What is sizeof Operator in C++?
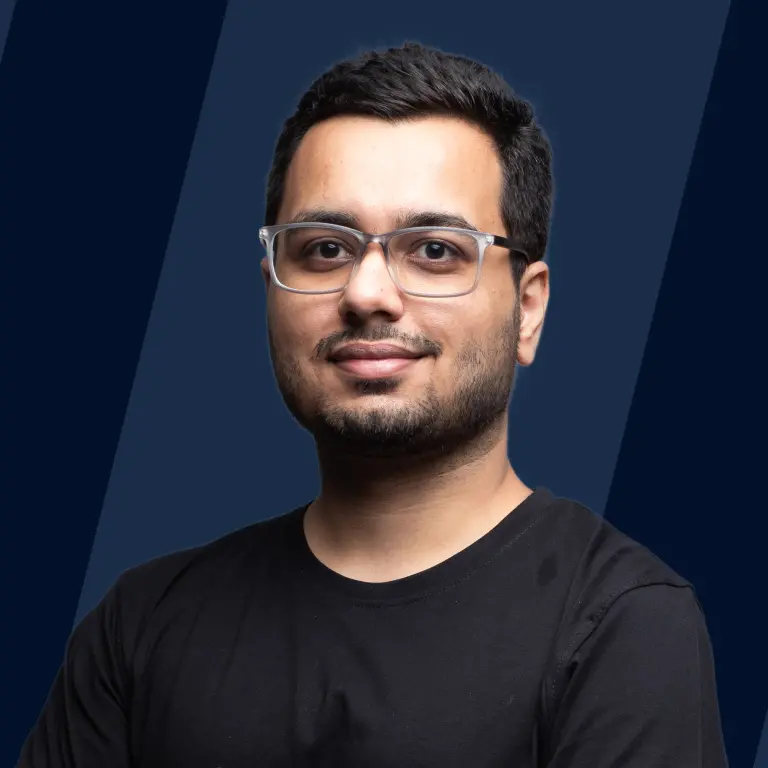
The C++ sizeof operator is a unary operator that calculates the size of data type, variables, and constants at compile time. The size returned from the operator is multiple of the size of char, which in most computers is 1 byte (8 bits). The value returned from the sizeof() operator is of the type size_t. It is an integer type defined in the header file <stddef.h>. This operator prevents programmers from specifying machine-dependent data size in the program.
The C++ sizeof operator operand can be one of the following:
- An expression: The C++ sizeof operator can be used with or without parentheses when used with an expression, and the expression is not evaluated.
- Type name: To use the C++ sizeof operator with a type name, it must be enclosed in parentheses.
The Syntax of the Sizeof Operator
When the operand is a data type, the syntax of the sizeof operator is as follows:
Here, the data_type can be the data type of variable, constants, data, structures, unions, or any other data type defined by the user.
How sizeof() Operator Works in C++?
The C++ sizeof operator can be used to return the size of a data type or expression and work straightforwardly by taking these values as an operand and working on them by returning their size in bytes.
Ouput
Here, the C++ sizeof operator helps find the size of data types and expressions. The sizeof operator is defined in the library iostream, which is included in the program. Using the sizeof operator, we can find the size of two data types, i.e., int and char. Notice that the value of the expression (1 + 2) is not evaluated; instead, we are returned the number of bytes that will be used to store the result obtained after evaluating the expression, which in our case is an integer or 4 bytes of memory.
Examples of Using the sizeof Operator
Let us see scenarios where the C++ sizeof operator can be used with examples.
a. When an Operand is of Data type
When the operand of a sizeof operator contains the data type of a variable, then the function returns the size of the data type passed as a parameter.
Output
Here, we evaluate the size of four different data types in C++ using the C++ sizeof operator. It is clear from the output that int occupies 4 bytes of computer memory, float occupies 4 bytes, double occupies 8 bytes, and char occupies only one byte.
b. When an Operand is of Class Type
The C++ sizeof operator can also be used to identify the size of data types created by users.
Output
For the above program, we can observe from the output that the class object takes 4 bytes of the memory. This was expected because the object has one data attribute, an integer taking 4 bytes of computer memory. If we add one more integer in the class definition, the size of the object will become 8 bytes, as shown in the program:
Output
c. When an Operand is of Array Type
Output
In the above program, we declare an array that contains five integer values. To evaluate the array, we used the C++ sizeof operator. According to the calculation, the value of sizeof(array) should be five times the size of the int, i.e., 5 * 4 = 20 bytes. The same result is obtained from the output using the sizeof operator.
When the C++ sizeof operator is allowed to a reference, the result is the same as the sizeof() operator applied to the object.
Let us calculate the array size using the function.
Output
Surprisingly, the array size in the above code is 8 bytes. This happened because we pass the pointer to the function here, and the function returns the size of an integer pointer int* whose size is 8 bytes in our 64-bit operating system.
d. When an Operand is of Pointer Type
Let us see what the sizeof operator returns when the operand is of pointer type.
Output
Here, from the output, we can observe the size of all the three-pointers are identical and in 8 bytes, but the size of the *pointer differ because the size of the data type pointed by the pointer in C++ is different. Because the first pointer point to an integer the size of 4 bytes, similarly, pointer two that points to a charred character occupies 1 byte, and the double data type pointed by the third pointer has the size of 8 bytes in memory.
e. When an Operand is an Expression
Output
In the example above, we declared two variables, var1 of type integer and var2 of type double. The result obtained by passing the expression to the sizeof operator is 8 bytes because the sum of an integer and double will be stored in type double, which occupies 8 bytes of computer memory.
Some Operands on Which the sizeof Operator Cannot be Applied
Some operands can not be used with the C++ sizeof operator. These are as follows:
- Functions
- Bit fields.
- Undefined classes.
- The type void.
- Dynamically allocated arrays.
- External arrays.
- Incomplete types.
- Parenthesized names of incomplete types
Although C++ throws an error when we try to pass a function as an operand in sizeof() functions, we can use the sizeof() function on the pointer to functions. For example, take a look at the below example.
Output
Here, we are using the sizeof() function to check the size of a function pointer greater and the function output in 8 bytes required to store the address of a function.
Benefits of Using sizeof()
Let us discuss the benefits of using the sizeof() operator:
- The sizeof() operator can help calculate and find an array's size or the number of elements contained in an array.
- The sizeof() operator can help us with the system type on which the program is running.
- The sizeof() operator helps check the memory taken by user-defined data types.
- The sizeof() operator programmers specify machine-dependent data size in the program.
Some Use Cases of sizeof():
a. To Find the Number of Elements in an Array
The sizeof() operator can be used to identify the size of an array in C++. Let us see how.
Ouput
b. To Allocate a Block of Memory Dynamically
The sizeof operator is of great help when we dynamically allocate memory. The sizeof() operator prevents us from hardcoding the values, thus making the dynamic memory allocation machine independent. For example, if we want to allocate ten blocks of integer memory and are unsure whether the computer is 64-bit or 32-bit, we can use the sizeof() operator.
Here, we use the sizeof() operator instead of guessing the value of a single integer.
Conclusion
- The sizeof() operator in C++ is a unary operator that calculates the size of data type, variables, and constants at compile time.
- The value returned from the sizeof() operator is of the type size_t.
- The sizeof() operator operands can be used with data types and expressions.
- The sizeof() operator can help to calculate and find the size of an array or the number of elements contained in an array or to allocate memory dynamically.