CRUD Operations in Java
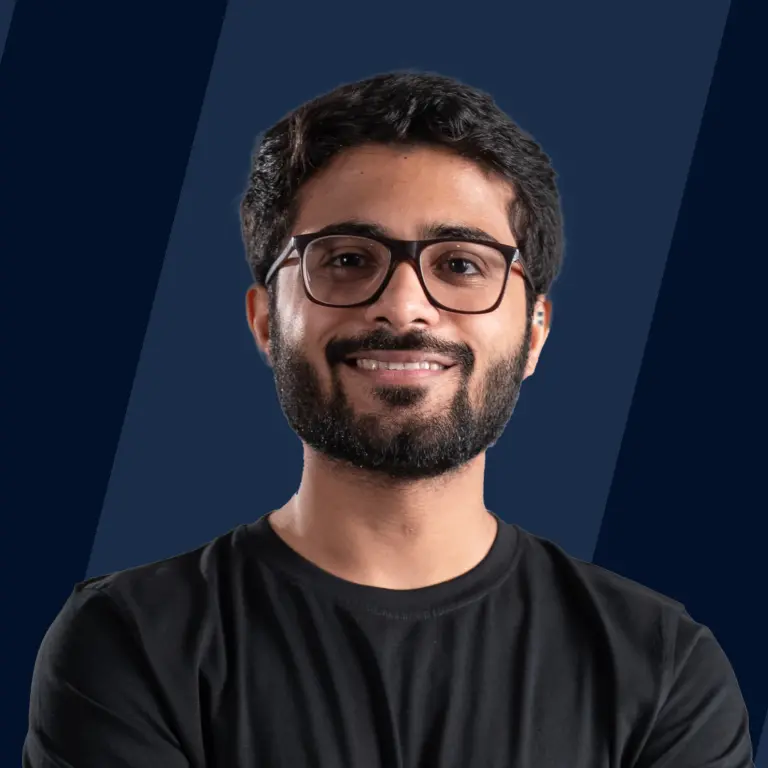
Overview
CRUD Operations in Java, which encompass Create, Read, Update, and Delete, serve as the foundational building blocks for managing data within software applications. These operations are pivotal when interacting with databases for various purposes. To Create data, developers instantiate objects representing the desired entities and subsequently persist them in a database. To Read operation pertains to the retrieval of existing data from a database. To Update data, Java developers employ mechanisms that allow for the modification of existing records within a database. the Delete operation involves the removal of data records from a database.
CRUD Operations in Java
Create Operation
The CRUD Operations in Java involves adding new data records or entities to a database. In Java, this is accomplished by creating instances of classes that represent the entities and then persisting them into the database. This can be achieved using SQL INSERT statements or with the help of Object-Relational Mapping (ORM) frameworks such as Hibernate or JPA. The process usually includes setting the attributes of the object, specifying the data to be stored, and then triggering the insertion process.
Example:
Let us look at an example, where we will be creating data.
Read Operation
The Read operation entails retrieving data from a database. In Java, this is done by querying the database to fetch specific records or information. SQL SELECT statements are commonly used for this purpose, allowing developers to retrieve data based on certain conditions or criteria. ORM frameworks simplify the reading process by providing methods to fetch objects from the database without writing raw SQL queries. Iltering and pagination are common requirements in read operations when dealing with large datasets.
Example:
Let us look at an example, where we will be reading data.
Update Operation
The Update operation involves modifying existing data in the database. In Java, developers can update data by locating the relevant record using SQL UPDATE statements or ORM methods. Once the record is identified, modifications are made to the object representing the data, and these changes are then persisted back to the database. This process ensures that the data in the database reflects the updated information. In some cases, updating records may require locking mechanisms to handle concurrency properly.
Example:
Let us look at an example, where we will be updating data.
