How to do a for_each loop in C++?
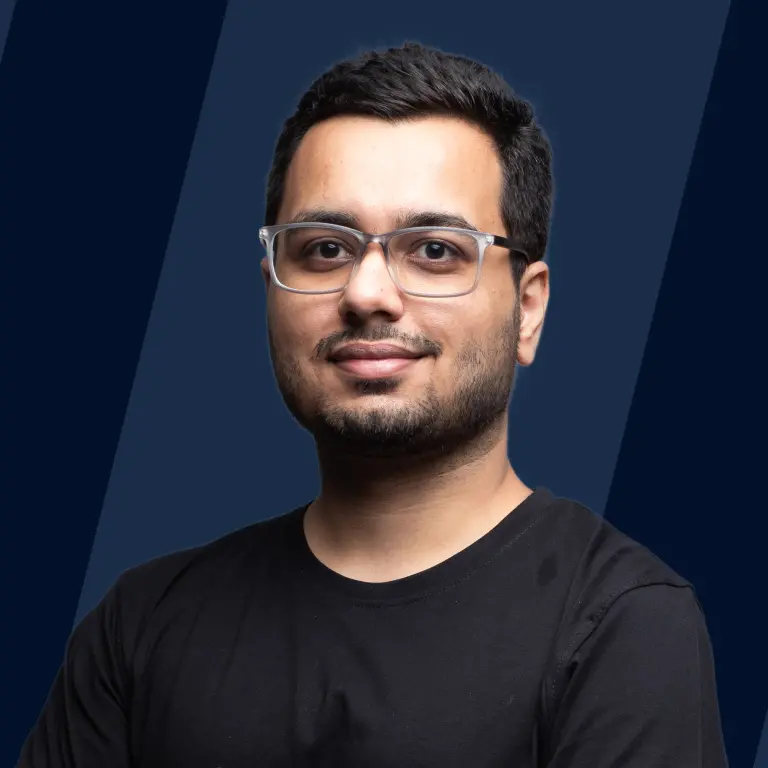
The for_each() is an inbuilt function used to iterate on the elements of a particular container, i.e., arrays, vectors, or any user-defined datatype. The for_each() function is in the <algorithm> header file.
Syntax of C++ for_each() Function
Let's understand the Syntax of the for_each() function in C++. The for_each() function takes three parameters.
Let's understand the parameters of the for_each() function in C++.
Parameters of C++ for_each() Function
- begin: The position we want to start the iteration on the particular container.
- end: The end position till we want to iterate the for_each function
- Function: The particular operation we want to perform on each container element.
Return Value of C++ for_each() Function
Let's understand the return type of the C++ for_each() function.
The return type of the C++ for_each() function is void. In simple terms, the for_each() function returns no value.
Exceptions of C++ for_each() Function
The C++ for_each() function throws an exception if any operation in the for_each() function during the iteration throws an exception.
Example of C++ for_each() Function.
Let's understand the C++ for_each() function using an example. In the below example, we are iterating on the first two elements of the given array using the for_each() function and printing the respective elements.
Output:
What is C++ for_each() Function?
The for_each() is an inbuilt function used to iterate on the elements of a particular container, i.e., arrays, vectors, or any user-defined datatype. The for_each() function is in the <algorithm> header file. The for_each() takes three parameters to iterate on the particular containers.
Purpose of for_each() Function in C++
Let's understand the purpose of the for_each function(). The for_each() function returns less code and doesn't allow any initialization or declaration statements in the body.
Advantages and Disadvantages of for_each Function in C++
Advantages of the for_each() Function
- The for_each() function requires less code than other loops in C++.
- The for_each() function makes the code more readable. Because the same for_each() convention can be used for different containers.
- The for_each() function is also used when we don't use the exact size of the container.
Disadvantages of for_each() Function
- We can't access the random element using the for_each() function in C++.
- We can transverse only in the forward direction in the for_each() function.
Examples of C++ for_each() Function
Let's understand the C++ for_each() function using an example.
Traversing Through an Array Using C++ for_each Function
In the below example, we are iterating on the elements of the given array using the for_each() function.
Output:
Traversing Through a Vector Using C++ for_each Function
In the below example, we are iterating on the elements of the vector elements using the for_each() function.
Output:
Using List with for_each Function
In the below example, we are iterating on the elements of the List elements using the for_each() function.
Output:
Using for_each Function with Lambdas
In the below example, we are iterating on the elements of the List elements using the for_each() function with lambdas. The lambdas expression is introduced in C++11, which helps to write the function in very short lines.
Output:
Conclusion
- The for_each() function in cpp requires less code than other generic loops.
- The for_each() function cannot access a random element of the particular container.
- The for_each() function can only transverse in the forward direction.