C++ Program to Read File Line by Line
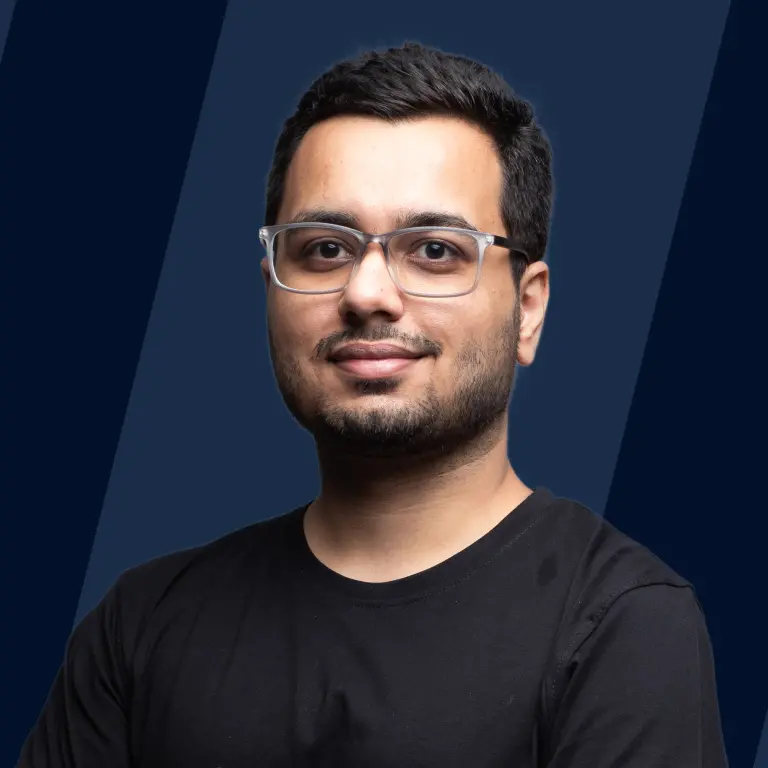
Overview
When working on any operating system, files play a crucial role in the daily lives of programmers, computer users, and students. These files assist us in storing our data securely and redundantly in various formats. The C++ examples to read data from a file line by line will therefore be covered in this article.
C++ Read File Line by Line
In C++ we can read files Line by Line by two methods. The first is to read the line token by token and the second is Line based on parsing, which is done using string streams that we get using the std::getline() Function.
std::getline() Function from a given string to read the content line by line into a std::string and later process them.
C++ Read File Line by Line Using std:: getline() Function
In C++, reading a file line by line is best done with the getline() function. When the delimiter char is reached, the function reads characters from the input stream and puts them in a string. The third optional element is the delimiter, which by default is taken to be the new line character \ns.
Syntax of std:: getline() Function
Here,
- is: It informs the method about the stream it should read input from and is an object of the stream class.
- str: The input is read from the stream and stored in this object, which is a string.
- delim: The function is instructed to cease reading more input when it reaches this character (provided by the user in the getline() function). It is optional.
Algorithm
- Begin
- Make an object to type a new file with the fstream class.
- To perform a write operation using the object newfile, call the open() function to open the xyz.txt file.
- If the file is open, then
- In the xyz.txt file, type the string My Function".
- Utilizing the close() method, close the file object newfile.
- To execute a read operation using object newfile, call the open() function to open the xyz.txt file.
- If the file is open, then
- Declare a string sa.
- Using the getline() method, read the entire data of the file object newfile, and then put it into the string sa.
- Print the data of string sa.
- If the file is open, then
- Utilizing the close() method, close the file object newfile.
- End.
Code Implementation
Output
Explanation
In the above code, we have first created an object new_file using the steam class. After that the file we have created we will open it and add some content to it and then close the file using the close() method.
After that, we will again open the file and create a string sa and then using the getline() function, we will read the entire file line by line and put the data into the string. After that, we printed the data, and at last, we closed the file.
Examples
Let's take an example to understand to concept of the getline() function.
Output
Ready to amplify your coding skills? Join our Free C++ course curated by industry experts and become a certified C++ pro!
Conclusion
- In C++, we can read a file line by line using the C++ STL library.
- We can use the std::getline() function to read the content of a file.
- The getline() function takes the 3 parameter as an argument. The third argument is optional.
- Any operations on a file must be verified to see if it is open.
- The line-based parsing in a file is always done through a string stream.
- The string that we provide reads the data from the file and then puts the data in the string.