What is the typedef Keyword in C++?
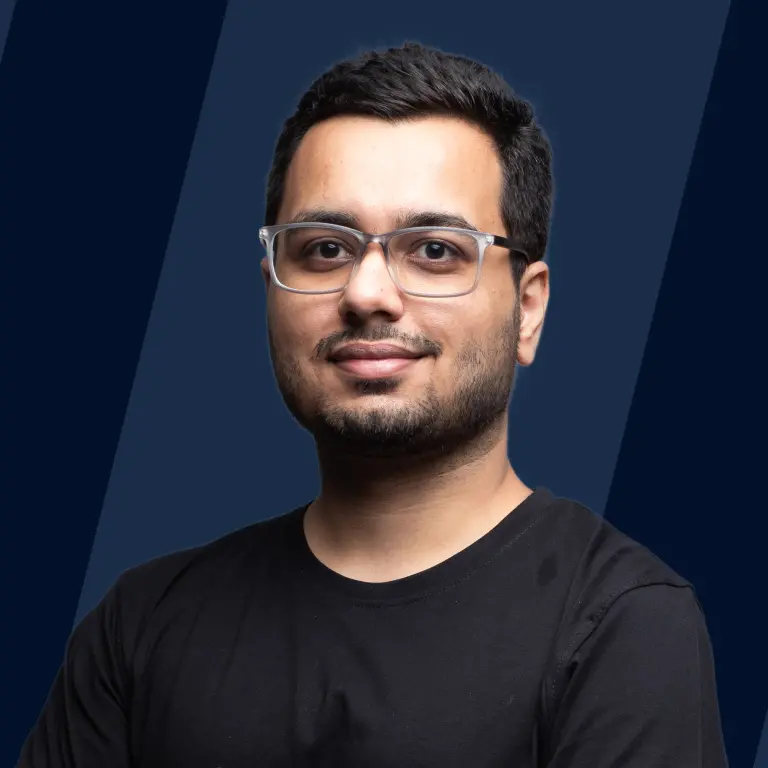
As the name suggests, typedef stands for type definition. C++ typedef is simply a way of giving a new name to an existing data type. In other words, it is a reserved keyword to create an alias name for a specific data type. The function or purpose served by the data type remains unchanged. We use it to simplify the code for the programmer's benefit while declaring higher-level data types such as structures and unions.
For example, if we want to declare some unsigned char variables, we must write unsigned char in a program, which can be tedious for some of us. So, we can use typedef to give unsigned int a new name of our choosing, which can be used whenever we want to use unsigned char in a program.
The Role of Typedefs
The typedef is a reserved keyword to create an alias name for a specific data type. The typedef keyword is simpler and easier to use than writing customized wrapper classes. Using the typedef keyword lets you focus more on what a variable should mean. This makes it easier to write clean code and makes it far easier to modify your code.
For example, Suppose you want to write a function get_data() that takes data as the `input and returns an error in the given data set. If you define your function in a general way, then it looks like
This type of declaration does not specify the exact purpose of the function or what the function will return. To avoid such confusion, we use the typedef keyword to modify the return data type to make our program more reliable and clean.
Now we can use error_t instead of float.
The above declaration clearly states that the function get_data() will return the error in the data.
Syntax of typedef Keyword
The syntax of typedef is very simple.
In the above syntax, existing_name is the name of an existing variable or data type, whereas 'alias_name' is another name assigned to it.
Example of typedef in C++
Let's take a simple example to understand the typedef keyword.
1. Use of typedef keyword with simple data type
Output
Explanation
We use the typedef keyword in the above code to replace the unsigned int with the var. We can now use var instead of unsigned int in the entire code when required.
2. Use of typedef keyword with structures
Output
Explanation
We use the typedef keyword in the above code to replace the struct student with the stu. We can now use stu instead of struct student in the entire code when required. It saves us from writing struct keywords again and again in our code.
