C++ push_back Function
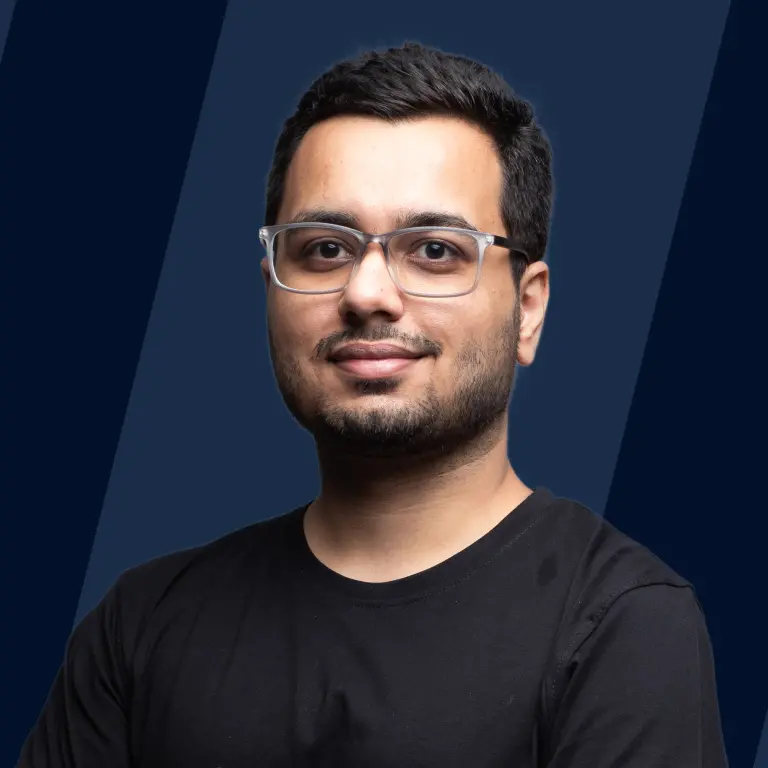
Vector is the part of C++ Standard Template Library. They are used to store the elements dynamically. push_back is one of the modifiers (used to modify a vector by removal or addition of elements) that exists in STL.
C++ push_back() is a pre-defined function that is used to insert data or elements at the end of the vector or it pushes the element in the vector from the back. The method is declared inside the <vector> and <bits/stdc++.h> header files.
C++ push_back() Function
Before learning about C++ pushback() function, let's learn a bit about STL and Vector.
In C++, the Standard Template Library (STL) is a set of template classes that provides access to common data structures and functions like the stack, arrays, list, etc. STL is a library that makes using different functions and data structures relatively easy.
STL contains a total of 4 components: - Algorithms - Containers - Functions - Iterators
** Vector ** is part of the C++ Standard Template Library. It stores elements dynamically. Vectors are STL containers that have a special feature to increase or decrease their size, considering the requirement of insertion and deletion inside a program.
The process of inserting and deleting elements starts from the last to the first. Deletion takes constant time, while insertion takes differential time, as a vector needs to resize after the element is accumulated.
In the case of a static array, we cannot insert elements once it is full, but when a vector becomes full, it doubles its size from the original. That's why it is beneficial to use a vector instead of a static array.
The vector class contains different member functions like other STL containers. Those member functions are as follows:- - Iterators - Capacity - Modifiers
- Iterators: Iterators are used to traverse and locate vectors, so contiguous memory is allocated to a vector to store the elements. There are different types of iterators in vector containers which are as follows:
-
cbegin(), cend(), begin(), end(), rbegin(), rend(), crbegin(), crend().
-
To learn about each iterator function in detail, refer to the article Vector in C++.
- Capacity: The member function which constitutes the size and capacity of a vector in C++. There are different types of capacity in a vector which are as follows:
-
size(), max_size(), reserve(), shrink_to_fit(), capacity(), empty(), resize(n).
-
To learn about each capacity function in detail, refer to the article Vector in C++.
- Modifiers: The functions used to modify a vector by adding or removing elements. There are different types of modifiers in a vector, which are as follows:
-
push_back(), pop_back(), insert(), assign(), erase(), swap(), clear().
-
To learn about each modifier function in detail, refer to the article Vector in C++.
Let's get back to the topic, which is C++ push_back() function.
C++ push_back() is a pre-defined function that is used to insert data or elements at the end of the vector or it pushes the element in the vector from the back. C++ push_back is a method that is part of the vector container in STL. Whenever an element is inserted into a vector, the size of the vector increases by one. The C++ push_back method is useless without a vector because it is broadly supported by the vector to manipulate the elements inserted within it.
Let's look at the syntax for using a C++ push_back method in a C++ program.
Syntax
Here, in the above syntax:
-
vector: It represents the name of the vector used inside the program.
-
push_back(): It represents the method push_back to insert element inside the vector.
-
value: It represents the value to be inserted inside the vector passed as an argument in the push_back() method.
Now, let's discuss about the uses of the C++ push_back method in detail.
Uses of C++ push_back() Function?
As the name suggests, C++ push_back method is used with a vector to push elements at the end of the vector that is after the current element.
As we know, a vector has the property of dynamically resizing means it adjusts its size when an element is pushed inside or deleted from the vector. Therefore, the size of the vector increases by one when an element is inserted at the end of the vector which automatically reallocates the storage space already allocated if the size of the new vector exceeds the current vector's capacity.
