C++ Vector Insert
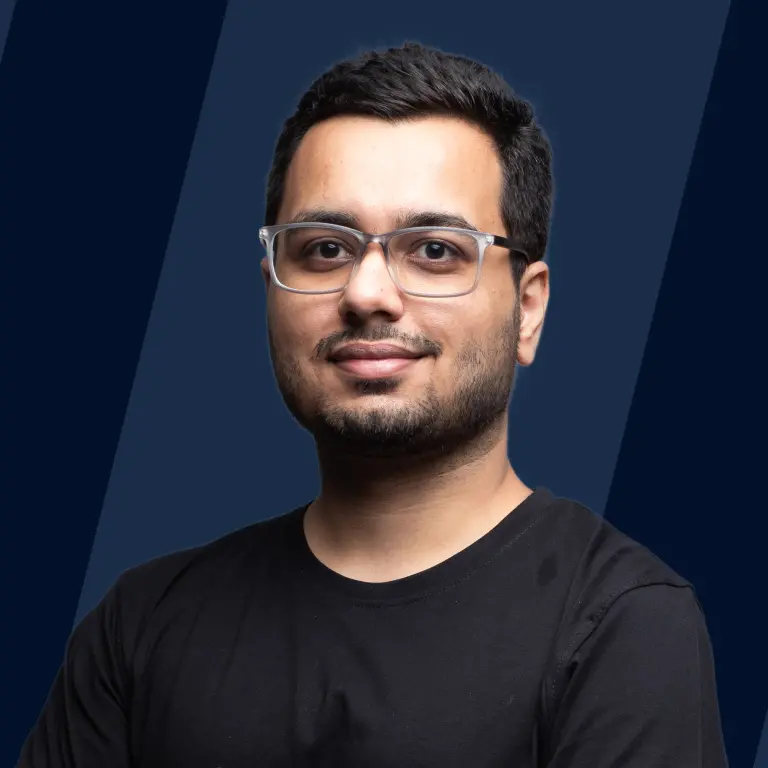
Overview
Vector is a dynamic and linear data structure defined in the Standard Template Library of C++. The vector insert function is defined in the <vector> header file of C++. It is used to insert a single element into a vector, the same element some number of times into a vector, or a list of elements from another iterable data structure like a set, deque, vector, etc., into a vector. We have to give the starting position or iterator indicating the starting position from where the insertion will begin in the vector.
Introduction to C++ Vector Insert Function
Vector is better than the arrays as it can be of the dynamic size, and a new memory block can be removed or added to the vector. The vector insert function gives a better experience of adding a new element to the vector at a given position which could be any position up to the size of the vector or at the end of the vector. For example:
In the above image, we first added an element at the starting position of the vector, then in the middle of the vector, and finally at the end of the vector.
We can add a single element or the same element some number of times in the vector, starting from the given first position given number will be added a given number of times, and all the elements present there will appear after the newly added elements. Let’s see an image for this:
In the above image, we have added elements 3 and 4 several times between 2 and 4, starting from index 2.
A third way to use the C++ vector insert method is to add some continuous elements from any iterable data structure like a set, vector, etc. Let's see an image to understand it in a better way:
In the above image, two vectors are present, and we inserted the second vector into the first vector at index 3 or after 7.
Parameters of C++ vector insert Function
There are three ways to use the vector insert function based on how we pass the arguments to it and which type of parameters we are passing to it, but the working of the C++ vector insert function remains the same. Let’s see each way to use the C++ vector insert function:
Syntax1:
In the above syntax, vector_name is the vector to which we want to add the data. Let's see its parameters:
- position is an iterator pointed toward the position where we want to add the data.
- val has the same data type as the vector_name vector. It is the value that we want to add to the vector.
Both parameters are compulsory to add, and none of them is optional.
Syntax2:
In the above syntax, vector_name is the vector to which we want to add the data. Let's see its parameters:
- position is an iterator pointed toward the position where we want to add the data into the vector.
- size is the variable indication number of times the given value will be added to the vector
- val has the same data type as the vector_name vector. It is the value that we want to add to the vector.