Delete Operation
The Delete operation revolves around removing data from a database. In Java, data deletion can be initiated using SQL DELETE statements or ORM functions. Developers identify the data to be deleted, and upon execution, the data record is removed from the database. It's crucial to exercise caution while performing deletion operations, as data loss can have unintended consequences.
Soft Deletes:
Soft deletes refer to a data management technique where records are marked as "deleted" within a database system instead of being physically removed. This approach maintains data integrity by enabling easy recovery, preserving data relationships, and offering an audit trail of deletions, while still allowing the database to reflect changes in data status. In situations where data retention is crucial, soft deletes offer a way to manage data while preserving its integrity and history.
Data Archival Strategies:
Data archival involves the systematic transfer of data from an operational database to a separate storage for long-term retention. This strategy optimizes performance by keeping active databases lean, ensures compliance with regulatory requirements, and enables historical analysis and reporting without cluttering the primary data storage. For scenarios where data retention is vital, implementing data archival strategies helps organizations manage large volumes of historical data effectively.
Example:
Let us look at an example, where we will be deleting data.
CRUD Operations for Student Management System in Java
To explain CRUD Operations in Java actions in the Student Management program, let's imagine making a MENU DRIVER program. This program will include a list of students. Inside this list, there will be student information. The menu will provide four main options: Add, Search, Remove, and Display student details.
Implementation
To implement CRUD Operations in Java for a Student Management System in Java, you'll need to create classes and methods to handle each operation. Below are the main components and steps involved in this implementation:
- Add Student
- Delete Student
- Update Student
- Display Student
- Exit Program
Tasks to Be Operated
The tasks that can be operated within this Student Management System include:
- Create:
Add a new student record to the system. - Read:
Retrieve student records by student ID, name, Contact Number of Student, etc. - Update:
Modify student information such as name, age, or Contact Number of Student. - Delete:
Remove a student record from the system.
The following items should be contained in the student record:
- Student ID
- Name of Student
- Contact Number of Student
Files to Be Created
We will be creating the below files as listed as follows:
- StudentRecordLinkedList:
- This class will manage the linked list of student records.
- It should have methods for adding, retrieving, updating, and deleting records.
- The linked list could be implemented using custom nodes or using Java's built-in LinkedList class.
- StudentRecordManagement:
- This class will provide a user interface for interacting with student records.
- It should have methods to perform CRUD operations using the StudentRecordLinkedList class.
- Input/output handling and user interaction can be managed within this class.
- Record:
- This could be a separate class representing a single student record with attributes like student ID, name, Contact Number of Student, etc.
- This class can be used to create instances of student records.
Let's create the file one by one:
StudentRecordLinkedList
Explanation:
- StudentRecordLinkedList class manages a linked list of student records.
- Inner Node class represents a node in the linked list.
- head is the starting point of the linked list.
Methods:
- addRecord(Record record):
Adds a new record to the end of the linked list. - findRecord(int studentId):
Searches for a record by Student ID and returns it if found. - updateRecord(int studentId, String newName, String newContactNumber):
Updates a record's name and contact number by Student ID. - deleteRecord(int studentId):
Deletes a record from the linked list by Student ID.
StudentRecordManagement
Explanation:
- StudentRecordManagement class demonstrates the usage of StudentRecordLinkedList for CRUD operations.
Main Method:
- Creates an instance of StudentRecordLinkedList.
- Adds two records to the linked list: one for Alice and another for Bob.
- Searches for a record with Student ID 1 (Alice) and displays it if found.
- Updates the record with Student ID 2 (Bob) with a new name and contact number.
- Deletes the record with Student ID 1 (Alice).
- Displays the remaining records (in this case, just the updated Bob's record).
Record
Explanation:
- Record class represents a student record with attributes: studentID, name, and ContactNumber.
- Constructor Record(int studentId, String name, String contactNumber) initializes the record's attributes.
- Getter methods getStudentId(), getName(), and getContactNumber() provide access to the attributes.