C++ push_back method is declared inside the <vector> header file and <bits/stdc++.h> header file. The Time complexity for insertion of elements using the C++ push_back method comes out to be O(1) as the operation is taking constant time.
Let's now see the parameter accepted in C++ push_back.
Parameters of C++ push_back() Function
The C++ push_back method takes only one parameter value as discussed above in the syntax section which specifies the value or data to be inserted or pushed inside the vector.
Return Value of C++ push_back() Function
The C++ push_back method which is used with vectors has no return value. It does not return anything, it just inserts the value in the vector.
Exceptions and errors of C++ push_back() Function
There may be certain errors and exceptions that we might face while using the C++ push_back method:
-
If the element which is passed as an argument in the push_back method is not supported inside the vector then undefined behavior will be shown.
-
C++ push_back method never throws an exception.
-
If any exception is thrown while using the push_back method then there will be no changes occurring in the container.
How does C++ push_back() Function Work?
As discussed in previous sections, Vector supports the C++push_back function and it is a built-in function of STL (Standard Template Library). C++ push_back method is used to insert an element in the vector from the rear end or back. C++ push_back method is used to perform manipulation with the elements inside the vector. Every time an element is inserted, the size of the vector increases by one. push_back method is a built-in function and that is why it is easy to use by the developers. There is no complex work done in the process of insertion of elements except that the element is inserted from one end and then the vector becomes useful for the end user. push_back method does not have any return type it just pushes a value inside the vector. If the push_back method is used then there will be no expectations thrown by the member functions.
As the push_back method only pushes elements inside a vector which is a very inexpensive task and therefore the time complexity comes out to be O(1) that is it takes constant time for insertion. There are no complex tasks to be performed rather than the insertion and deletion of elements. As discussed earlier, no exceptions will be thrown while using the push_back method which allows the developers to work without worrying about the exceptions. C++ push_back method which supports vectors can be used with different built-in data types like int, float, string, etc.
Examples of C++ push_back() Function
Let's learn about some of the practical implementations of the C++ push_back method used with vectors to understand it more clearly.
Example 1
In this example, we will create a simple vector and then we will start pushing elements inside that vector using the push_back method. Code
Output
Explanation
In the above example, we have declared a Vector vec1 which is of integer type. Inside vector vec1, we have inserted elements from 1 to 5 using the push_back() method which takes i as a parameter.
After the insertion of elements in the vector, we printed the pushed values using a for loop as seen in the output.
Example 2
In this example, we have created a vector with its values and then inserted a single value using the C++ push_back method which shows the insertion takes place from the back of the vector.
Code
Output
Explanation
In the above example, we have declared a vector vec2 with values. After declaration, the value 2 is inserted using the push_back method which is inserted from the back.
After insertion, we used iterator from the start of the vector to the end of the vector to print the elements of the same as seen in the output.
Example 3
This example demonstrates the creation of a vector and then inserting elements from the back using the push_back which increases the size of the vector by one each time an insertion takes place.
Code
Output
Explanation
In the above example, we have declared a Vector vec3 which is of integer type. First, we checked the size of the vector before the insertion. Then inside vector vec3, we have inserted five elements using the push_back() method.
After the insertion of elements in the vector, first, we printed the pushed values using a for loop and then printed the size of the vector after the insertion of elements as seen in the output.
Related Functions in C++
- Standard Template Library
Standard Template Library is a set of template classes that provides access to common data structures and functions like the stack, arrays, list, etc. STL in C++ is a library that uses different functions and data structures relatively easily.
To learn about STL containers like List in C++ refer to the article List in C++,Set in C++ refer to the article Set in C++, Maps in C++ refer to the article Maps in C++, Pair in C++ refer to the article Pair in C++, etc.
- Vector
Vector is the part of C++ Standard Template Library. They are used to store the elements dynamically. Vectors are STL containers that have a special feature to increase or decrease the size considering the requirement of insertion and deletion inside a program.
To learn about Vector in C++ in detail, refer to the article Vector in C++.
FAQs
Q. What is push_back in C++?
A. push_back method in C++ is a built-in function of STL (Standard Template Library) used with vectors to push elements in a vector.
Q. Why C++ push_back method is used?
A. C++ push_back method is used to insert data or elements inside a vector. The element is inserted from the rear end (back) in the vector. Whenever an element is inserted inside the vector, its size increases by one.
Q. What is the complexity of inserting elements using the push_back method?
A. As the push_back method only pushes elements inside a vector which is a very inexpensive task and therefore the time complexity comes out to be O(1) that is it takes constant time for insertion.
Conclusion
-
C++ push_back method is declared inside <vector> header file and <bits/stdc++.h> header file.
-
C++ push_back() is a pre-defined function that is used to insert data or elements at the end of a vector and having no return value.
-
Vector has the property of dynamically resizing means it adjusts its size when an element is pushed inside or deleted from the vector.
-
The Time complexity for insertion of elements using the C++ push_back method comes out to be O(1) as the operation is taking constant time.
-
The C++ push_back method takes only one parameter which specifies the value or data to be inserted or pushed inside the vector.
-
If the element which is passed as an argument in the push_back method is not supported inside the vector then undefined behavior will be shown.