All the parameters are compulsory to add, and if we miss adding size, then this syntax will look like the previous syntax.
Syntax3:
In the above syntax, iterator1 and iterator2 are the iterators of any iterable data structure like vector, set, deque, etc., and will data all the data from there or the vector_name vector at the given position. Here, iterator1 indicates the starting position of the data structure from which we want to take data to add to the vector, and iterator2 indicates the ending position before which we need to take data. All three parameters mentioned above are compulsory to add, and none are optional.
Return Value of C++ vector insert Function
The return value of the C++ vector insert function is an iterator. This iterator will point towards the index where the value is added in case of adding a single element or the index from where we start adding multiple values to the vector in case we add more than one value.
Exceptions of C++ vector insert Function
Usually, the C++ vector insert function works fine without throwing any exceptions, but in some cases, it might not work. Let’s discuss all of them:
- If the given elements to add are not copyable, it will throw an error indicating they are non-copyable.
- It will show undefined behavior if the position given by the iterators is invalid and will throw a segmentation fault error, and the program will halt.
Examples of C++ vector insert Function
We have seen the basics of the C++ vector insert function and different ways to use the insert function. Now let’s see an example of each case we have discussed for using the C++ vector insert method:
Inserting a Single Value into the Vector
For inserting a single value in the vector, we will use syntax1. We will create a vector and add a single value in the middle of the vector.
Code:
Output:
Explanation:
In the above code, we have declared and initialized a vector vect. After that, using the C++ vector insert function, added the value 4 after the second index ( 0-based indexing ) that is pointed by vect.begin() + 3. At last, printed the values of the vector using for loop.
Inserting the Same Value Multiple times
For inserting the same multiple values in the vector, we will use syntax2. We will create a vector and add a value some number of times in the middle of the vector. We will provide a number and a frequency that indicates the number of times the given number will be added to the vector.
Code:
Output:
Explanation:
In the above code, we have declared and initialized a vector vect. After that, using the C++ vector insert function, we added the value 3, 5 times after the first index ( 0-based indexing ) that is pointed by vect.begin() + 2. At last, printed the values of the vector using for loop.
Insert Another Vector in Vector at Any Position
For inserting the other vector in the given vector, we will use syntax3. We will create two vectors and add all the values present in the second vector into the first vector at a given position.
Code:
Output:
Explanation:
In the above code, we have declared and initialized two vectors, vect1 and vect2. After that, using the C++ vector insert function, we added the value of vector vect2 from the zeroth index (vect2.begin) to the last index ( using vect2.end() to give one index next value) into the vect1 after the second index ( 0-based indexing ) that is pointed by vect1.begin()+3—at last, printed the values of the vector using for loop.
How does the C++ vector Insert Function Work?
The C++ vector insert function is defined in the <vector> header file. We can add a new element at the last of the vector using the push_back function. For adding a new element at any position of the vector, we can use the insert function. Also, by using the insert function on a vector, the current vector changes as new values are added, and no new vector is created. The vector insert function takes an iterator as a parameter to add the given value into the vector and adds the given value/values there. As the size of the vector increase by adding a new value or values, the automatic reallocation of the memory of elements may occur. If the size of the vector increases more than its capacity, it will throw an error of memory limit pass. The vector insert function performs a good job and does the work of many lines in a single expression for adding a new value in the vector at any place. Still, adding the values takes the average O(N) time (where N is the total number of elements in the vector).
Related Functions in C++
We have seen the working of the C++ vector insert function with examples. These are some articles that are related to the insert function: Vector in C++
Conclusion
- Vector insert is used to insert a single element, the same element some number of times, or a list of elements from another iterable data structure, like a set, deque, vector, etc., into another given vector.
- There are three ways to use the vector insert function based on how we pass the arguments and which parameters we pass to it.
- The return value of the C++ vector insert function is an iterator pointed toward the first position where the value is added to the vector.
- If the given elements to add are not copyable or the position given by the iterators is invalid, then the vector insert function throws an error.
- The average time complexity of the C++ vector insert function is O(N), where N is the total number of elements present in the vector.