for every individual comparison between elements and values which is being searched.
Syntax of C++ find() Function
Let's see the syntax of the find() function where the template is having the InputIterator class with first, and last as the range and iterator for value comparison.
Syntax:
Note: first and last is the range in which the value will be searched.
Parameters of C++ find() Function
There is a total of three parameters that we use inside the find() function in C++ to search an element. They are as follows:
-
first:- It is an argument that specifies the first element of the range(first, last) inside which we want to perform the search for an element.
-
last:- It is an argument that points to the last element of the range(first, last) inside which we want to perform the search for an element.
-
value:- It is an argument that we want to search inside a given range(first, last).
Return Value of C++ find() Function
The find() function in C++ returns the iterator to the first element that refers to the value we want to search inside a particular range. If the value is not matched, then the function returns the iterator to the last element of the range.
Exceptions of C++ find() Function
The find() function in C++ throws an exception if the arguments or parameter used inside the function throws an exception. If the comparison of elements or operations on an iterator throws an exception then also the function throws an exception.
Note: If the parameters or arguments are not valid then it causes undefined behaviour.
How Does C++ find() Function Work?
find() function in C++ works in a different way than a normal function does. As an argument, they use the range that lies between the first and last element and key values that have to be searched. The function traverses the whole range of elements and finds the key value, if the value is found inside the range then the iterator points to the value and returns it. After every possible comparison if the value does not match, then the function returns the last element. The first occurrence of the key value is returned by the function if there is more than one key value located inside the range.
The comparison operator(==) is used for comparing and manipulating elements in sequence while finding the first occurrence of the key value. If any needless action is performed during the comparison of elements then the function throws an exception. Also if baseless or invalid arguments are passed in the function then it throws an exception. The find() function in C++ operates on linear time complexity which is O(n) and since we are not using any extra space then the space complexity will be O(1).
find() function in C++ aids in the reusability of the code as we don't have to define the function whenever we want to call it because it is already defined in the standard library used in C++. It is also used in dividing the program to make the code more clear and visible to the programmers which help in developing an enhanced version of the code.
Examples
For example, we have the following inputs, and accordingly, we are having outputs that show whether the given inputs are found or not.
-
Input: 25 11 16 14 33 Searching element 11 Output: Element 11 is found.
-
Input: 10 15 19 21 17 10 32 55 Searching element 7 Output: Element 7 not found.
-
Input: 9 21 45 58 Searching element 58
Output : Element 58 found at position : 3 (counting from 0th index)
Now, Let's understand them with proper coding implementation.
Example-1
Let's take an example that illustrates the use of the find() function in C++ inside a specific range which includes beginning and ending.
Code:
Output:
Explanation
In the above program, we have included the required header file which is required to run the program error-free. Then we declared a variable a which we want to search for having value 20 then we declared a vector vec with five elements inside which we will search the key value. After declaring the vector, we invoke the find() function where vec.begin(), vec.end() and a is passed as the parameters or arguments and the result is stored in res variable. Then we check whether res is equal to the last element of the vector then we display the message "Element is not found" and if the res is not equal to the last element then we will display the message "Element is found".
Example-2
Let's take another example to search an element using the find() function which will return the element's index.
Code:
Output:
Explanation
The above program is the same as 'example-1' but here the find() function in C++ is used to search the value from the actual vector using an iterator by matching all the elements. Here, if itr is not equal to the last element of the vector then we display the message "Element is found at " and also display the index of the element inside the vector.
Unleash your coding potential with C++. Enroll in our Free C++ course and learn to create powerful, performance-driven applications.
Conclusion
- find() in C++ is a function used to search an element inside a specified range and returns the first occurrence of the element.
- find() in C++ takes first_element, last_element, and the value which we want to search is taken as arguments.
- If the value is not matched within the range then the find() function returns the last element after all comparisons and searches.
- The time complexity of the find() function in C++ is comes out to be linear i.e, O(n).
- Since no extra space is used, the space complexity of the find() function comes out to be O(1).