C++ Vector Resize
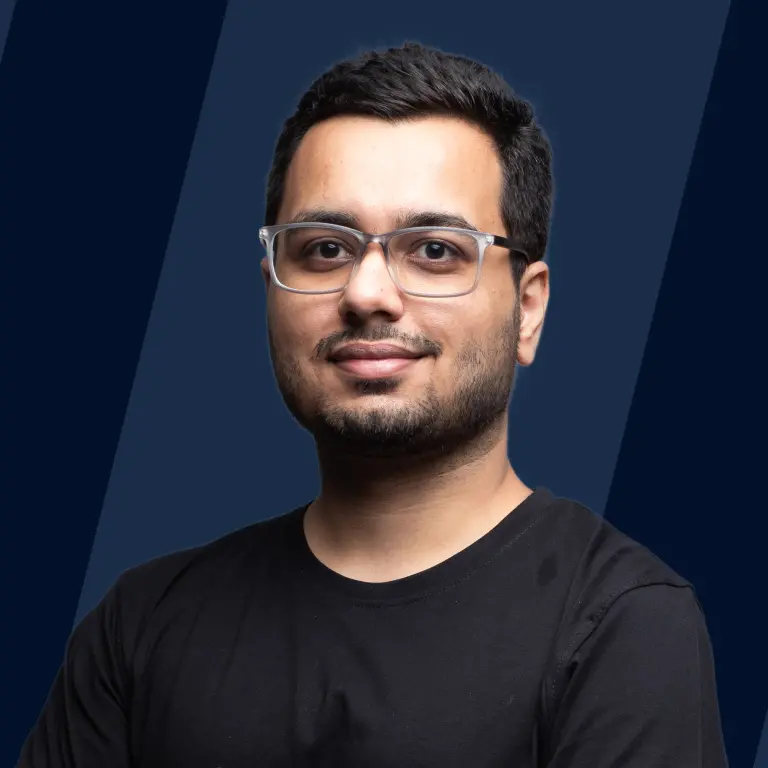
Overview
Capacity is the member function that constitutes the size and capacity of a vector in C++. The C++ vector resize method is a built-in STL function (capacity function) used to manipulate a vector's size by inserting or deleting elements from the vector. C++ vector resize method is defined in the <vector> and <bits/stdc++.h> header file, which has to be included in the programs where the resize method will be used.
Introduction to C++ Vector Resize Function
Before learning about the C++ vector resize method, let's learn about STL.
In C++, we have the Standard Template Library, a set of template classes that provides access to common data structures and functions like the stack, arrays, lists, etc. STL in C++ is a library that uses different functions and data structures relatively easily.
STL contains a total of 4 components: - Algorithms - Containers - Functions - Iterators
Algorithms
Algorithms are the collection or group of different functions used to work on various elements. They provide different operations that are to be performed on the elements of a container. There are several algorithms present in STL, such as Sorting algorithms, Searching algorithms, etc.
Containers
Containers, also known as Container classes, are used to store data and objects. Several types of containers are available in STL, like vector, arrays, queue, list, etc.
Functions
Functions are the instance of classes present in the STL that can overload the function call operator.
Iterators
Iterators, as the name suggests, work upon a sequence of values. Iterators allow generality in the standard template library.
Now let's learn about Vectors in C++.
Vector is part of the C++ Standard Template Library. They are used to store the elements dynamically. Vectors are STL containers with a special feature to increase or decrease the size considering the requirement of insertion and deletion inside a program. To use Vector inside a C++ program, we have to include <bits/stdc++.h> and <vector> header file.
The process of inserting and deleting elements starts from the last index to the first one. Deletion takes constant time, while insertion takes differential time as a vector needs to resize after accumulating the element.
In the case of a static array, we cannot insert elements once it is full, but when the size of a vector becomes full, it doubles its size from the original. That's why using a vector instead of a static array is beneficial.
The vector class contains different member functions, just like other STL containers. Those member functions are as follows:- - Iterators - Capacity - Modifiers
- Iterators: Iterators are used to traverse and locate vectors, that are why contiguous memory is allocated to a vector to store the elements. There are different types of iterators in vector containers which are as follows:
- cbegin() , cend(), begin(), end() , rbegin(), rend(), crbegin(), crend().
- Capacity: The member function constitutes a vector's size and capacity in C++. There are different types of capacity in a vector which are as follows:
- size() , max_size(), reserve() , shrink_to_fit(), capacity(), empty(), resize(n).
- Modifiers: The functions which are used to modify a vector by adding or removing elements from it. There are different types of modifiers in a vector which are as follows:
- push_back(), pop_back(), insert(), assign(), erase(), swap(), clear().
Now, let's get back to our topic, which is a method.
The C++ vector resize is a built-in function of STL (Standard Template Library) which is used to manipulate the size of a vector by inserting or deleting elements from the vector. The size of the vector can be increased or decreased using the resize() method. The C++ vector resize method is defined in the <vector> and <bits/stdc++.h> header file, which has to be included in the programs where the resize method will be used.
The C++ vector resize method changes the size of a vector so that it can contain the memory space used to store some number of elements, where the number of elements will be passed as the parameter to the resize function.
The number of elements will be passed as the parameter. If the number of elements is more than the vector's current size, then the vector's size will get expanded by inserting as many elements as needed at the end.
Let's look at the syntax used for using the C++ vector resize` method in a program.
Syntax
new_size and value is passed as a parameter in the resize method.