3. Use of typedef keyword with pointers
Output
Explanation
We use the typedef keyword in the above code to replace the int* with the ptr. We can now use ptr instead of int* in the entire code when required. The name ptr itself suggests that it is a pointer variable. This increases the overall readability of the code.
How Does typedef Work in C++?
- typedef is one of the reserved keywords. It gives the same level of Abstraction as the actual data type and modifies data types that programmers use to allow them to focus more on the coding concepts. It also makes it easy to write programming codes and clean them using destroy() or other default ways because garbage collectors are the primary region for deleting unnecessary codes and cleaning up memory space locations. The sizes must be computed based on the data types, and it allocates memory space for large storage data type variables and small amounts of storage variables.
- The typedef keyword is also used to simplify variable declarations for some compound types such as struct, union, etc. We may also utilize structure pointers in the typedef keyword to declare many variables of the same type with single-level statements, regardless of whether the pointers are included in the structure type. The typedef keyword will be used to rewrite the preceding number of coding lines that specify functional pointers, which shortens and simplifies the programs' length and complexity. The functional declarations will be more obscure, and they explicitly state that they will return variables by accepting certain types or inputs.
- The two standard typedef declarations, typedef type-declaration, and typedef type-definition identifier are covered by the new name types alias with certain syntactic declarations for the language identifiers. The other type is covered with some standard libraries, and in other POSIX (Portable Operating System Interface), specifications are included with the typedef definitions. It is more frequent in both prefixes and suffixes.
How Do typedefs Provide Abstraction from the Original Types?
The typedef keyword provides a level of Abstraction away from the actual data types (data type of the variable) being used, allowing you to focus more on the concept of what a variable should mean. This makes it easier to write clean code and makes it far easier to modify your code. For example, if you decided you needed to support sizes that were too large to store in an int, then you need to make just one change in your entire code, i.e., the typedef itself, to make size_t equivalent to a long.
This approach of employing typedefs can also be helpful, especially when using the STL extensively because the STL template can be long and difficult to type. Typedefs allow you to generate abbreviations for these long type names quickly, making modifications across your code much easier if you want to modify the templated type.
For example, if we want to declare a map of strings to long, it isn't very pleasant to write the syntax again and again in your code. Additionally, it provides minimal information on what it actually signifies. Using a C++ typedef here saves typing while also adding clarity.
We now use the typedef keyword to redeclare it more meaningfully.
It is now evident that this map is intended to represent the scores associated with various individuals. Furthermore, if you decided you wanted scores to be floating point numbers, you could easily change the mapping from integers to floating point values.
Difference between typedef and #define
typedef | #define |
---|---|
The compiler performs the interpretation of the typedef keyword. | The interpretation of the #define statements is performed by the preprocessor. |
Typedef definition should be terminated with a semicolon. | #define should not be terminated with a semicolon |
The typedef keyword is only capable of assigning symbolic names to types. | On the other hand, #define can be used to define an alias for values as well, e.g., you can define 1 as ONE, 3.14 as PI, etc. |
The typedef keyword follows the scope rule, which states that if a new type is defined within a scope (within a function), the new type name will only be visible while the scope is present. | In the case of #define, when the preprocessor sees it, it replaces all occurrences (No scope rule is followed). |
Conclusion
- Typedef stands for type definition. typedef is simply a way of giving a new name to an existing data type. In other words, it is a reserved keyword to create an alias name for a specific data type.
- C++ typedef keyword is also used to simplify variable declarations for some compound types such as struct, union, etc.
- The syntax of C++ typedef is very simple.
- The typedef keyword provides a level of Abstraction away from the actual data types (data type of the variable) being used, allowing you to focus more on what a variable should mean.
- The compiler interprets the typedef keyword, whereas the preprocessor performs it in the case of #define.
- The typedef keyword can only assign symbolic names to types, whereas #define can be used to define an alias for values as well, e.g., you can define 1 as ONE, 3.14 as PI, etc.