C++ Program to Find the First and Last Digits of a Number
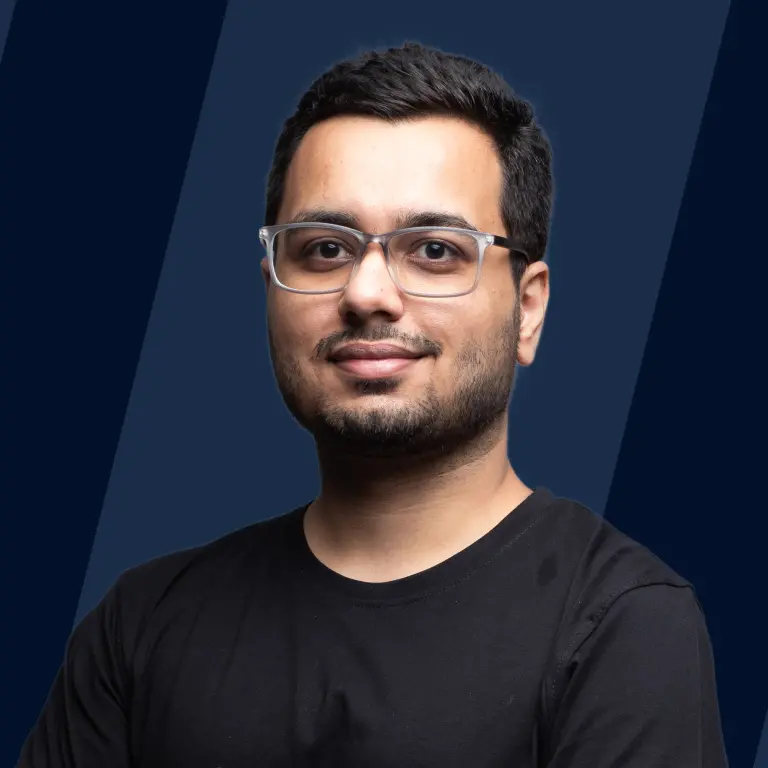
Overview
This article will help us write the C++ program to find the first and last digits of a number which would be of an integer datatype. Finding the first digit and last digit of a number is a very common and basic subpart of several coding questions.
Introduction
When dealing with coding questions, we often need to find the first and the last digit of a number to solve the bigger question. For finding the first digit, there are two approaches, With Loop and Without Loop, and for finding the last digit, we have a basic and common approach using the modulus operator(%).
Approach 1 (with Loop)
-
To find the number's last digit, we directly get it using the modulus operator (%). As in n%m, the modulus operator gives us the remainder when the n is divided by m.
-
For getting the first digit using loop, we can divide the number by 10 till the number is greater than 10 as when we divide a number by 10, the last digit gets removed each time. By doing this, we can also get the first digit of the number.
-
For calculating the first digit, we kept a copy of the number num in variable first_digit. Initially, it contains the original number, but after applying the above-mentioned approach, we would convert it to the first digit without changing the original number num.
-
This common approach in C++ programs is to find a number's first and last digits.
Eg: Original Number - 1526 Initially, variable num has to value - 1526, and then we copied the same value to variable first_digit - 1526 and after that, to get the 1 assigned to the first_digit variable, we would keep on dividing the first_digit variable by 10 till it contains value 1.
Code:
Output:
Approach 2 (without Loop)
-
Another very common approach without using loops in a C++ program to find the first and last digit of a number is given below.
-
For calculating the first digit of the number without using loops, we can first calculate the number of digits using the log10() functions as it gives us the value which, when raised to the power of 10, gives us the number num. So by taking the integer part of that log10(num), we can get the (number of digits of num-1) value.
-
For example, if num=1526, the log10(num) gives us the value 3.183, and by taking the integer part of this value, we get 3, which is equal to the value of (number of digits in num - 1).
-
So now, by dividing the number num by , we get the first digit of the number and calculate the last digit of the number. We directly get it using the modulus operator (%) as in n%m; the modulus operator gives us the remainder when the n is divided by m.
Eg: As in the above example, we have num - 1526 and no of digits of num -1 = 3
So when we divide num by , we get
. Now, by taking the integer part of the above value, we get 1, which is the first digit of the number num.
Code:
Output:
Conclusion
So in the above article, we learned two approaches in C++ program to find the first and last digit of a number. Some of the important points to recall when we need to find the first and last digit of the number :
-
In the C++ program to find the first digit of a number, there are two ways to calculate the first digit using :
- Loop
- Without Loop
-
To get the number's last digit, we can get that by using the modulus operator (%).
For example, the first and last digit of the number 3425 would be 3 and 5, respectively.