C++ min() Function
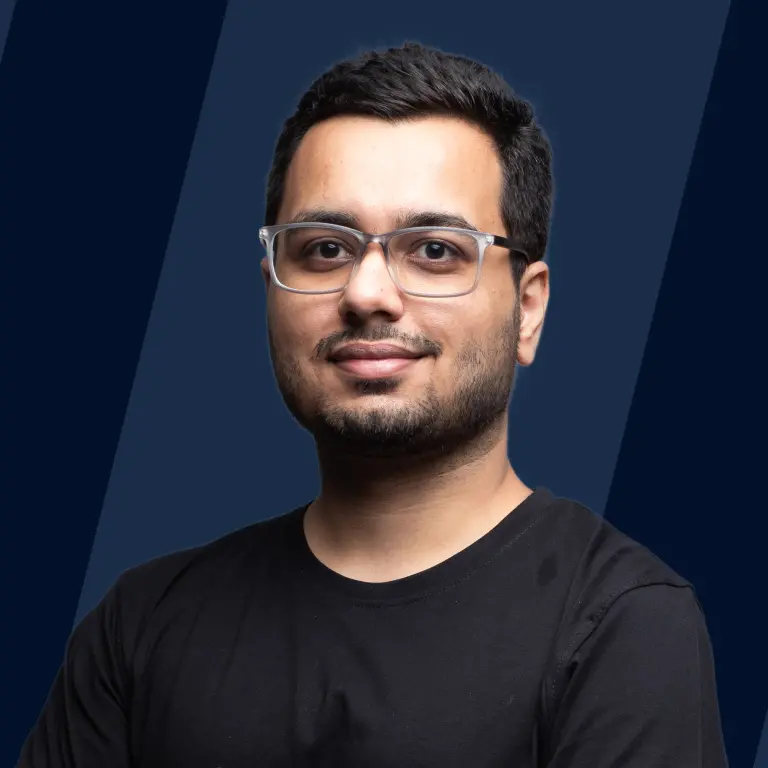
Overview
There are many inbuilt comparator functions in C++ that compare two or more values with each other. One such function is the C++ min() function. The article contains all the details about the C++ min() function.
C++ min() Function
The C++ min() function is a pre-defined function in the C++ library. It is defined in the algorithm header of the C++ library. It returns the minimum element from all the elements passed into this function as a parameter. If two minimum elements are equal, it returns the first element.
3 Versions of C++ min() Function
Version 1: For comparing elements using "< ".
It will compare the two elements passed as the arguments and will return the minimum of the two elements. If both elements are equal, then it will return the first one.
Syntax
C++ Implementation
Output
Time Complexity
The time complexity of the above code is because the min function compares only two elements with each other, which will take a constant amount of time.
Space Complexity
The space complexity of the above code is also because we are using just two variables, which will take a constant amount of space.
Time complexity :
Auxiliary Space :
Version 2: For comparing elements using a pre-defined function
It is also used for comparing the two elements passed as the arguments by using a binary function, which the programmer will define. It will return the minimum of the two elements, and if both are equal, it will return the first one.
Syntax
Code
Output
Time Complexity
The time complexity of the above code is because the min function compares only two elements with each other, which will take a constant amount of time.
Space Complexity
The space complexity of the above code is also because we are using just two variables, which will take a constant amount of space.
Time complexity :
Auxiliary Space :
Version 3: For Finding Minimum Element in a List
It is used for comparing the list of elements passed as the arguments using a comparator function, which the programmer will define. It will return the minimum of all the elements, and if two or more smallest elements are present, it will return the first one.
Syntax
Code
Output
Time Complexity
The time complexity of the above code is because the min function compares the list of elements with each other, which will take Time complexity.
Space Complexity
The space complexity of the above code is also because we are using just two variables inside the comparator function, which will take a constant amount of space.
Time complexity :
Auxiliary Space :
Parameters of C++ min() Function
The C++ min() function accepts a maximum of 3 arguments, the details of which are given below.
element1, element2: these are the values to be compared.
comp: This is a binary function that accepts two values as arguments of type T and returns a value after converting it to bool. The value returned is true if the element passed as the first argument is less than the second, else it will return false.
Return Value of C++ min() Function
The C++ min() function will return the minimum value from all the values passed in the function as an argument.
Exceptions of C++ min() Function
-
If the invalid arguments are passed in the min() function, it can cause undefined behavior.
-
It will throw an error if any comparison throws any exception.
How does the C++ min() Function work?
-
The min() function accepts two elements as an argument.
-
After that, it will compare the two elements with each other.
-
The element with the smaller value is returned by the min() function.
-
If the value of both elements is the same, then the min function returns the first element.
Uses of C++ min() Function?
-
It is used to find the smaller value from the two values passed as an argument.
-
It is also used to find the smallest value from the list.
Examples of C++ min() Function
Example 1:
In this example, we will see how the C++ min() function works for values of int data type.
C++ Implementation
Output
Example 2:
In this example, we will see how the C++ min() function works for values of float data type.
C++ Implementation
Output
Example 3:
In this example, we will see how the C++ min() function works for values of the char data type.
C++ Implementation
Output
Example 4:
This example shows how the C++ min() function works for values of double data types.
C++ Implementation
Output
Example 5:
In this example, we will see how the C++ min() function works for values of string data type.
C++ Implementation
Output
Related Functions in C++
Conclusion
In this quick tutorial, we have discussed the C++ min() function, and we can extract the following conclusions from the article.
-
The min() function is used to find the minimum element from all the elements passed as an argument.
-
The min() function compares two elements simultaneously to find the minimum element. If a comparator is passed, then it uses that to compare the two elements.
-
We should not pass any invalid arguments because if the invalid arguments are passed in the C++ min() function, then it can cause undefined behavior.