C++ Max
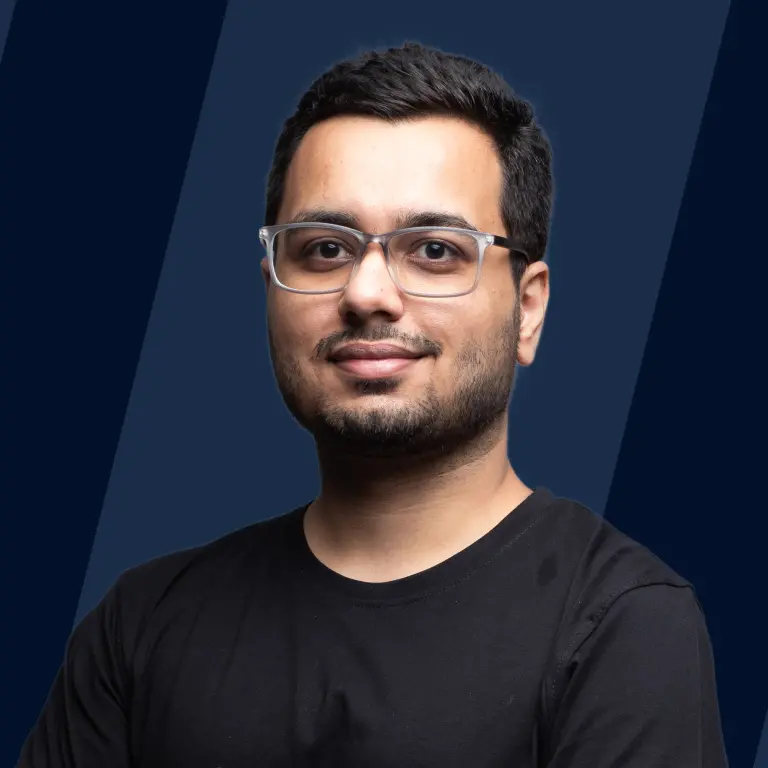
Overview
In C++, the max() function is used to find the largest element among many elements passed onto the function as parameters. We can also find the largest element by comparing them using a binary function defined by the programmer and then passing it as an argument or finding out the largest element in a given list.
If there is more than one larger element, the max(), the function returns the first occurrence. In C++, the max() function is available in the header file <algorithm.h>.
C++ max() Function
In C++, the max() function is very useful for programmers to find the largest element from a set of elements. There are three ways in which we can compare elements using the max() function, depending on how the elements are passed as an argument to the max() function.
-
Passing two elements onto its argument to compare - In this case, the larger value is returned. The first one is returned if both are equal. Example: std::max(5, 20)
-
Using a binary function - A binary function is a pre-defined function by the programmer which takes two inputs. The binary function is passed as an argument to the max() function. It compares the two inputs and returns true or false based on the condition defined by the programmer. Here, we have declared a binary function as cmp. Example: std::max( x , y , cmp )
-
From a list - In this case, the max() function in C++ finds the largest element in a given list. If there is more than one larger element in the list, it returns the first element. Example: max({15 , 2, -8 , 6}) or Example: max({10 , 5 , 37 , -13}, cmp)
Here, we have declared a binary function as cmp.
3 Versions of C++ max() Function
1. For comparing elements, using "< "
The max() function in C++ is used to find the largest of the numbers passed to it as arguments. The C++ max() function compares the numbers using the less operator.
Syntax
In this case, a and b are the values to be compared. It returns the larger value of the two.
The C++ max() function uses fewer operators to compare the values by default. Alternatively, you can specify your comparison operator or function if you don't want it to work with <.
Code
Output
In the above example, in line 7, we use the max() function to find the larger value between 2 and 5. If you see the other lines, we can also compare integers, floating point numbers, characters, and strings using ASCII values.
In the case of two similar numbers, the max() function returns the first one.
Time Complexity - O(1)
Auxiliary Space - O(1)
Parameters of C++ max() Function
Below are the parameters that can be passed to max().
Syntax:
a: It is the first number to be compared.
b: It is the second number to be compared with the first one.
Two or more variables with the same data type can be passed as parameters for comparison.
comparator: It is a binary function that takes two variables as parameters and returns a bool value.
Return Value of C++ max() Function
In the max() function, a variable of the same data as inputs is returned, and it is the largest among all the values passed.
Exceptions of C++ max() Function
When any comparison throws an error, the max() method also throws. Invalid arguments cause undefined behavior.
In the above example, we pass two values of different data types into the max() function, which is not a valid step. Similarly, the next comparison between a character and an integer cannot be compared.
Therefore, in the max() function, you must pass two variables of the same data type.
How does the C++ max() Function work?
std::max is a template function (defined in the header <algorithm>) that can be used to compare primitive datatypes. For non-primitive data types, the programmer can define a comparison function with the max() function. The less operator is used by default to compare the values. Alternatively, you can specify your comparison operator or function if you don't want it to work with <.
C++ max() function can therefore be used on primitive data types, i.e., floats, doubles, and long doubles since they provide less than the operator.
Uses of C++ max() Function
In the case of different types of arguments, std::max is safer because it requires explicit conversion. A 64-bit int, for example, can't be accidentally converted to a 64-bit float by the compiler. As a result, templates should be used as a default.
C++ max functions are type-specific, so you should prefer them if you're using C++. They provide type safety and prevent mixed-type comparisons, which can sometimes be undesirable.
Examples of C++ max() Function
Example 1
Using the max() function with the absolute values returns the maximum of the two values irrespective of their signs. Thus, the program returns -6 in the code below.
Code:
Output
Example 2
In this case, you can find the maximum value from an array using the below code.
Code:
Output
Explanation
We need to traverse the array elements using the max() function to find the maximum value from an array. Each element has to be compared with a pre-defined variable by the user. At the end of the array, you'll get the maximum element among all the array elements.
Related Functions in C++
- strcmp() in C++ - In strcmp(), the two strings are compared lexicographically, starting from the first character and continuing until the two strings have the same characters, or NULL characters are encountered.
- floor() in C++ - When used in C++, the floor() method returns the largest integer number that is less than or equal to the provided parameter.
FAQs
Q. Why is std::max preferable over fmax?
A. fmax is specifically designed to be used with floating point numbers (thus the "f"). As a result of the conversion, function call overhead, etc., you might suffer performance or precision losses if you use it with ints. The fmin/fmax command forces all floating point calculations to be performed.
Become a coding champion at your college with our Free C++ course. Enroll now and turn your passion into programming excellence.
Conclusion
- In C++, the max() function is used to find the largest element from a set of elements.
- There are three ways to find the maximum value. You can pass two values, use a binary function, or pass a list of elements into the arguments of the max() function.
- The max() function can be used on primitive data types, i.e., floats, doubles, and non-primitive data types, i.e., classes, arrays, etc.
- The max() method also fails when any comparison fails. Invalid parameters lead to an undefined response.
- The table below summarises all possible variations with the max() function in C++.
Function | Description |
---|---|
max(x, y) | Returns the larger value among x and y |
max(x, y, cmp) | Returns the larger value among x and y according to the binary function cmp |
max(initializer_list) | Returns the largest of all the elements of the list |
max(initializer_list, cmp) | Returns the largest of all the elements of the list according to the binary function cmp |