Parameters of C++ Vector Resize Function
In the above-given syntax, vector_name represents the vector used in the program. new_size represents the new vector size. value represents the value to be inserted in the vector.
Return Value of C++ Vector Resize Function
The C++ vector resize function has no return type or value. It is just used to manipulate the size of a vector.
Exceptions of C++ Vector Resize Function
A bad_alloc exception is thrown if the memory reallocation fails to the vector after using the resize().
Examples of C++ Vector Resize Function
Let's look at some examples to understand the usage of the C++ vector resize function in different conditions, like when the size of the vector is decreased after resizing, the size of the vector is increased after resizing, etc.
Size of the vector container is decreased after resizing.
In this example, we will create a vector and then push the elements inside it using the push_back() method. After this, we will resize the vector by decreasing its size using the resize() method.
Code
Output
Explanation
In the above program, after declaring and pushing the values inside the vector vec1, we have printed the elements present inside the vector before using the resize() method. Now, we used the resize() with a parameter of size 6, which is less than the size of the vector. Since the value of size is less than the current vector size, the size of the vector will be decreased, and it destroys the extra elements inside the vector, which can be seen in the output above.
Size of the Vector Container is Increased After Resizing.
In this example, we will create a vector and then push the elements inside it using the push_back() method. After this, we will resize the vector by increasing its size using the resize() method.
Code
Output
Explanation
In the above program, after declaring and pushing the values inside the vector vec2, we have printed the elements inside the vector before using the resize() method. Now, we used the resize() with a parameter or size 9, which is more than the size of the vector. Therefore, if the size value is more than the current vector size, the size of the vector will be increased. Since no particular value is provided in the parameter to be initialized, the remaining space will contain a value of 0 which can be seen in the output.
Size of the Vector Container is Increased After Resizing, and New Elements are Initialized with the Specified Value
In this example, we will create a vector and then push the elements inside it using the push_back() method. After this, we will resize the vector by increasing its size and initializing new elements with a particular value using the resize() method.
Code:
Output
Explanation
In the above program, after declaring and pushing the values inside the vector vec3, we have printed the elements inside the vector before using the resize() method. Now, we used the resize() with a parameter or size 9, which is more than the size of the vector. Therefore, if the value of size is more than the current vector size, the size of the vector will be increased, and since the new value to be initialized is specified in the resize() method, the remaining space will contain the same value which can be seen in the output.
How does the C++ Vector Resize Function work?
As we have discussed earlier, the C++ vector resize method is used to change the size of a vector by inserting or deleting elements from the vector. The vector is resized in such a way that it contains the memory to accommodate some number of elements in which the number of elements is passed as the parameter to the C++ vector resize method. Suppose the number of elements to be accommodated in the vector is greater than the current size of the vector. In that case, the vector will be expanded to the memory space equal to the parameter passed in the resize() method needed to store the required number of elements at the end. A new value is passed to be initialized as an element in the vector then all the newly allocated memory space inside the vector will contain the same value. If no value is passed in the parameter, then the newly allocated memory space in the vector will contain 0.
As in the previous section, we have discussed some examples in which we have increased and decreased the size of the vector using the resize() method. In one of the examples, it is shown that if we don't specify a value and increase the size of the vector using resize(), then the newly allocated space will contain 0 as the element. Similarly, one of the examples shows that if we specify the value to be initialized when we increase the vector size using the resize() method, then the newly allocated spaces will contain the same value.
Please refer to the "Examples of C++ Vector Resize Function" section to better understand the working of the C++ vector resize method.
Related Functions in C++
-
Standard Template Library Standard Template Library is a set of template classes that provides access to common data structures and functions like the stack, arrays, list, etc. STL in C++ is a library that uses different functions and data structures relatively easily. To learn about STL containers like List in C++ refer to the article List in C++,Set in C++ refer to the article Set in C++, Maps in C++ refer to the article Maps in C++, Pair in C++ refer to the article Pair in C++, etc.
-
Vector Vector is part of the C++ Standard Template Library. They are used to store the elements dynamically. Vectors are STL containers with a special feature to increase or decrease the size considering the requirement of insertion and deletion inside a program. To learn about Vector in C++ in detail, refer to the article Vector in C++.
FAQs
Q. What is vector resize() method in C++?
A. The C++ vector resize is a built-in function of STL (Standard Template Library) that manipulates the size of a vector.
Q. Why C++ vector resize() method is used?
A. C++ vector resize method is used to manipulate the size of a vector by inserting or deleting elements from the vector. The size of the vector can be increased or decreased using the resize() method.
Q. What is the complexity of resizing a vector using the resize() method?
A. As the resize() manipulates the size of a vector according to the parameter passed in the resize() method, the time complexity comes out to be O(n). That is, it takes Linear time to resize the vector.
Conclusion
- The C++ vector resize is a built-in function of STL (Standard Template Library), which is used to manipulate the size of a vector by inserting or deleting elements from the vector.
- C++ vector resize method is defined in <vector> and <bits/stdc++.h> header file.
- The C++ vector resize method changes the size of a vector so that it can contain the memory space used to store some elements.
- The number of elements will be passed as the parameter. If the number of elements is more than the vector's current size, then the vector's size will get expanded by inserting as many elements as needed at the end.
- If the number of elements is less than the vector's current size, then the vector's size will be decreased, and the existing elements will be destroyed or removed.
- The C++ vector resize function has no return type or value. It is just used to manipulate the size of a vector.
- A bad_alloc exception is thrown if reallocation fails.
- The Time Complexity comes out to be O(n). That is, it takes Linear time to resize the vector.