C++ String to Double
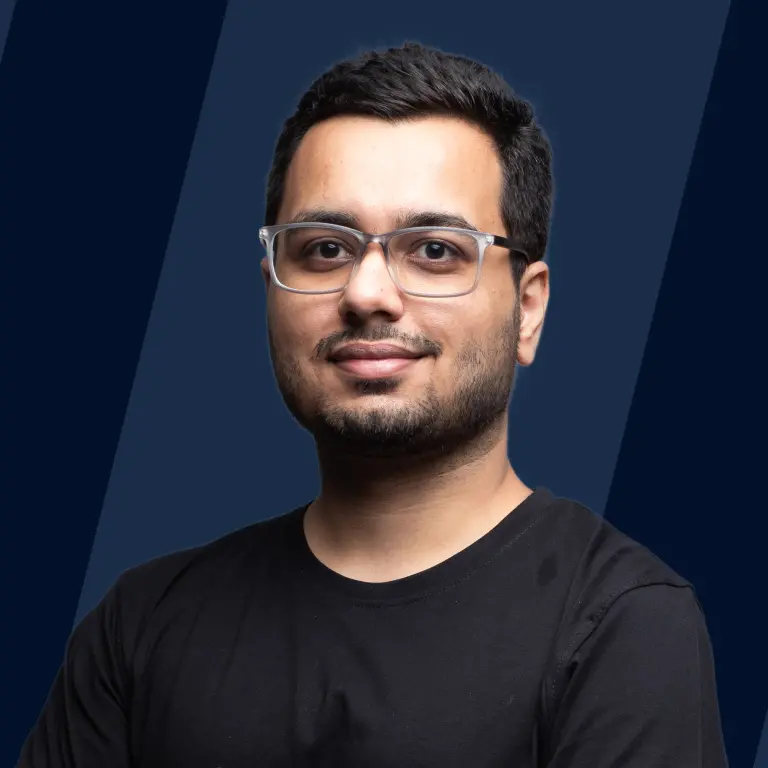
Sometimes in our program, we need to extract numerical values from a given string, now that string can be entered by the user or simply a pre-initialized string value into our program, and now to perform the extraction. First, we should know how to Convert String to Double in C++.
Different Ways to Convert String to Double in C++
There are various ways in C++ to convert a string value into a double value. For example -
- stod()
- stold()
- atof()
- stof()
Let's discuss each of the methods mentioned above in detail.
C++ String to Double Conversion Using stod()
Syntax
Parameters
The function stod() in c++ receives one compulsory parameter, str, and one optional parameter, position. str - the string we have to convert into double position - a type size_t value that will store the number of processed characters if the function call executes successfully.
Example
Program - C++ String to Double Conversion
Output
Explanation
We first initialize two string variables, str1 and str2. Then, with the help of the stod() function, we convert the above-initialized string values into double values. We compute the sum of the extracted values to demonstrate that the values are successfully extracted, and finally, we print the result.
C++ String to Double Conversion Using stold()
Syntax
Parameters
The function stold() in C++ receives one compulsory parameter, str, and one optional parameter, position.
- str - the string which we have to convert into double
- position - a type size_t value that will store the number of processed characters if the function call executes successfully.
Example
Program - C++ String to Double Conversion
Output
Explanation
Here again, we are firstly initializing two string variables, str1 and str2. After that, with the help of the stold() function, we convert the above-initialized string values into double values. Still, the difference here is the function stold() returns a long double value instead of the double value returned by the function stod(). Then we compute the sum of the extracted values to demonstrate that the values are successfully extracted, and finally, we print the result.
C++ String to Double Conversion Using atof()
Syntax
Parameters
The function atof() in c++, receives a single compulsory parameter str str - a character array consisting of a string which we have to convert into the float value
Example
Program - C++ String to Double Conversion
Output
Explanation
Here instead of two string values, we are initializing two character arrays to hold the target string because the function atof() works only on char arrays and not on string objects, after with the help of atof() function, we are converting the above char arrays into double values, remember the function atof() converts the given char array into float first, after that, we are manually doing widening conversion of that float value into a double value. We compute the sum of the extracted values to demonstrate that the values are successfully extracted, and finally, we print the result.
C++ String to Double Conversion Using stof()
Syntax
Parameters
The function stof() in c++ receives one compulsory parameter, str, and one optional parameter, position. str - the string which we have to convert into double position - a value of type size_t that will store the position of the next character in the str after the numerical value.
Example
Program - C++ String to Double Conversion
Output
Explanation
Here, we are firstly initializing two string variables, str1 and str2, after with the help of the function stof(), we are converting the above-initialized string values into double values, remember like the function atof() the function stof() as well converts the given string into a floating point number first, and after that, we are manually converting it to double, then we are computing the sum of the extracted values to demonstrate that the values are successfully extracted, and finally we are printing the result.
C++ String to Double Conversion Using strtod()
In this example, we will see how to use the strtod() function to convert a string value into a double value.
Program - C++ String to Double Conversion
Output
Explanation
Here, firstly we are initializing a char array str1. Along with that we are also declaring a char pointer ptr. After that, we call the function strtod() and pass the initialized char array and the declared char pointer. What the function strtod() does, is it parses the passed char array into the double value, stores the result into the variable d1, and sets the pointer ptr here to point to the first character after the last valid double character in the string, this is why in the output we can see the ptr is pointing to the first white space after the number 4 and using that we can print the whole remaining string.
Related Articles:
Conclusion
- Sometimes, in our program, we must convert a string value into a double value. Various ways are used to do that.
- We can use a list of c++ predefined functions like stod(), stold(), atof(), stof(), strtod() etc.
- All these functions accept the target string, which we have to convert and return a double or a floating point value based on the function we used.