Final Outputs
Input Validation and Handling Edge Cases:
In any software application like Student Management System, especially one that deals with user inputs, it's important to implement input validation and handle potential edge cases to ensure the system's stability and user experience. Here are some considerations to keep in mind while implementing theCRUD Operations in Java for the Student Management System:
-
Input Validation:
When users interact with the system, it's crucial to validate the inputs they provide to prevent errors and unexpected behaviour.- Add Operation:
Before adding a new student record, ensure that the input data is valid. Validate fields like student ID, ensuring it's unique and within a valid range. Check for valid names, avoiding special characters or numbers. Validate other fields like contact information or scores as per their expected formats. - Update Operation:
When updating a student's information, validate the input data similarly to the add operation. Ensure that the student ID being updated exists in the system.
- Add Operation:
-
Handling Edge Cases:
Anticipating and handling edge cases can prevent crashes and provide a smoother user experience.- Invalid Input:
If a user provides invalid input (e.g., non-numeric characters for student ID), provide clear error messages explaining the issue and prompt them to re-enter valid data. - Non-Existent Records:
When searching for or attempting to update/delete a student record, check if the record exists before proceeding. If it doesn't exist, inform the user that the record couldn't be found. - Empty Student List:
If the student list is empty and a user tries to perform operations like searching, updating, or deleting, provide a message indicating that there are no records to operate on. - Error Handling:
Implement exception handling to gracefully handle runtime errors. Use try-catch blocks to catch exceptions that might occur during input parsing or database interactions.
- Invalid Input:
-
User-Friendly Messages:
Whenever an error occurs or an edge case is encountered, provide clear and user-friendly messages. These messages should guide the user on what went wrong and how they can rectify it.
By incorporating input validation and addressing potential edge cases, you enhance the usability and reliability of the Student Management System. It reduces the likelihood of crashes or incorrect data manipulations, contributing to a more positive user experience.
FAQs
Q: How do CRUD Operations in Java apply to different types of data sources?
A: CRUD Operations in Java aren't confined to databases alone; they extend their utility to various data sources. In relational databases, they align with SQL statements: INSERT (Create), SELECT (Read), UPDATE (Update), and DELETE (Delete).
Q: How do CRUD Operations in Java relate to RESTful APIs?
A: RESTful APIs closely parallel CRUD Operations in Java and find extensive use in constructing web services. This relationship is observed in several key aspects.
Q: What challenges might developers face when implementing CRUD Operations in Java?
A: Developers might encounter various challenges when implementing CRUD Operations in Java. Ensuring data integrity requires careful handling of updates and deletes to maintain consistency.
Q: How can developers enhance security when implementing CRUD Operations in Java?
A: Enhancing security during CRUD Operations in Java implementation involves adopting several best practices. Employing role-based access control ensures that only authorized users can perform specific CRUD actions. Parameterized queries and input validation prevent SQL injection attacks.
Conclusion
- CRUD Operations in Java, constituting Create, Read, Update, and Delete, serve as the cornerstones of modern software development, offering a structured approach to data manipulation.
- These operations hold paramount significance as they establish a universal language for developers to interact with data, fostering consistency and simplification across various applications.
- Their significance becomes particularly evident in the context of maintaining data integrity, ensuring that data remains accurate, reliable, and free from anomalies throughout its lifecycle.
- CRUD operations are not confined to databases alone; they possess remarkable adaptability, extending their utility to diverse data sources such as files, APIs, and in-memory data structures.
- In relational databases, these operations seamlessly correspond to SQL statements, allowing developers to seamlessly insert, retrieve, update, and delete records within tables.
- Files, as data sources, effectively embrace CRUD operations by facilitating data writing, reading, updating, and deletion, thereby enabling efficient data management.
- The convergence of CRUD operations with RESTful APIs underscores their versatility, offering a standardized means of communication between distributed systems and enabling seamless resource interaction.
- The implementation of CRUD operations isn't without its challenges. Developers must tackle concerns related to data consistency, concurrency control, performance optimization, and error handling.