C++ String Replace
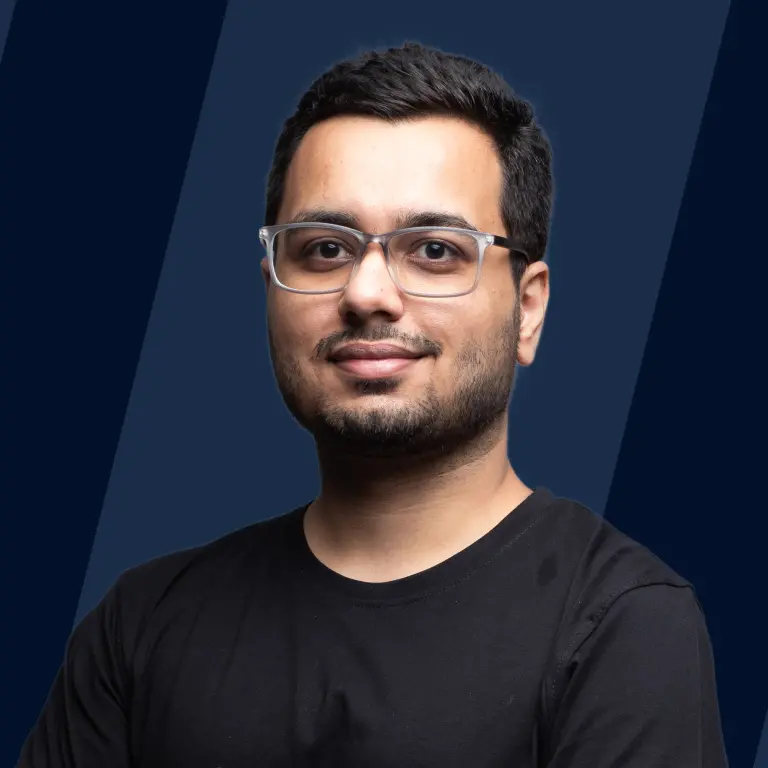
Overview
The C++ string replace function replaces a portion of the string starting from the given position up to provided length and replaces it with another given string. It is defined in the C++ string library, so to use the C++ string replace() function, we must include the <string> header file.
Introduction to C++ String replace() Function
The C++ string replace() function is used to replace a substring of a string with another given string. The position from where the substring has to be started, the length of the substring, and the other string which has to replace the substring are passed in the parameter as an argument.
Syntax of C++ String replace() Function
The C++ string function mainly takes three parameters.
Note: str1 is the string on which the replace function is performed.
Parameters of C++ String replace() Function
- Position : The position defines the starting position of the substring of str1.
- length: The number of characters starting from the position to be replaced by another string.
- str2: str2 is the string that will replace the substring of string str1.
Return Value of C++ string replace Function
The C++ string replace() function does not return any value.
Exceptions of C++ String Replace Function
- An out_of_range exception is thrown if the pos is greater than or equal to the str length.
- A length_error exception is thrown if the resulting string length exceeds the max size.
Note: The string will be unchanged if the program throws an exception.
How does the C++ String Replace Function Work?
Now, as we know, the C++ string replace() function is used to replace a portion of the string, which starts at the position covering the length amount of length, with the str2 string provided as an argument. Now we will see how the C++ replace function works with the help of an example and its explanation.
C++ Implementation
Output
Explanation
- In the first step, we declared string1 and initialized it with "Today I will eat Apple".
- In the second step, we declared string2 and initialized it with "Mango".
- Then we used the replace() function with the parameters as follows
- position = 17
- length = 5
- string2 = "Mango"
- The function replace() will replace the substring of string1, which will start from position 17 and have a length of 5, so the "mango" string will replace the "apple" string.
- Finally, we have printed the result.
Uses of C++ string Replace Function
- The C++ replace function is used to replace the substring of a string with another string.
- The C++ replace function makes the code more readable, as it is already implemented in the library. It works faster and requires less space.
Examples of C++ string replace Function
Example 1
In this example, we will see how we can use C++ replace() function to replace the substring of a string with another string.
C++ Implementation
Output
Explanation
- In the first step, we declared string1 and initialized it with the string "C++ and Java".
- In the second step, we declared string2 and initialized it with the string "Python".
- Then we used the replace() function with the parameters as follows
- position = 8
- length = 6
- string2 = "Python"
- The function replace() will replace the substring of string1, which will start from position 8 and have a length of 6, so the "Python" string will replace the "Java" string.
- Finally, we have printed the result
Example 2
In this example, we will see how we can use C++ replace() function to throw an error if we pass a position that is greater than the length of the string
C++ Implementation
Error
Explanation
The replace function will throw an out_of_range exception. This is because we have passed the position as 23, but the actual length of the string1 is less than 23, and the replace function cannot find the starting position of the substring, so it is throwing an out_of_range exception.
Related Functions in C++
- remove_if() function: The C++ remove_if() function is used to eliminate all the elements which satisfy the condition of a given range [first, last) without changing the order of the other elements.
- Other C++ String functions
Conclusion
- The C++ string replace function is used to replace a portion of the string, which starts at the position covering the given length amount of length, with the str2 string provided as an argument.
- The C++ string replace function takes three parameters as arguments i.e. position, length, and str2.
- If the position passed as an argument is greater than or equal to the actual length of the string, then the C++ replace function will throw an out_of_range exception.